Question
C# I need help to convert my code into 2 methods: 2 METHODS: decimal CalcAndPrintInvoiceBody(int qty, bool discount, bool promotionPrize) Performs the necessary calculations to
C# I need help to convert my code into 2 methods:
2 METHODS:
-
decimal CalcAndPrintInvoiceBody(int qty, bool discount, bool promotionPrize)
Performs the necessary calculations to enable printing the main part of the invoice, and then prints it. The return value represents the total invoice amount. Notes:
-
Call this method from Main().
-
Print the shipping address in Main() before you call this method.
-
Determine whether the loyalty discount is applicable in Main() before you call this method.
-
Conduct the contest to determine whether the user will receive the promotional prize in Main()
before you call this method.
-
The method must produce the following elements of output on the invoice:
-
o Quantity o Costperanvil o Subtotal o Loyaltydiscountamount(if applicable) o Taxable amount o Sales tax o Total o The promotional gift congratulatory message
After the method concludes and control is returned to Main(), print the total message: (Your total today is {total:C}. Thanks for shopping with Acme!), where the value of the variable total is the returned value from CalcAndPrintInvoiceBody
-
decimal CalcAndPrintInvoiceBody(int qty, bool discount, bool promotionPrize)
Performs the necessary calculations to enable printing the main part of the invoice, and then prints it. The return value represents the total invoice amount. Notes:
-
Call this method from Main().
-
Print the shipping address in Main() before you call this method.
-
Determine whether the loyalty discount is applicable in Main() before you call this method.
-
Conduct the contest to determine whether the user will receive the promotional prize in Main()
before you call this method.
-
The method must produce the following elements of output on the invoice:
-
decimal GetAnvilPrice(int qty)
Returns the price per anvil based on quantity requested.
Notes:
-
This method must be called from CalcAndPrintInvoiceBody.
-
You must use the array-based version of accurate and efficient range checking.
2)CODE:
//Calculate subtotal: price * qty using volume discount if (numberOfAnvils
subTotal = numberOfAnvils * (decimal)quantityBasedCostPerAnvil;
//Subtract loyalty discount if applicable loyaltyDiscountAmt = loyaltyDiscount ? loyaltyDiscountAmt = Decimal.Round(subTotal * -(decimal)LoyaltyDiscountFactor, 2) : 0; //loyaltyDiscountAmt = loyaltyDiscount ? loyaltyDiscountAmt = subTotal * (decimal)-LoyaltyDiscountFactor : 0;
taxableAmount = subTotal + loyaltyDiscountAmt;
//Add tax and calculate final total tax = Decimal.Round((subTotal + loyaltyDiscountAmt) * (decimal)SalesTax, 2); //tax = (subTotal + loyaltyDiscountAmt) * (decimal)SalesTax;
shipping = ShippingCostPerAnvil * numberOfAnvils; total = taxableAmount + tax + shipping;
//Display invoice Write(" Press any key to display invoice. . ."); ReadKey(); Write(" ");
WriteLine(ACMEBanner1); WriteLine(ACMEBanner2); WriteLine("CUSTOMER INVOICE"); WriteLine(" SHIP TO:"); WriteLine($"\t\t{fullName}"); WriteLine("\t\t" + streetAddress); WriteLine("\t\t{0}", city); WriteLine("\t\t{0}", stateAbbreviation); WriteLine("\t\t{0}", zipCode);
WriteLine($" Quantity:\t\t\t{numberOfAnvils,10}"); WriteLine($"Cost per anvil:\t\t\t{quantityBasedCostPerAnvil,10:C}"); WriteLine($"Subtotal: \t\t\t{subTotal,10:C}");
if (loyaltyDiscount) { WriteLine($"Less {LoyaltyDiscountFactor:P0} Futility Club: \t{loyaltyDiscountAmt,11:C}"); WriteLine($"Taxable amount: \t\t{taxableAmount,10:C}"); }
WriteLine($"Sales Tax: \t\t\t{tax,10:C}"); WriteLine($"Shipping: \t\t\t{shipping,10:C}");
WriteLine("\t\t\t\t__________"); WriteLine($"TOTAL: \t\t\t\t{total,10:C}");
//Promotional gift? if (sendGift) { WriteLine(" And congratulations on winning FREE Invisible Paint!!!"); }
WriteLine(ACMEBanner1);
//Goodbye!
WriteLine(" Your total today is {0:C}. Thanks for shopping with Acme!", total); WriteLine(" And don\'t forget: Acme anvils fly farther, drop faster, and land harder than " + "any other anvil on the market!");
Write(" Ready to take another order? "); } while (ReadLine().ToUpper() == "Y");
WriteLine(" Okay, then. Buh-bye!");
}
using System; using static System.Console; namespace OrderAnvilsSolution { class OrderAnvils3 { static void Main(string[] args) { int numberOfAnvils; int guess; int contestNumber: string fullName; string streetAddress; string city: string stateAbbreviation; ; string zipCode = ""; 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 bool fieldValidationSuccess = false; bool loyaltyDiscount; bool sendGift; decimal subTotal; decimal tax; decimal shipping: decimal total; decimal loyaltyDiscountAmt; decimal taxableAmount; double quantityBasedCostPerAnvil; const double SalesTax = 0.0995; //9.95% sales tax reduction due to election year. const decimal CostPerAnvillieri = 80M; //'M' because it's a variable of type decimal const decimal CostPerAnvillier2 = 72.35M; const decimal CostPerAnvillier3 = 67.99M; const decimal Shipping CostPerAnvil = 99M; const double LoyaltyDiscountFactor = 0.15; //15% discount const int GameMin = 1; const int GameMax = 5; const string ACMEBanneri = "********** const string ACMEBanner2 = "************************ ACME Anvils Corporation *********** const string ACMEBanner3 = "\a********* ************** NEW ORDER *********** 80 //OPTIONAL BONUS: Add a state array. string[] states = new string[] { "AK", "WA", "OR", "CA", "AZ", "NM", "CO", "UT" }; Random contestNumbers = new Random(); //Instantiate random number generator //Welcome message WriteLine(ACMEBanner2); WriteLine("Supporting the animation industry for 70 years and counting!"); //Start the outer loop do { WriteLine(" Countdown to Order"); for (int i = 3; i > 0; i--) { WriteLine(i); System.Threading.Thread. Sleep(1000); //1000 milliseconds == 1 second } //Collect input data WriteLine(ACMEBanner3); WriteLine(" \ali, I'mStep by Step Solution
There are 3 Steps involved in it
Step: 1
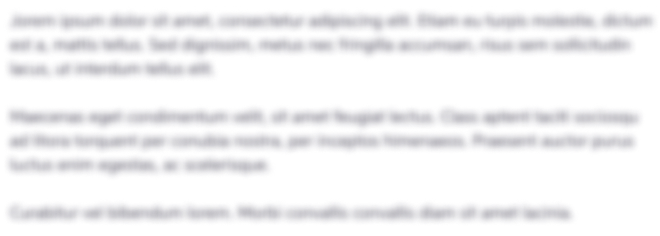
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started