Question
C++ Error running code... Using: Visual Studio Express 2015 for Windows Desktop It seems to be at this line of the code --> cashier1 =
C++ Error running code...
Using: Visual Studio Express 2015 for Windows Desktop
It seems to be at this line of the code --> cashier1 = *curr; (line 297 for me)
I have the file in the right location and even hardcode a value from the text file in the ELSE statement when it can't open the file. Still get the error messages.
Errors I'm getting:
When I click on Ignore on the error above, I get the next error message below.
Code
// FP_test1.cpp : Defines the entry point for the console application.
//
#include "stdafx.h"
#include
#include
#include
#include
#include
#include
using namespace std;
//customer class to hold information pulled from notepad
class Customer
{
//all information on the customer pulled from notepad
int number;
int arrival_time;
int serv_time;
public:
Customer()
{
number = 0;
arrival_time = 0;
serv_time = 0;
}
void set_number(int x)
{
number = x;
}
void set_arrival_time(int x)
{
arrival_time = x;
}
int get_arrival_time()
{
return arrival_time;
}
void set_serv_time(int x)
{
serv_time = x;
}
int get_serv_time()
{
return serv_time;
}
};
//Cashier class to act as 5 queues for customer info to be held in
class Cashier
{
int timer; //minutes in use
char status; // 'a' -available, 'i' - inactive
queue time_limit; //depends on customer serv_time
int in_line;
public:
Cashier()
{
timer = 0;
status = 'a';
in_line = 0;
}
char get_status()
{
return status;
}
void inc_timer()
{
timer++;
}
int get_timer()
{
return timer;
}
int get_in_line()
{
return in_line;
}
void add_customer(int serv_time)
{
//sets timer to 0 for first input, since i reset it on pop its
/eeded for first entry.
if (time_limit.empty())
{
timer = 0;
}
//puts the serv_time on the queue of time_limits
time_limit.push(serv_time);
//add customer to line
in_line++;
if (in_line == 6)
{
status = 'i';
}
}
int get_curr_cust_time_limit()
{
return time_limit.front();
}
void rmv_frnt_cust()
{
time_limit.pop();
timer = 0;
in_line--;
status = 'a';
}
};
//Sim_data_type class to hold overall simulation data
class Sim_data_type
{
int time_cnt; // ranges in values from 0 to 600
int num_serviced; // number of customers serviced so far
long tot_wait_time; // of all customers in the queues
int num_turned_away; // count of customers turned away
public:
Sim_data_type()
{
time_cnt = 0;
num_serviced = 0;
tot_wait_time = 0;
num_turned_away = 0;
}
void inc_cnt()
{
time_cnt++;
}
int get_cnt()
{
return time_cnt;
}
void inc_num_turned_away()
{
num_turned_away++;
}
int get_num_turned_away()
{
return num_turned_away;
}
void inc_num_serviced()
{
num_serviced++;
}
int get_num_serviced()
{
return num_serviced;
}
int get_avg_wait_time()
{
return tot_wait_time / num_serviced;
}
void add_tot_wait_time(int x)
{
tot_wait_time = tot_wait_time + x;
}
};
#include
#include
#include
#include
#include
using namespace std;
int main(void)
{
//setting up the 5 lines of cashiers
Cashier cashier1;
Cashier cashier2;
Cashier cashier3;
list lines;
list::iterator curr = lines.begin();
list::iterator next = lines.begin();
for (int i = 0; i
{
lines.push_back(cashier1);
}
//creating Customer variable to temporarily hold input
Customer cust;
//creating queue to hold input from file
queue custInput;
//creating variable to hold simulation data
Sim_data_type sim;
//file for getting input
ifstream in_file;
//used to temperarily hold the line obtained from the file
string line;
//used to temperarily hold the selected substring
string substring;
//opening file
in_file.open("output.txt");
if (in_file.fail())
{
//cout
//return 0;
//getting the first digits for (number)
substring = 1;
cust.set_number(atoi(substring.c_str()));
//getting the next digits for (arrival_time)
substring = 10;
cust.set_arrival_time(atoi(substring.c_str()));
//getting the next digits for (serv_time)
substring = 2;
cust.set_serv_time(atoi(substring.c_str()));
//adding newly created customer to the data queue
custInput.push(cust);
}
else
{
//get data from file until end of file
while (!in_file.eof())
{
//gets input until new line
getline(in_file, line);
//if extracted string isn't empty
if (line.length() > 0)
{
//getting the first digits for (number)
substring = line.substr(0, 2);
cust.set_number(atoi(substring.c_str()));
//getting the next digits for (arrival_time)
substring = line.substr(4, 4);
cust.set_arrival_time(atoi(substring.c_str()));
//getting the next digits for (serv_time)
substring = line.substr(9, 3);
cust.set_serv_time(atoi(substring.c_str()));
//adding newly created customer to the data queue
custInput.push(cust);
}
}
//closing file
in_file.close();
}
//while store not closed
while (sim.get_cnt()
{
//increment minutes
sim.inc_cnt();
for (int i = 0; i
{
cashier1 = *curr;
curr++;
cashier1.inc_timer();
*curr = cashier1;
}
//move cashiers to proper positions
curr = lines.end();
next = lines.end();
next--;
while (curr != lines.begin())
{
curr--;
next--;
cashier1 = *curr;
cashier2 = *next;
if (cashier1.get_in_line()
{
//swap placements so cashier with the smallest line is in
//front
cashier3 = cashier2;
cashier2 = cashier1;
cashier1 = cashier3;
}
}
//while customers can still be served
if (sim.get_cnt()
{
//set cust to temporarily hold front of custInput
cust = custInput.front();
//put customer in smallest line
curr = lines.begin();
cashier1 = *curr;
//if the front of queue is ready to join line
while (cust.get_arrival_time() == sim.get_cnt())
{
//flag to see if more than one customer has arrived at the
//same time
int flag = 0;
//if another round move to next line
if (flag > 0)
{
curr++;
cashier1 = *curr;
}
//set the customer to the first available/shortest
//lane; if none are open turn them away
if (cashier1.get_status() == 'a')
{
//adding customers serv time to cashiers queue
cashier1.add_customer(cust.get_serv_time());
//removing customer from input we added to queue
custInput.pop();
//setting cust to new front so while checks correctly
cust = custInput.front();
}
else
{
sim.inc_num_turned_away();
}
flag++;
}
}
//increment sim variables for output later
//if cashiers timer = the serv time of cust
//then remove that cust
curr = lines.begin();
cashier1 = *curr;
for (int i = 0; i
{
if (cashier1.get_timer() == cashier1.get_curr_cust_time_limit())
{
//add the customer-to-be-removed's wait time to total wait
sim.add_tot_wait_time(cashier1.get_curr_cust_time_limit());
//remove it front queue
cashier1.rmv_frnt_cust();
//increment the total number serviced
sim.inc_num_serviced();
}
curr++;
cashier1 = *curr;
}
}
//final output
cout
cout
cout
//exit program
return 0;
}
output.txt file
1 10 2 2 14 7 3 29 7 4 46 3 5 53 5 6 55 7 7 67 6 8 70 6 9 79 3 10 93 1 11 104 2 12 108 4 13 124 1 14 143 3 15 161 3 16 170 1 17 174 7 18 183 3 19 198 6 20 199 4 21 207 1 22 211 3 23 223 4 24 231 2 25 231 5 26 245 7 27 259 6 28 275 1 29 289 1 30 293 5
Microsoft Visual C++Runtime Library Debug Assertion Failed! Program: C:windowsisystem321MSVCP140D.dl File: c:\program files (x86)\microsoft visual studio 14.0\vcu ncludellist Line: 211 Expression: listit erator not derefrencable For information on how your program can cause an assertion failure, see the Visual C++ documentation on asserts. (Press Retry to debug the application) Abort Retry IgnoreStep by Step Solution
There are 3 Steps involved in it
Step: 1
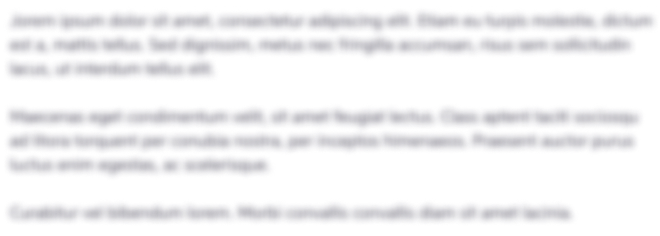
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started