Question
(C++) I need to write a class that can take in a HuffmanNode and use it in a Linked Binary Tree. I have written my
(C++) I need to write a class that can take in a HuffmanNode and use it in a Linked Binary Tree. I have written my own class trying to implement this in "LinkedBinaryTree.hpp", but it seems I don't know how to add new nodes and add child nodes to it. Could you help me add any member functions I may be missing/correct any mistakes I made, and make a main() program that will help me understand this better. My code is below.
// LINKED BINARY TREE FILE --- What I wrote
#ifndef LINKEDBINARYTREE_H_
#define LINKEDBINARYTREE_H_
#include "HuffmanBase.hpp"
#include
//Forward Declerations
template
template
/**********************************************************************
Tree Node Class
**********************************************************************/
template
class TNode {
private:
E elem; // Element value
TNode
TNode
TNode
//Default Constructor
TNode
TNode
//Provides friends access.
friend class LinkedBinaryTree
friend class Position
};
/**********************************************************************
TreeNode Position Class
**********************************************************************/
template
class Position {
private:
//Pointer to the TNode.
TNode
public:
//Constructor
Position(TNode
//Gets Element
E& operator*() { return v->elem; }
//Gets left child
Position left() const { return Position(v->left); }
//Gets right child
Position right() const { return Position(v->right); }
//Gets Parent
Position parent() const { return Position(v->par); }
//Root of the Tree?
bool isRoot() const { return (v->par == NULL); }
//An external node?
bool isExternal() const { return (v->left == NULL && v->right == NULL); }
//Provides friends access.
friend class LinkedBinaryTree
typedef typename std::list
};
/**********************************************************************
Linked Binary Tree Class
**********************************************************************/
template
class LinkedBinaryTree {
public:
LinkedBinaryTree(); //Constructor
int size() const; //Number of nodes.
bool empty() const; //Is tree empty?
Position
std::list
void addRoot(); //Add root to empty tree.
void addRoot(const E& e); //Add root to empty tree.
void expandExternal(const Position
Position
protected:
//Utility funtions
void preorder(TNode
private:
TNode
int nodeCounter;
};
#endif // !LINKEDBINARYTREE_H_
template
inline LinkedBinaryTree
template
inline int LinkedBinaryTree
{
return nodeCounter;
}
template
inline bool LinkedBinaryTree
{
return (size() == 0);
}
template
inline Position
{
return Position
}
template
inline std::list
{
std::list
//Preorder Traversal.
preorder(_root, pl);
return std::list
}
template
inline void LinkedBinaryTree
{
_root = new TNode
nodeCounter = 1;
}
template
inline void LinkedBinaryTree
{
_root = new TNode
_root->elem = e;
nodeCounter = 1;
}
template
inline void LinkedBinaryTree
{
//p's node.
TNode
//Add a new left child.
v->left = new TNode
//Sets v to be it's parent.
v->left->par = v;
//Add a new right child.
v->right = new TNode
//Sets v to be it's parent.
v->right->par = v;
//Increments nodeCounter by two.
nodeCounter += 2;
}
template
inline Position
{
//Gets p's node and parent.
TNode
TNode
TNode
//Child of root?
if (v == _root) {
//Make sibling root.
_root = sibling;
sibling->par = NULL;
}
else {
//w's grandparent.
TNode
//Replace parent and sibling.
if (v == gPar->left) {
gPar->left = sibling;
}
else {
gPar->right = sibling;
}
sibling->par = NULL;
}
//Deletes removed TNodes.
delete w;
delete v;
//Decrements nodeCounter.
nodeCounter -= 2;
//Returns Sibling.
return Position
}
template
inline void LinkedBinaryTree
{
//Adds this node.
pl.push_back(Position
//Traverse left subtree
if (v->left != NULL) {
preorder(v->left, pl);
}
if (v->right != NULL) {
preorder(v->right, pl);
}
}
// HUFFMAN NODE HPP
class HuffmanNode { public: HuffmanNode(char c, size_t f, HuffmanNode *p, HuffmanNode *l, HuffmanNode *r); HuffmanNode(char c, size_t f);
char getCharacter() const; size_t getFrequency() const;
bool isLeaf() const; bool isBranch() const; bool isRoot() const;
class Compare { public: Compare(bool lessThan = true) : lessThan(lessThan) {}; bool operator()(const HuffmanNode &n1, const HuffmanNode &n2) const; bool operator()(const HuffmanNode *n1, const HuffmanNode *n2) const;
private: bool lessThan; };
public: HuffmanNode *parent; HuffmanNode *left; HuffmanNode *right;
private: char character; size_t frequency; };
//HUFFMAN NODE CPP
#include "HuffmanBase.hpp"
HuffmanNode::HuffmanNode(char c, size_t f, HuffmanNode *p, HuffmanNode *l, HuffmanNode *r) {
character = c;
frequency = f;
parent = p;
left = l;
right = r;
}
HuffmanNode::HuffmanNode(char c = '\0', size_t f = 0) {
character = c;
frequency = f;
parent = nullptr;
left = nullptr;
right = nullptr;
}
char HuffmanNode::getCharacter() const
{
return character;
}
size_t HuffmanNode::getFrequency() const
{
return frequency;
}
bool HuffmanNode::isLeaf() const
{
return (left == nullptr && right == nullptr);
}
bool HuffmanNode::isBranch() const
{
return (left != nullptr || right != nullptr);
};
bool HuffmanNode::isRoot() const
{
return (parent == nullptr);
};
bool HuffmanNode::Compare::operator()(const HuffmanNode &n1, const HuffmanNode &n2) const
{
if (n1.frequency == n2.frequency) {
return lessThan ? n1.character < n2.character : n1.character >= n2.character;
} else {
return lessThan ? n1.frequency < n2.frequency : n1.frequency >= n2.frequency;
}
}
bool HuffmanNode::Compare::operator()(const HuffmanNode *n1, const HuffmanNode *n2) const
{
return operator()(*n1, *n2);
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
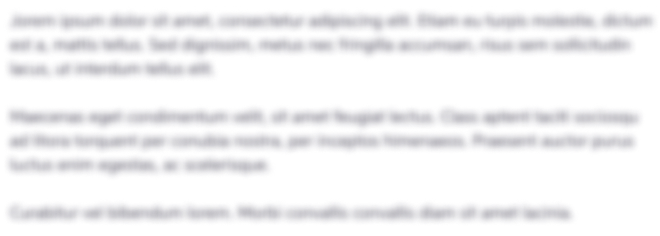
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started