Question
C++ I only need help with the FIX ME portion, can you also explain why using that call or syntax is appropriate for each FIX
C++ I only need help with the FIX ME portion, can you also explain why using that call or syntax is appropriate for each FIX ME portion.
// define a structure to hold bid information
struct Bid {
string bidId; // unique identifier
string title;
string fund;
double amount;
Bid() {
amount = 0.0;
}
};
// Internal structure for tree node
// Add node declaration
struct node {
int info;
struct node *left;
struct node *right;
} *root;
class BinarySearchTree {
private:
Node* root;
void addNode(Node* node, Bid bid);
void inOrder(Node* node);
Node* removeNode(Node* node, string bidId);
public:
BinarySearchTree();
virtual ~BinarySearchTree();
void InOrder();
void Insert(Bid bid);
void Remove(string bidId);
Bid Search(string bidId);
};
/**
* Default constructor
*/
BinarySearchTree::BinarySearchTree() {
// FixMe (1): initialize housekeeping variables
//root is equal to nullptr
}
/**
* Destructor
*/
BinarySearchTree::~BinarySearchTree() {
// recurse from root deleting every node
}
/**
* Traverse the tree in order
*/
void BinarySearchTree::InOrder() {
// FixMe (2): In order root
// call inOrder fuction and pass root
}
/**
* Traverse the tree in post-order
*/
void BinarySearchTree::PostOrder() {
// FixMe (3): Post order root
// postOrder root
}
/**
* Traverse the tree in pre-order
*/
void BinarySearchTree::PreOrder() {
// FixMe (4): Pre order root
// preOrder root
}
/**
* Insert a bid
*/
void BinarySearchTree::Insert(Bid bid) {
// FIXME (5) Implement inserting a bid into the tree
// if root equarl to null ptr
// root is equal to new node bid
// else
// add Node root and bid
}
/**
* Remove a bid
*/
void BinarySearchTree::Remove(string bidId) {
// FIXME (6) Implement removing a bid from the tree
// remove node root bidID
}
/**
* Search for a bid
*/
Bid BinarySearchTree::Search(string bidId) {
// FIXME (7) Implement searching the tree for a bid
// set current node equal to root
// keep looping downwards until bottom reached or matching bidId found
// if match found, return current bid
// if bid is smaller than current node then traverse left
// else larger so traverse right
Bid bid;
return bid;
}
/**
* Add a bid to some node (recursive)
*
* @param node Current node in tree
* @param bid Bid to be added
*/
void BinarySearchTree::addNode(Node* node, Bid bid) {
// FIXME (8) Implement inserting a bid into the tree
// if node is larger then add to left
// if no left node
// this node becomes left
// else recurse down the left node
// else
// if no right node
// this node becomes right
//else
// recurse down the left node
}
void BinarySearchTree::inOrder(Node* node) {
// FixMe (9): Pre order root
//if node is not equal to null ptr
//InOrder not left
//output bidID, title, amount, fund
//InOder right
}
void BinarySearchTree::postOrder(Node* node) {
// FixMe (10): Pre order root
//if node is not equal to null ptr
//postOrder left
//postOrder right
//output bidID, title, amount, fund
}
void BinarySearchTree::preOrder(Node* node) {
// FixMe (11): Pre order root
//if node is not equal to null ptr
//output bidID, title, amount, fund
//postOrder left
//postOrder right
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
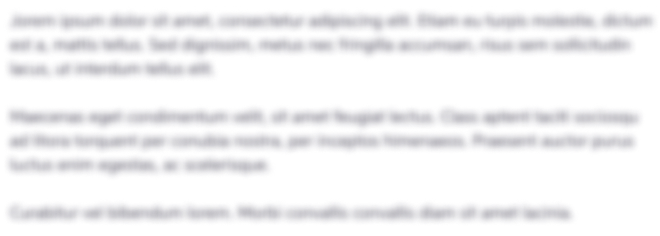
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started