Question
C++ .h programming , need help implementing the updates to four .h files : PLEASE LABEL WHICH CODE GOES TO WHAT FILE IN THE ANSWER!
C++ .h programming , need help implementing the updates to four .h files :
PLEASE LABEL WHICH CODE GOES TO WHAT FILE IN THE ANSWER! THANKS
What is done so far : will provide test cases as well so you know what is right so you dont write something i dont need.
Node.h:
#pragma once
template
class List;
//This class represents a node in a linked list
//Do not modify anything in this file
template
class Node{
friend class List
private:
T value; //value stored by this node
Node * next; //pointer to the next node in list
public:
Node(T v){
value = v;
next = nullptr;
}
};
List.h::
#pragma once
#include
#include
#include "Node.h"
using namespace std;
//This class represents a linked list of node objects
//Do not modify anything in the class interface
template
class List{
private:
Node
int mySize; //size (or length) of this list
public:
List();
~List();
int size();
bool empty();
void insertStart(T);
void insertEnd(T);
void insertAt(T, int);
void removeStart();
void removeEnd();
void removeAt(int);
T getFirst();
T getLast();
T getAt(int);
int find(T);
//Print the name and this list's size and values to stdout
//This function is already implemented (no need to change it)
void print(string name){
cout
cout
cout
Node
while(iterator != nullptr){
cout value
iterator = iterator->next;
}
cout
}
}; //end of class interface (you may modify the code below)
//Implement all of the functions below
//Construct an empty list by initializig this list's instance variables
template
List
}
//Destroy all nodes in this list to prevent memory leaks
template
List
}
//Return the size of this list
template
int List
}
//Return true if this list is empty
//Otherwise, return false
template
bool List
}
//Create a new node with value, and insert that new node
//into this list at start
template
void List
}
//Create a new node with value, and insert that new node
//into this list at end
template
void List
}
//Create a new node with value
template
void List
}
//Remove node at start
//Make no other changes to list
template
void List
}
//Remove node at end
//Make no other changes to list
template
void List
}
//Remove node at position j
//Make no other changes to list
template
void List
}
//Return the value of the first node in the Linked List,
//If no first node, return the default constructed value: T()
template
T List
}
//Return the value of the last node in the Linked List,
//If no first node, return the default constructed value: T()
template
T List
}
//Return the value of the node at position j in the Linked List,
//If no first node, return the default constructed value: T()
template
T List
}
//Return the position of the (first) node whose value is equal to the key
//Otherwise, return -1
template
int List
}
ListStack.h:
#pragma once
//This class represents a Stack implemented via Linked List
//Do not modify anything in the class interface
template
class ListStack{
private:
List
public:
ListStack();
~ListStack();
int size();
bool empty();
void push(T);
T pop();
//Print the name and this ListStack's size and values to stdout
//This function is already implemented (no need to change it)
void print(string name){
stack.print(name);
}
}; //end of class interface (you may modify the code below)
//Implement all of the functions below
//Construct an empty ListStack by initializing this ListStack's instance variables
template
ListStack
}
//Destroy all nodes in this ListStack to prevent memory leaks
template
ListStack
}
//Return the size of this ListStack
template
int ListStack
}
//Return true if this ListStack is empty
//Otherwise, return false
template
bool ListStack
}
//Create a node with value
template
void ListStack
}
//Pop a node from the Stack.
//Note that here that includes both removing the node from the stack
//AND returning its value.
template
T ListStack
}
ListQueue.h:
#pragma once
//This class represents a Queue implemented via Linked List
//Do not modify anything in the class interface
template
class ListQueue{
private:
List
public:
ListQueue();
~ListQueue();
int size();
bool empty();
void enqueue(T);
T dequeue();
//Print the name and this ListQueue's size and values to stdout
//This function is already implemented (no need to change it)
void print(string name){
queue.print(name);
}
}; //end of class interface (you may modify the code below)
//Implement all of the functions below
//Construct an empty ListQueue by initializing this ListQueue's instance variables
template
ListQueue
}
//Destroy all nodes in this ListQueue to prevent memory leaks
template
ListQueue
}
//Return the size of this ListQueue
template
int ListQueue
}
//Return true if this ListQueue is empty
//Otherwise, return false
template
bool ListQueue
}
//Create a new node with value, and insert that new node
//into this ListQueue in its correct position
template
void ListQueue
}
//Dequeue an element from the queue.
//Note that here that means both removing it from the list
//AND returning the value
template
T ListQueue
}
(Main functions for a subset of the test cases : T#.cpp is the main function for test case #, returns 0 when test case passes, returns 1 when test case fails.)
Case 1 :
#include "List.h" #include "Node.h" #include
using namespace std;
int main() { List // This can be an ofstream as well or any other ostream stringstream buffer; // Save cout's buffer here streambuf *sbuf = cout.rdbuf(); // Redirect cout to our stringstream buffer or any other ostream cout.rdbuf(buffer.rdbuf()); // Use cout as usual // cout list1.print("list"); int test[9]; string bob; // This section skips the info printout // section of the print function buffer >> bob; buffer >> bob; buffer >> bob; buffer >> bob; buffer >> bob; buffer >> bob; int test2[] = {4,-8,16,-32,64,-128,256,-512,1024}; for (int i=0; i> bob; test[i] = stoi(bob); // ASSERT_EQ(test[i],test2[i]); } // When done redirect cout to its old self cout.rdbuf(sbuf); for (int i=0; i Case 16 : #include "ListStack.h" #include using namespace std; int main() { ListStack // This can be an ofstream as well or any other ostream stringstream buffer; // Save cout's buffer here streambuf *sbuf = cout.rdbuf(); // Redirect cout to our stringstream buffer or any other ostream cout.rdbuf(buffer.rdbuf()); // Use cout as usual // cout stack3.print("list"); char test[12]; char test2[] = {'z', 'p', 'a', 'b', 'c', 'e', 'h', 'i', 'j', 'k', 'l', 'm'}; string bob; // This section skips the info printout // section of the print function buffer >> bob; buffer >> bob; buffer >> bob; buffer >> bob; buffer >> bob; buffer >> bob; for (int i=0; i> bob; // test[i] = stoi(bob); test[i] = bob.at(0); } // When done redirect cout to its old self cout.rdbuf(sbuf); for (int i=0; i PLEASE LABEL WHICH CODE GOES TO WHAT FILE IN THE ANSWER! THANKS
Step by Step Solution
There are 3 Steps involved in it
Step: 1
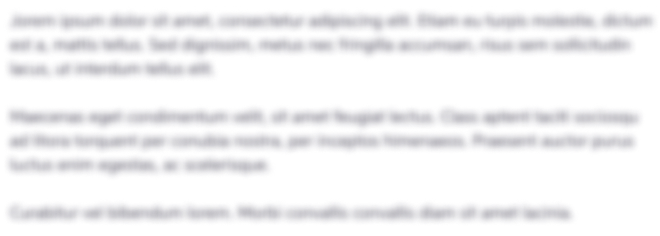
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started