Question
C++. Difficulty getting function for call correctly in driver. I'm having trouble getting the program that gets the position of a given entry in this
C++. Difficulty getting function for call correctly in driver.
I'm having trouble getting the program that gets the position of a given entry in this list. This method will have one parameter of type ItemType that specifies the entry you are searching for. If the entry is found, its position will be returned (an integer in the range 1 through itemCount). If the list contains multiple entries with that same value, return the position of the entry with the smallest position number. If the entry is not found in the list, the method returns zero.
Here is the code I have:
ArrayList.cpp
#include
#include
#include "ArrayList.h" // ADT list operations
using namespace std;
void displayList(ListInterface
{
if (listPtr->isEmpty())
cout << "The list is empty" << endl;
else
{
cout << "The list contains " << endl;
for (int pos = 1; pos <= listPtr->getLength(); pos++)
{
cout << listPtr->getEntry(pos) << " ";
}
cout << endl;
}
}
int main()
{
ListInterface
cout << "List should be empty" << endl;;
displayList(listPtr);
cout << "Length : " << listPtr->getLength() << "; should be 0" << endl << endl;
listPtr->insert(1, "two");
displayList(listPtr);
cout << "Length : " << listPtr->getLength() << "; should be 1" << endl << endl;
listPtr->insert(1, "one");
displayList(listPtr);
cout << "Length : " << listPtr->getLength() << "; should be 2" << endl << endl;
listPtr->insert(3, "three");
displayList(listPtr);
cout << "Length : " << listPtr->getLength() << "; should be 3" << endl << endl;
listPtr->insert(3, "two.five");
displayList(listPtr);
cout << "Length : " << listPtr->getLength() << "; should be 4" << endl << endl;
listPtr->insert(5, "five");
displayList(listPtr);
cout << "Length : " << listPtr->getLength() << "; should be 5" << endl << endl;
//getPosition
//Call getPosition to display item in list
listPtr->getPosition("one");
displayList(listPtr);
cout << "The position of one in the list is: " << listPtr->getPosition("one") << endl;
cout << "List should be full; next insert should fail" << endl;
if (listPtr->insert(6, "six"))
cout << "Inserted \"six\" at position 6" << endl;
else
cout << "Cannot insert \"six\" at position 6" << endl;
displayList(listPtr);
cout << "Length : " << listPtr->getLength() << "; should be 5" << endl << endl;
cout << "Remove first entry" << endl;
listPtr->remove(1);
displayList(listPtr);
cout << "Length : " << listPtr->getLength() << "; should be 4" << endl << endl;
cout << "Remove last entry" << endl;
listPtr->remove(4);
displayList(listPtr);
cout << "Length : " << listPtr->getLength() << "; should be 3" << endl << endl;
cout << "Remove remaining entries" << endl;
listPtr->remove(1);
listPtr->remove(1);
listPtr->remove(1);
displayList(listPtr);
cout << "Length : " << listPtr->getLength() << "; should be 0" << endl << endl;
cout << "Try to remove entry from empty list" << endl;
if (listPtr->remove(1))
cout << "Removed an entry" << endl;
else
cout << "Cannot remove entry" << endl;
// test clear
listPtr->insert(1, "one");
displayList(listPtr);
cout << "Length : " << listPtr->getLength() << "; should be 1" << endl << endl;
listPtr->insert(2, "two");
displayList(listPtr);
cout << "Length : " << listPtr->getLength() << "; should be 2" << endl << endl;
cout << "Clearing list" << endl;
listPtr->clear();
displayList(listPtr);
cout << "Length : " << listPtr->getLength() << "; should be 0" << endl << endl;
return 0;
} // end main
ListInterface.h
/** Interface for the ADT list
Listing 8-1
@file ListInterface.h */
#ifndef _LIST_INTERFACE
#define _LIST_INTERFACE
template
class ListInterface
{
public:
virtual bool isEmpty() const = 0;
virtual int getLength() const = 0;
virtual bool insert(int newPosition, const ItemType& newEntry) = 0;
virtual bool remove(int position) = 0;
virtual void clear() = 0;
virtual ItemType getEntry(int position) const = 0;
virtual void replace(int position, const ItemType& newEntry) = 0;
virtual int getPosition(const ItemType& anEntry) const = 0;
}; // end ListInterface
#endif
ArrayList.h
/** ADT list: Array-based implementation.
Listing 9-1.
@file ArrayList.h */
#ifndef ARRAY_LIST_
#define ARRAY_LIST_
#include "ListInterface.h"
#include
template
class ArrayList : public ListInterface
{
private:
static const int DEFAULT_CAPACITY = 5; // Small capacity to test for a full list
ItemType items[DEFAULT_CAPACITY+1]; // Array of list items (not using element [0]
int itemCount; // Current count of list items
int maxItems; // Maximum capacity of the list
public:
ArrayList();
// Copy constructor and destructor are supplied by compiler
bool isEmpty() const;
int getLength() const;
bool insert(int newPosition, const ItemType& newEntry);
bool remove(int position);
void clear();
/** @throw PrecondViolatedExcep if position < 1 or
position > getLength(). */
ItemType getEntry(int position) const;
/** @throw PrecondViolatedExcep if position < 1 or
position > getLength(). */
void replace(int position, const ItemType& newEntry);
//getPosition
int getPosition(const ItemType& anEntry) const;
}; // end ArrayList
/** Implementation file for the class ArrayList.
@file ArrayList.cpp */
template
ArrayList
{
} // end default constructor
template
bool ArrayList
{
return itemCount == 0;
} // end isEmpty
template
int ArrayList
{
return itemCount;
} // end getLength
template
bool ArrayList
{
bool ableToInsert = (newPosition >= 1)
&& (newPosition <= itemCount + 1)
&& (itemCount < maxItems);
if (ableToInsert)
{
// Make room for new entry by shifting all entries at
// positions >= newPosition toward the end of the array
// (no shift if newPosition == itemCount + 1)
for (int entryPosition = itemCount; entryPosition >= newPosition; entryPosition--)
items[entryPosition + 1] = items[entryPosition]; // copy the entry right
// Insert new entry
items[newPosition] = newEntry;
itemCount++; // Increase count of entries
} // end if
return ableToInsert;
} // end insert
template
bool ArrayList
{
bool ableToRemove = (position >= 1) && (position <= itemCount);
if (ableToRemove)
{
// Remove entry by shifting all entries after the one at
// position toward the beginning of the array
// (no shift if position == itemCount)
for (int entryPosition = position; entryPosition < itemCount; entryPosition++)
items[entryPosition] = items[entryPosition + 1]; // copy entry on the right to left
itemCount--; // Decrease count of entries
} // end if
return ableToRemove;
} // end remove
template
void ArrayList
{
itemCount = 0;
} // end clear
template
ItemType ArrayList
{
// Enforce precondition
bool ableToGet = (position >= 1) && (position <= itemCount);
assert( ableToGet );
return items[position];
} // end getEntry
template
void ArrayList
{
// Enforce precondition
bool ableToSet = (position >= 1) && (position <= itemCount);
assert (ableToSet);
items[position] = newEntry;
} // end replace
// End of implementation file.
template
int ArrayList
{
//Variable for position
int position = 0;
//Make sure list isn't empty
if(!isEmpty())
{
//Variable for index
int index = 0;
//Locate index
while((position == 0) && (index < itemCount))
{
//Index is at getEntry
if(anEntry == items[index])
{
position = index + 1;
}
}
}
//return position
return position;
}
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
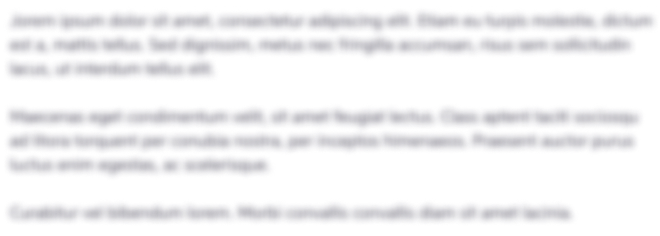
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started