Question
C++ DATA STRUCTURES UNSORTED LISTS. I'm just trying to run the project, it seems that all my function declaration are fine (I called it sorted
C++ DATA STRUCTURES UNSORTED LISTS.
I'm just trying to run the project, it seems that all my function declaration are fine (I called it sorted but I know it's unsorted lol), but Im not sure how to declare the function in my main program. Here are the instructions of my project (please use my functions already declared).
An Unsorted Type ADT is to be extended by the addition of function SplitLists, which as the following specifications: SplitLists(UnsortedType list, ItemType item, UnsortedType& list1. UnsortedType& list2) Function: Divideslist into two lists according to the key of item. Preconditions:list has been initialized and is not empty. Post conditions: list1 contains all the items of list whose keys are less than or equal to items key; list2 contains all of the items oflist whose keys are greater than items key. a. Implement SplitLists as an array-based member function of the Unsorted List ADT. b. Implement SplitLists as a linked member function of the Unsorted List ADT.
-----------------------------------------------------------------------------------------------------------------------------------------------
#pragma once
#include
#include
using namespace std;
const int MAX_ITEMS = 5;
enum RelationType {LESS, GREATER, EQUAL};
//create class type
class ItemType
{
public:
ItemType();
RelationType ComparedTo(ItemType) const;
void Print(ofstream&) const;
void Initialize(int number);
private:
int value;
};
//////////////////////////////////////////////////////
#include
#include
#include "main.h"
using namespace std;
ItemType::ItemType()
{
value = 0;
}
RelationType ItemType::ComparedTo(ItemType otherItem) const
{
if (value < otherItem.value)
return LESS;
else if (value > otherItem.value)
return GREATER;
else return EQUAL;
}
void ItemType::Initialize(int number)
{
value = number;
}
void ItemType::Print(ofstream& out) const
{
out << value << "";
}
//////////////////////////////////////////////////////
#pragma once
#include
#include"main.h" //included
using namespace std;
ItemType info[MAX_ITEMS];
class SortedType {
public:
//constructor
SortedType();
void MakeEmpty();
bool IsFull () const;
ItemType GetItem(ItemType item, bool& found);
int GetLength() const;
//void SplitLists();
void PutItem(ItemType item);
//void DeleteItem(int item);
void ResetList();
ItemType GetNextItem();
private:
int length;
int currentPos;
ItemType info[MAX_ITEMS];
int currentPOS;
};
////////////////////////////////////////////////////////////////////////////////
#include
#include "SortedList.h"
using namespace std;
SortedType::SortedType() //length starts at = 0
{
length = 0;
} //complete
bool SortedType::IsFull() const {
return (length == MAX_ITEMS);
}//COMP
int SortedType::GetLength() const { //return length
return length;
} //complete
void SortedType::MakeEmpty()
{
length = 0;
} //complete
void SortedType::PutItem(ItemType item)
{
info[length] = item;
length++;
} //complete
void SortedType::ResetList()
{
currentPos = -1;
} //complete
ItemType SortedType::GetNextItem()
{
currentPos ++;
return info[currentPos];
} //comple
ItemType SortedType::GetItem(ItemType item, bool& found)
{
bool moreToSearch;
int location = 0;
found = false;
moreToSearch = (location
while (moreToSearch && !found)
{
switch (item.ComparedTo(info[location]))
{
case LESS:
case GREATER: location++;
moreToSearch = (location
moreToSearch = (location != NULL);
break;
case EQUAL: found = true;
item = info[location];
break;
}
}
return item;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
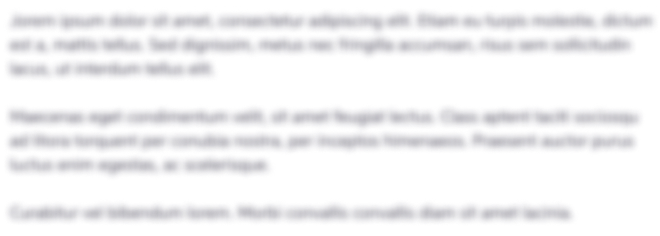
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started