Question
c# ...For this task you are asked to create a NumberList class. This class will need to keep track of a list of any number
c# ...For this task you are asked to create a NumberList class. This class will need to keep track of a list of any number of floating-point values and be able to perform operations on these values.Use a private List
The NumberList class will have the following methods:
void Add(float number)
This method adds number to the end of the list. If myList is a NumberList containing {1, 2} calling myList.Add(3) will mean that myList now contains {1, 2, 3}.
List
This method returns a list containing all the numbers in the NumberList. As you should be using a private List to keep track of these numbers anyway, this method should just return that List.
float Minimum()
This method returns the lowest number in the list.
float Maximum()
This method returns the highest number in the list.
float Sum()
This method returns the sum of all numbers in the list. If myList is a NumberList containing {1, 2, 3} calling myList.Sum() will return 6.
float Average()
This method returns the average/mean of all numbers in the list. If myList is a NumberList containing {1, 2, 3} calling myList.Sum() will return 2, as the sum of those numbers is 6 and 6 divided by 3 (the number of numbers in the list) is 2.
int Count()
This method returns the number of numbers in the list. If myList is a NumberList containing {1, 2, 3} calling myList.Count() will return 3.
int DeleteBelow(float threshold)
This method removes from the list all numbers that are below threshold. If myList is a NumberList containing {1, 2, 3} calling myList.DeleteBelow(1.5) will remove 1, leaving just {2, 3}.
int DeleteAbove(float threshold)
This method removes from the list all numbers that are above threshold. If myList is a NumberList containing {1, 2, 3} calling myList.DeleteAbove(1.5) will remove 2 and 3, leaving just {1}.
int CountBelow(float threshold)
This method counts how many numbers in the list are below threshold. If myList is a NumberList containing {1, 2, 3} calling myList.CountBelow(2.5) will return 2.
int CountAbove(float threshold)
This method counts how many numbers in the list are above threshold. If myList is a NumberList containing {1, 2, 3} calling myList.CountAbove(2.5) will return 1.
GIVEN THE FOLLOWING CODE:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
namespace Numbers { public class NumberList { // Your private List
public void Add(float number) { // ... }
public List
public float Minimum() { // ... } public float Maximum() { // ... } public float Sum() { // ... } public float Average() { // ... } public int Count() { // ... } public void DeleteBelow(float threshold) { // ... } public void DeleteAbove(float threshold) { // ... } public int CountBelow(float threshold) { // ... } public int CountAbove(float threshold) { // ... } } public class Program { private static string ListToString(List
Console.WriteLine("{0} should be 1", myList.Minimum()); Console.WriteLine("{0} should be 5", myList.Maximum()); Console.WriteLine("{0} should be 15", myList.Sum()); Console.WriteLine("{0} should be 3", myList.Average()); Console.WriteLine("{0} should be 5", myList.Count()); Console.WriteLine("{0} should be 2", myList.CountBelow(2.5f)); Console.WriteLine("{0} should be 2", myList.CountAbove(3.5f));
Console.WriteLine("{0} should be 1, 2, 3, 4, 5", ListToString(myList.Numbers())); myList.DeleteBelow(2.5f); Console.WriteLine("{0} should be 3, 4, 5", ListToString(myList.Numbers()));
myList.Add(6); myList.Add(7);
myList.DeleteAbove(4.5f); Console.WriteLine("{0} should be 3, 4", ListToString(myList.Numbers()));
Console.WriteLine(" Press enter to exit."); Console.ReadLine(); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
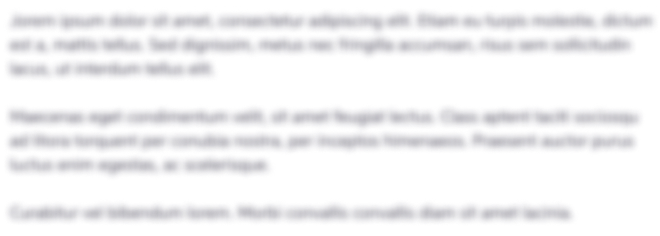
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started