Question
C++ Help Calculating Primes (Pointers) Rating best and helpful solution thumbs up!!! Compile code first please. Task: You must construct class functions that can be
C++ Help Calculating Primes (Pointers)
Rating best and helpful solution thumbs up!!! Compile code first please.
Task:
You must construct class functions that can be used to do various generation of and calculations on prime numbers. The class and main function are already given. Use of vectors not allowed.
Note that both prime numbers and arrays only make sense with respect to positive values. That is to say, there are no negative prime numbers and there is no way to have a negative number of array elements, so we will be using unsigned int heavily in this assignment.
With respect to the implementation, keep this in mind:
You must support the wrap around of prime numbers with some logic. For example, the largest prime that can be stored in a 32 bit unsigned integer is 4294967291, as such if we ever need the next prime after that, it will be 2. Similarly, the prime previous to 2 is 4294967291.
You will need to reference the maximum value of the unsigned int. To do that you must include the climits header which has the UINT_MAX constant defined.
As a restriction you are not allowed to use brackets [ ] in the implementation except when required with the new and delete operators -- use pointer & offset notation instead.
The specific members should be implemented as follows:
primes constructor: The first parameter refers to the starting value, the second parameter refers to the total number of primes to store. This should dynamically allocate a one-dimensional array of the specified size and begin filling it with prime numbers beginning with the specified starting value and incrementing until it is full. The default values are to start at 2 and fill it with 10 primes.
primes copy constructor: Should perform a deep copy
primes destructor: Should deallocate memory.
display: Display each prime in the array along with which position it is in.
renew: Should deallocate memory of the existing array then reallocate it with the appropriate starting value and total number of primes to store, same as the constructor
prime_count: should return the number of primes stored in the array
static methods: You do not use the static keyword before a method in the implementation
next_prime: If a value is provided, return the next prime after that value. If no value is provided (default parameter 0) then return the next prime after current. current should be equal to the value returned when this function is over.
previous_prime: If a value is provided, return the previous prime before that value. If no value is provided (default parameter 0) then return the previous prime before current. current should be equal to the value returned when this function is over.
is_prime: returns true if the value is prime, false if it is not
current: stores the current prime for use with next_prime/previous_prime
You will need to place this line in your implementation file:
unsigned int primes::current = 2;
Variables:
parray: The pointer for the dynamically allocated array of primes
count: A count of how many primes are stored in the array (i.e. the size of the array)
Sample terminal session:
My code (class and main function already given, just need functions):
#include
using namespace std;
class primes { public: primes(unsigned int = 2, unsigned int = 10); primes(const primes&); ~primes(); void display() const; void renew(unsigned int, unsigned int); unsigned int prime_count() const; static unsigned int next_prime(unsigned int = 0); static unsigned int previous_prime(unsigned int = 0); static bool is_prime(unsigned int); static unsigned int current;
private: unsigned int *parray, count; };
unsigned int primes::current = 2;
//int x refers to starting value //int y refers to total number of primes to store primes::primes(unsigned int x, unsigned int y) { }
//Perform Deep Copy primes::primes(const primes&) { /* for (int i = 0; i
//Should deallocate memory primes::~primes() {
}
//Display each prime in the array along with which position it is in void primes::display() const {
}
//Should deallocate memory of the existing array then reallocate it //with the appropriate starting value and total number of primes to store, //same as the constructor void primes::renew(unsigned int x, unsigned int y) {
}
//Should return the number of primes stored in the array unsigned int primes::prime_count() const {
}
//If a value is provided, return the next prime after that value. //If no value is provided (default parameter 0) then return the next prime // after current. current should be equal to the //value returned when this function is over unsigned int primes::next_prime(unsigned int x) {
}
//If a value is provided, return the previous prime before that value. //If no value is provided (default parameter 0) then return the previous prime //before current. current should equal to the //value returned when this function is over. unsigned int primes::previous_prime(unsigned int x) {
}
//Returns true if the value is prime, false if it is not bool primes::is_prime(unsigned int x) {
}
int main() { primes p1; cout (williams bobby 071 time /williams.out There are 10 primes in pl Element #0: 2 Element #1: 3 Element #2 : 5 Element #3: 7 Element 4: 11 Element #5: 13 Element #6: 17 Element #7: 19 Element 8: 23 Element #9: 29 There are 10 primes in p2 Element #0: 83 Element 1 89 lement #2: 97 Element #3: 101 Element #4: 103 Element 5: 107 Element #6: 109 Element #7: 113 Element #8: 127 Element 9: 131 There are 5 prines in p3 Element #0: 100003 Element #1: 10001 9 Element 2: 100043 lement #3: 100049 Element #4 : 100057 There are 3 prinmes in p1 Element 0: 60013 lement #1: 60017 Element #2 : 60029 There are 3 primes in p4 Element 0: 60013 lement #1: 60017 Element #2: 60029 Current value: 2 999983 999979 1.3 Nor prime! 1 18 NOT prine! 2 is prime! 3 is prime! 4 is NOT prine 4294967290 1s NOT prime! 1294967291 is prime! 4294967291 4294967291 4294967279 There are 10 primes in p5 Element #0: 4294967279 Element #1: 4294967291 Element #2: 2 Element #3: 3 Element : 5 Element #5: 7 Element #6: 11 Element #7: 13 Element 8: 17 Element #9: 19 There are 1 primos in p!5 Element #0: 2 real Om36.4285 user m36.3285 OmO.073a williamsebobby 071s real time could be To reiterate, the only time that matters above is the combination of usersys time. artificially high due to other people running processes at the same time
Step by Step Solution
There are 3 Steps involved in it
Step: 1
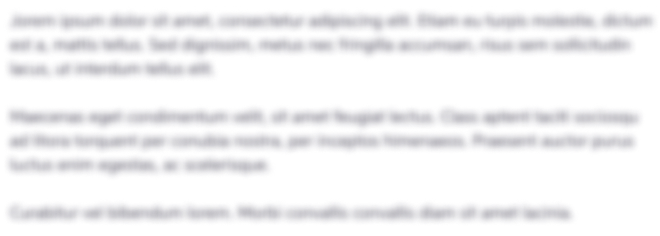
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started