Question
//C++ // Extend the Linked List, edit this code so that it can add and print the following options: //1. Get the average of the
//C++
// Extend the Linked List, edit this code so that it can add and print the following options:
//1. Get the average of the data in the linked list
//2. Get the minimum value in the linked list //3. Get the maximum value in the linked list //4. "FindAll" how many times a certain given value is repeated in the list ( could be 0,1,...ListSize)
#include "stdafx.h"
#include
using namespace std;
struct Node
{
int Element;
Node* Next;
};
/*********************** InsertBeginning ************************************/
void InsertBeginning(int X, Node* L)
{
Node* TmpCell = new Node;
TmpCell->Element = X;
TmpCell->Next = L->Next;
L->Next = TmpCell;
}
/*********************** InsertEnd ************************************/
void InsertEnd(int X, Node* L)
{
Node *P = L;
while (P->Next != NULL)
{
P = P->Next;
}
Node* baby = new Node;
baby->Next = NULL;
L->Next = NULL;
P->Next = baby;
}
/************************* Find ***********************************/
Node* Find(int X, Node* L)
{
Node* P;
P = L->Next;
while (P != NULL && P->Element != X)
P = P->Next;
return P;
}
/********************** PrintList ************************************/
void PrintList(Node* L)
{
Node* P = L; // P points to the empty header
cout << " List: ";
while (P->Next != NULL)
{
P = P->Next; // move one node forward
cout << P->Element << " "; // print out only the element part
} // stop when there is no next node
cout << endl;
}
/************************* DeleteWholeList ************************/
void DeleteWholeList(Node* L)
{
Node* P;
Node* Tmp;
P = L->Next;
L->Next = NULL;
while (P != NULL)
{
Tmp = P->Next;
free(P);
P = Tmp;
}
}
/********************** Delete *****************************************/
void Delete(int X, Node* L)
{
Node* P;
Node* TmpCell;
P = L;
while (P->Next != NULL && P->Next->Element != X)
P = P->Next;
if (P->Next != NULL)
{ /* X is found; delete it */
TmpCell = P->Next;
P->Next = TmpCell->Next; /* Bypass deleted cell */
free(TmpCell);
}
}
/************************** Count ******************************************/
int Count(Node* L)
{
Node* P = L; // P points to the empty header
int c = 0;
while (P->Next != NULL)
{
P = P->Next; // move one node forward
c++; // print out only the element part
} // stop when there is no next node
return c;
}
/********************** Sum *****************************************/
int Sum (Node* L)
{
Node* P = L; // P points to the empty header
int Sum = 0;
while (P->Next != NULL)
{
P = P->Next; // move one node forward
Sum = Sum + P->Element; // print out only the element part
} // stop when there is no next node
return Sum;
}
/********************** Main *****************************************/
int main()
{
/* Making an empty linked list */
Node* L = new Node;
L->Next = NULL;
int i;
int choice;
do {
cout << " What would you like to do ? ";
cout << " 1. Insert data 2. Find data 3. Delete data 4. Print list 5. Count 6. Sum 7. Insert End 8. Exit ";
cin >> choice;
switch (choice)
{
case 1: cout << " Enter number to insert: ";
cin >> i;
InsertBeginning(i, L);
break;
case 2: cout << " Enter number to search for: ";
cin >> i;
if (Find(i, L) == NULL)
cout << " Number NOT in List ";
else
cout << " Number found in list ";
break;
case 3: cout << " Enter number to delete: ";
cin >> i;
Delete(i, L);
break;
case 4: PrintList(L);
break;
case 5:
cout << " You have " << Count(L) << "nodes ";
break;
case 6:
cout << "Sum is" << Sum(L) << " ";
break;
case 7: cout << " Enter number to insert: ";
cin >> i;
InsertEnd(i, L);
break;
}
} while (choice <7);
DeleteWholeList(L);
cin >> i;
return 0;
}
\\C++
Step by Step Solution
There are 3 Steps involved in it
Step: 1
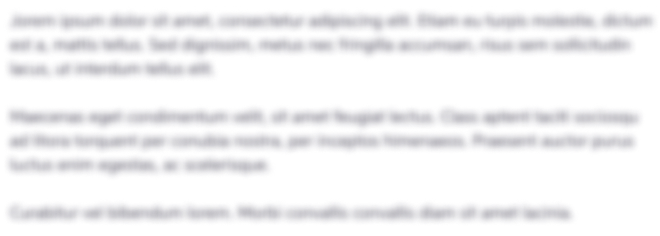
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started