C++.
Don't copy from existing answers. They were not helpful. I will upvote if the answer is good.
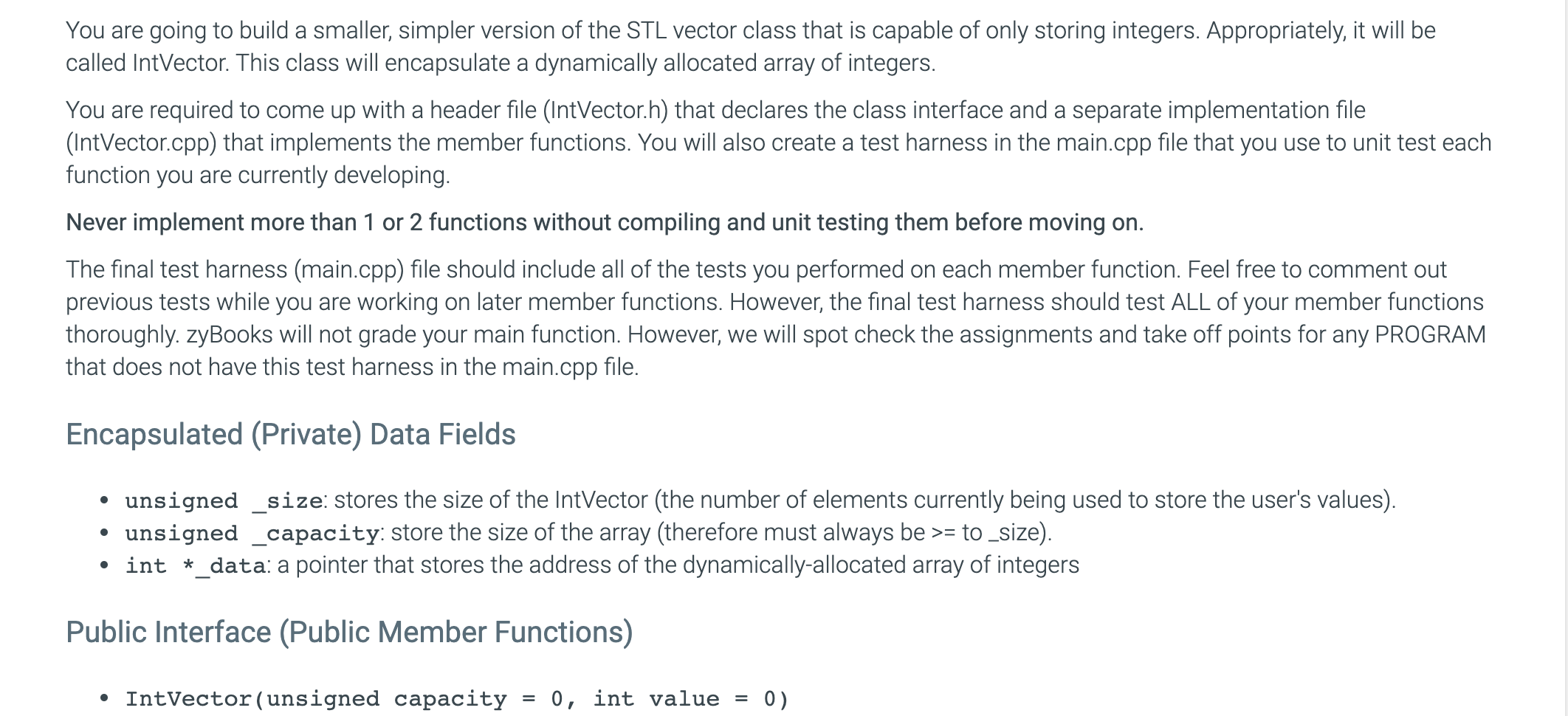
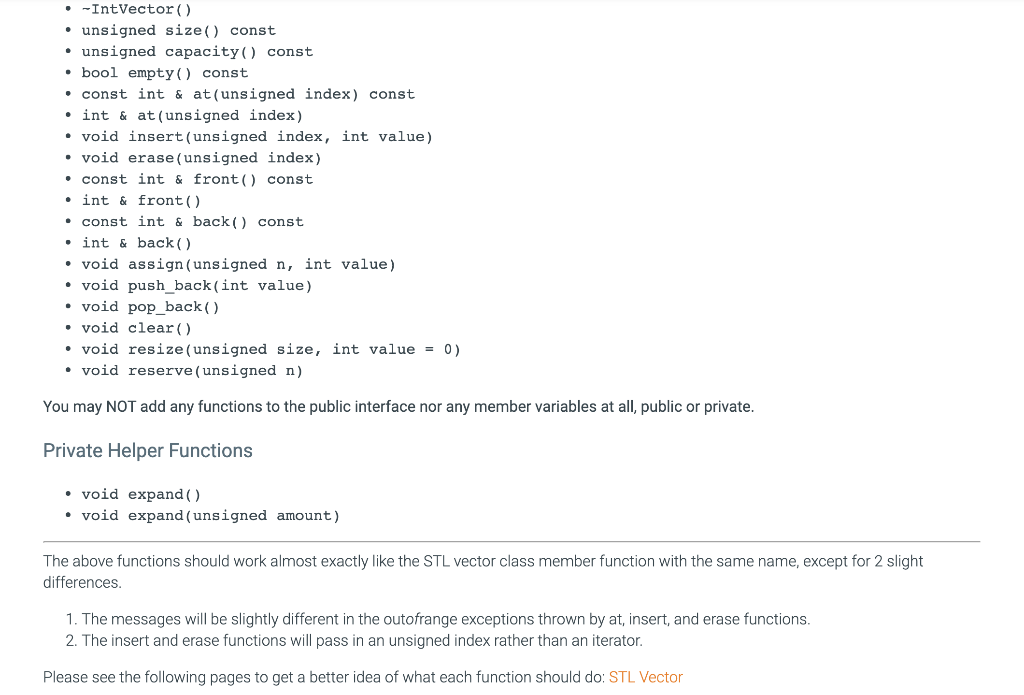
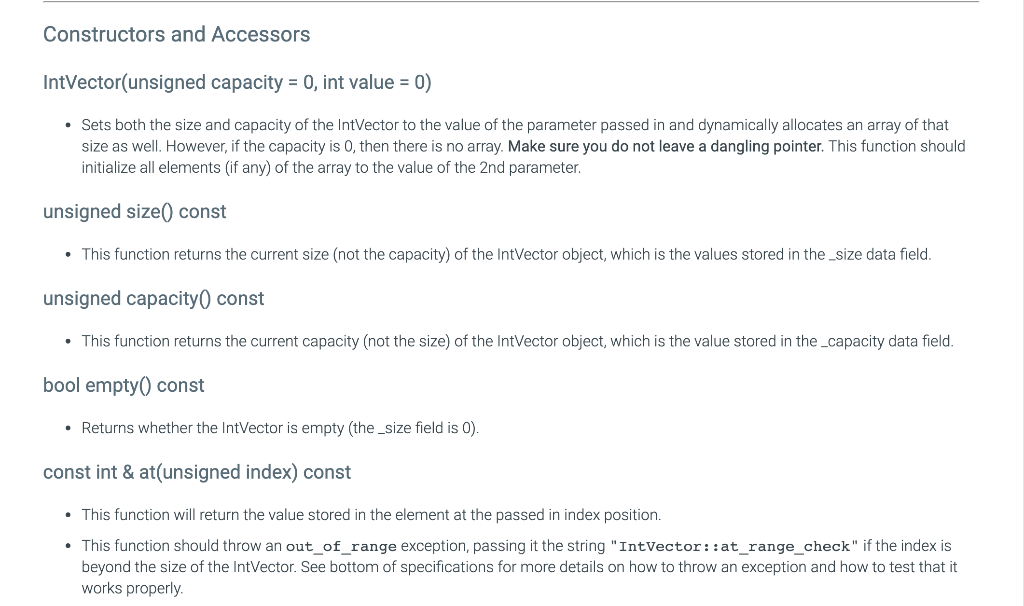
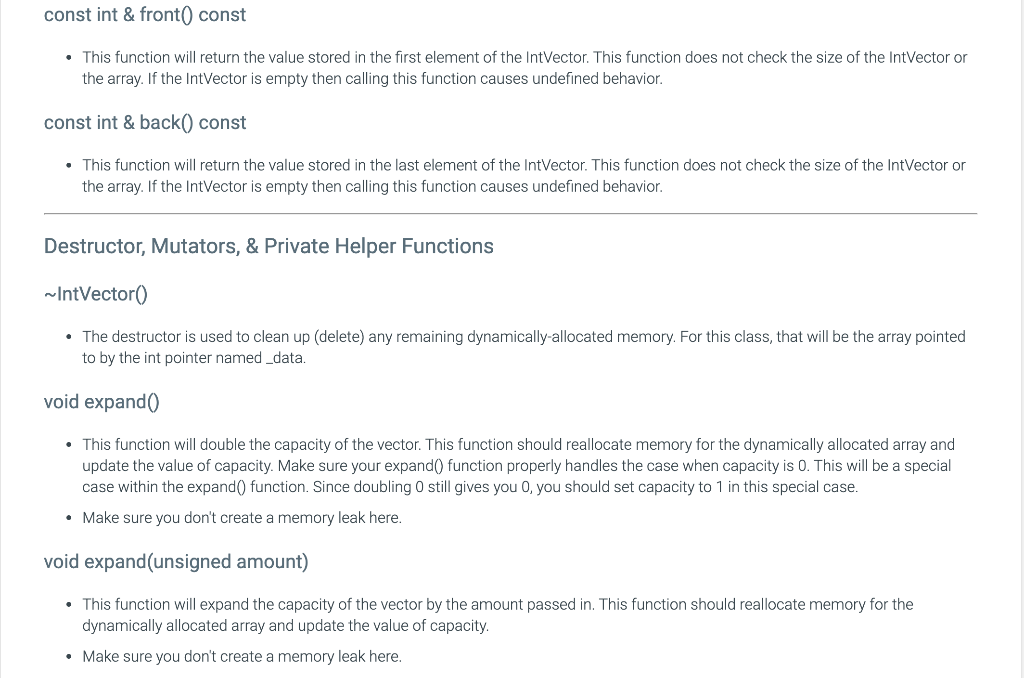
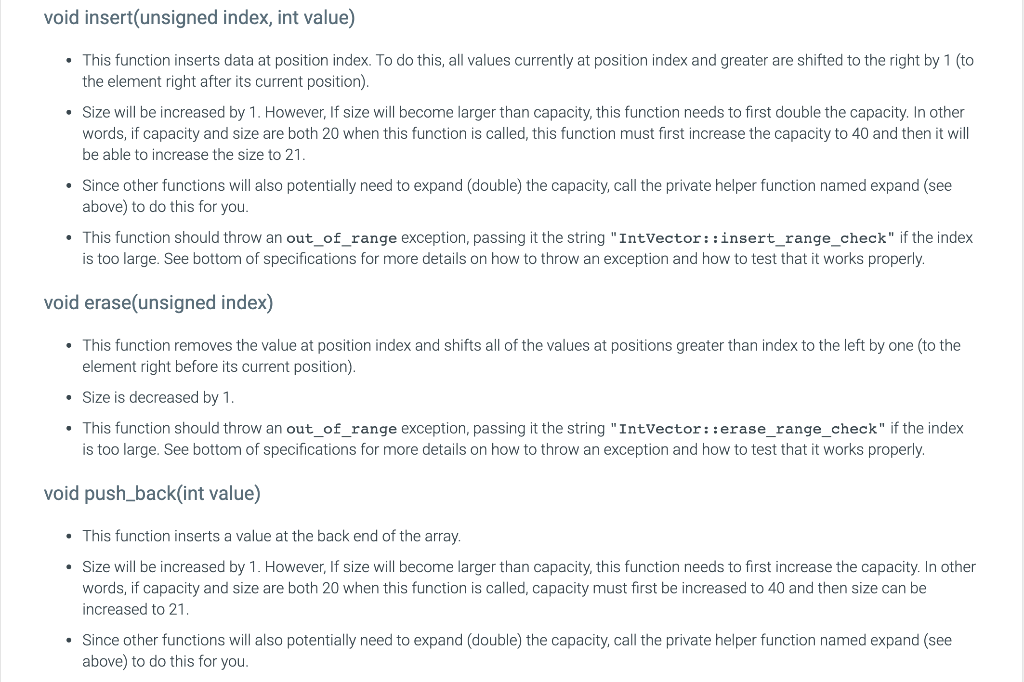
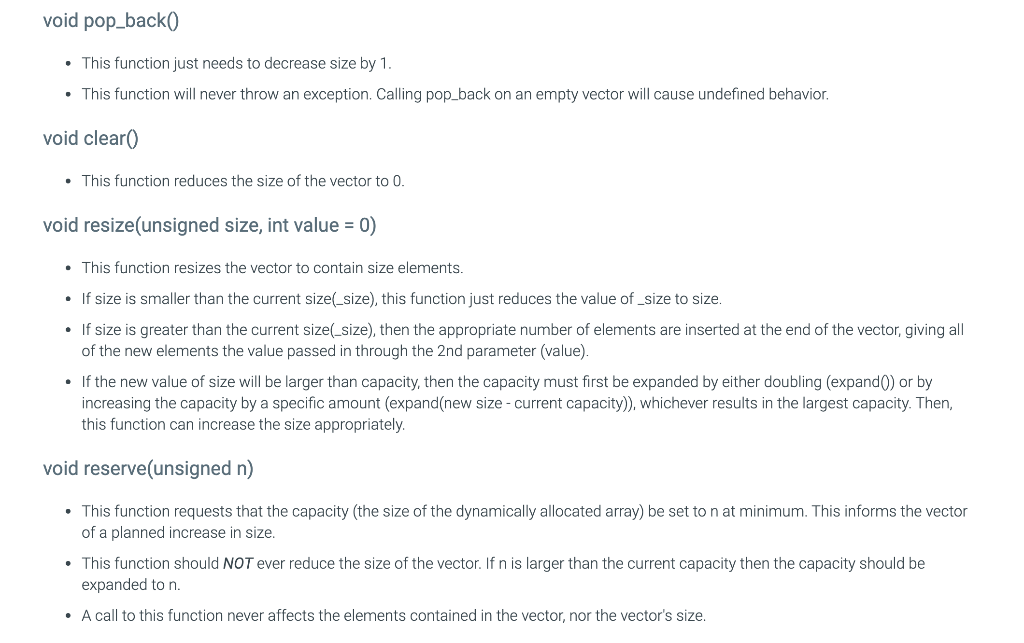
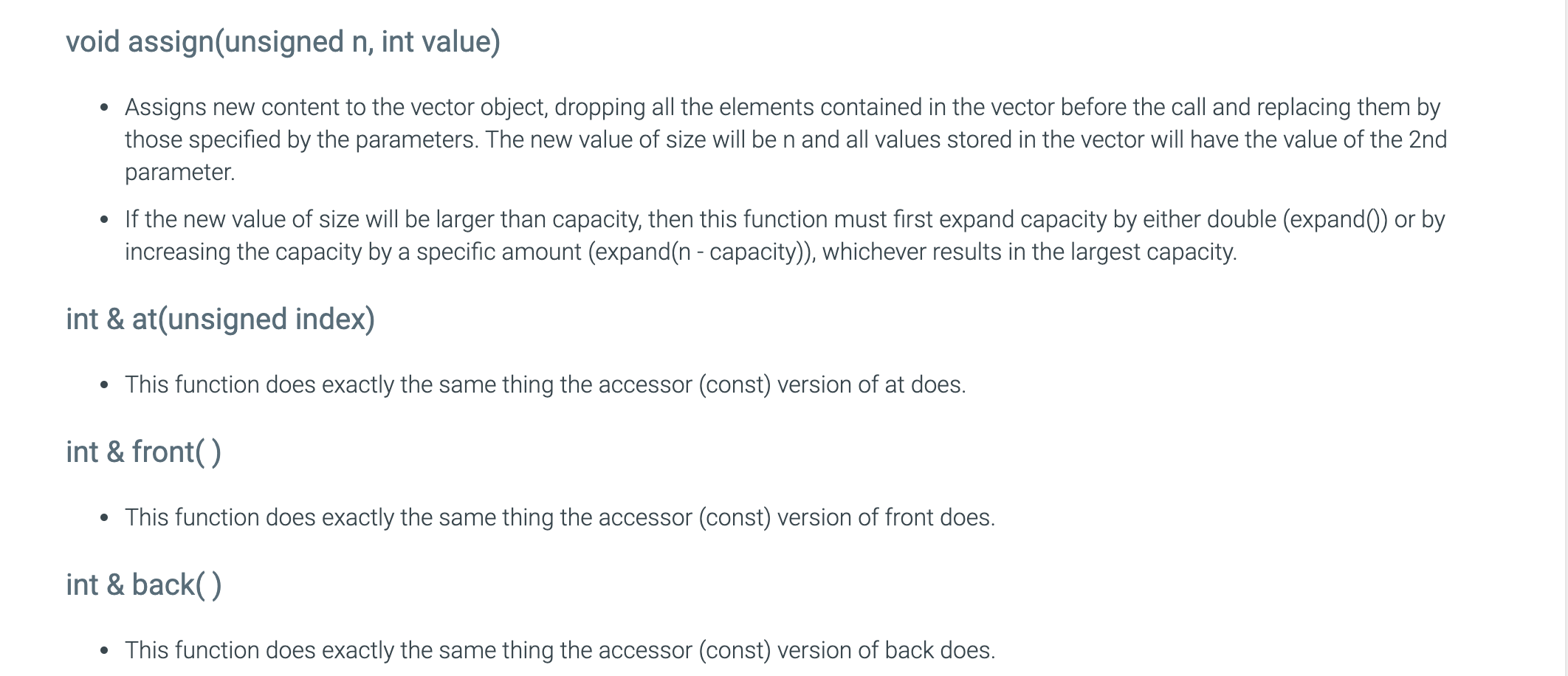
You are going to build a smaller, simpler version of the STL vector class that is capable of only storing integers. Appropriately, it will be called IntVector. This class will encapsulate a dynamically allocated array of integers. You are required to come up with a header file (IntVector.h) that declares the class interface and a separate implementation file (IntVector.cpp) that implements the member functions. You will also create a test harness in the main.cpp file that you use to unit test each function you are currently developing. Never implement more than 1 or 2 functions without compiling and unit testing them before moving on. The final test harness (main.cpp) file should include all of the tests you performed on each member function. Feel free to comment out previous tests while you are working on later member functions. However, the final test harness should test ALL of your member functions thoroughly. zyBooks will not grade your main function. However, we will spot check the assignments and take off points for any PROGRAM that does not have this test harness in the main.cpp file. Encapsulated (Private) Data Fields unsigned _size: stores the size of the IntVector (the number of elements currently being used to store the user's values). unsigned _capacity: store the size of the array (therefore must always be >= to _size). int *_data: a pointer that stores the address of the dynamically-allocated array of integers Public Interface (Public Member Functions) IntVector(unsigned capacity = 0, int value = 0) . -IntVector() unsigned size() const unsigned capacity() const .bool empty() const const int & at(unsigned index) const int & at(unsigned index) void insert(unsigned index, int value) void erase (unsigned index) const int & front() const int & front() const int & back() const int & back() void assign(unsigned n, int value) void push_back(int value) void pop_back() void clear() void resize(unsigned size, int value = 0) void reserve (unsigned n) You may NOT add any functions to the public interface nor any member variables at all, public or private. Private Helper Functions void expand() void expand (unsigned amount) The above functions should work almost exactly like the STL vector class member function with the same name, except for 2 slight differences. 1. The messages will be slightly different in the outofrange exceptions thrown by at, insert, and erase functions. 2. The insert and erase functions will pass in an unsigned index rather than an iterator. Please see the following pages to get a better idea of what each function should do: STL Vector Constructors and Accessors IntVector(unsigned capacity = 0, int value = 0) Sets both the size and capacity of the IntVector to the value of the parameter passed in and dynamically allocates an array of that size as well. However, if the capacity is 0, then there is no array. Make sure you do not leave a dangling pointer. This function should initialize all elements (if any) of the array to the value of the 2nd parameter. unsigned size() const This function returns the current size (not the capacity) of the IntVector object, which is the values stored in the size data field. unsigned capacity const This function returns the current capacity (not the size) of the IntVector object, which is the value stored in the _capacity data field. bool empty() const Returns whether the IntVector is empty (the _size field is 0). const int & at(unsigned index) const This function will return the value stored in the element at the passed in index position. This function should throw an out_of_range exception, passing it the string "IntVector::at_range_check" if the index is beyond the size of the IntVector. See bottom of specifications for more details on how to throw an exception and how to test that it works properly. const int & front const This function will return the value stored in the first element of the IntVector. This function does not check the size of the IntVector or the array. If the IntVector is empty then calling this function causes undefined behavior. const int & back() const This function will return the value stored in the last element of the IntVector. This function does not check the size of the IntVector or the array. If the IntVector is empty then calling this function causes undefined behavior. Destructor, Mutators, & Private Helper Functions IntVector The destructor is used to clean up (delete) any remaining dynamically-allocated memory. For this class, that will be the array pointed to by the int pointer named _data. void expand This function will double the capacity of the vector. This function should reallocate memory for the dynamically allocated array and update the value of capacity. Make sure your expand() function properly handles the case when capacity is 0. This will be a special case within the expand function. Since doubling 0 still gives you 0, you should set capacity to 1 in this special case. Make sure you don't create a memory leak here. void expand(unsigned amount) This function will expand the capacity of the vector by the amount passed in. This function should reallocate memory for the dynamically allocated array and update the value of capacity. Make sure you don't create a memory leak here. void insert(unsigned index, int value) This function inserts data at position index. To do this, all values currently at position index and greater are shifted to the right by 1 (to the element right after its current position) Size will be increased by 1. However, If size will become larger than capacity, this function needs to first double the capacity. In other words, if capacity and size are both 20 when this function is called, this function must first increase the capacity to 40 and then it will be able to increase the size to 21. Since other functions will also potentially need to expand (double) the capacity, call the private helper function named expand (see above) to do this for you. This function should throw an out_of_range exception, passing it the string "IntVector::insert_range_check" if the index is too large. See bottom of specifications for more details on how to throw an exception and how to test that it works properly. void erase(unsigned index) This function removes the value at position index and shifts all of the values at positions greater than index to the left by one (to the element right before its current position). Size is decreased by 1. This function should throw an out_of_range exception, passing it the string "IntVector::erase_range_check" if the index is too large. See bottom of specifications for more details on how to throw an exception and how to test that it works properly. void push_back(int value) This function inserts a value at the back end of the array Size will be increased by 1. However, If size will become larger than capacity, this function needs to first increase the capacity. In other words, if capacity and size are both 20 when this function is called, capacity must first be increased to 40 and then size can be increased to 21. Since other functions will also potentially need to expand (double) the capacity, call the private helper function named expand (see above) to do this for you. void pop_back() This function just needs to decrease size by 1. This function will never throw an exception. Calling pop_back on an empty vector will cause undefined behavior. void clear() This function reduces the size of the vector to 0. void resize(unsigned size, int value = 0) This function resizes the vector to contain size elements. If size is smaller than the current size(_size), this function just reduces the value of size to size. If size is greater than the current size(_size), then the appropriate number of elements are inserted at the end of the vector, giving all of the new elements the value passed in through the 2nd parameter (value) If the new value of size will be larger than capacity, then the capacity must first be expanded by either doubling (expand) or by increasing the capacity by a specific amount (expand(new size - current capacity)), whichever results in the largest capacity. Then, this function can increase the size appropriately. void reserve(unsigned n) This function requests that the capacity (the size of the dynamically allocated array) be set to n at minimum. This informs the vector of a planned increase in size. This function should NOT ever reduce the size of the vector. If n is larger than the current capacity then the capacity should be expanded to n. A call to this function never affects the elements contained in the vector, nor the vector's size. void assign(unsigned n, int value) Assigns new content to the vector object, dropping all the elements contained in the vector before the call and replacing them by those specified by the parameters. The new value of size will be n and all values stored in the vector will have the value of the 2nd parameter. If the new value of size will be larger than capacity, then this function must first expand capacity by either double (expand()) or by increasing the capacity by a specific amount (expand(n - capacity)), whichever results in the largest capacity. int & at(unsigned index) This function does exactly the same thing the accessor (const) version of at does. int & front() This function does exactly the same thing the accessor (const) version of front does. int & back() This function does exactly the same thing the accessor (const) version of back does