Question
C++: Implement this into a linked list #include #include #include #include using namespace std; struct PERSON{ string SSN; string fullName; }; //Checks if array needs
C++:
Implement this into a linked list
#include
struct PERSON{ string SSN; string fullName; };
//Checks if array needs to be resized to the inputs 'i' and 'd' which will either //double the size of the array or divide the array by half. void checkArraySize(struct PERSON *&arrayData, int &arraySize, char option){ if(option == 'i'){ if (arrayData[arraySize - 1].SSN.length() != 0){ // If last element in array contains an object struct PERSON *newArray = new PERSON[arraySize * 2];//temporary array with double the size // Move data from old array to the new larger array for (int x = 0; x < arraySize; x++) { newArray[x] = arrayData[x]; } delete[] arrayData; arrayData = newArray; arraySize = arraySize * 2; //double the size of the array } } // Check if the array needs to be decreased else if(option == 'd'){ if (arrayData[(arraySize/4) - 1].SSN.length() == 0){ // If the array is less than 1/4 fullName struct PERSON *newArray = new PERSON[arraySize/2]; // Create a new temp array with half the size // Transfer data from the old array to the new smaller array for (int x = 0; x < arraySize; x++) { if (arrayData[x].SSN.length() == 0) { break; } newArray[x] = arrayData[x]; } delete [] arrayData; // Release the old array arrayData = newArray; // the old array with the new smaller array arraySize /= 2; // Update the array size variable } } }
//Adds the data to the end of the data in the array. If the provided data has duplicated SSN with an existing //entry, the software will discard the provided data, if it does not not, it will increase the insertCount by 1. void insertInfo(struct PERSON *&arrayData, int &arraySize, fstream &input, int &index, int &insertCount){ // Declare variables string firstName, lastName; string personSSN; string skipInput; input >> personSSN; // Input the SSN of the entry that needs to be inserted into the array checkArraySize(arrayData, arraySize, 'i'); // Check to see if the array is full before attempting to insert //a new entry into the array // Loop through the array for(int x = 0; x < arraySize; x++){ if (personSSN == arrayData[x].SSN) { // Check if the ssn is already in the array input >> skipInput; input >> skipInput; break; }else if(arrayData[x].SSN.length() == 0){ // If that ssn is NOT in the array then add that entry input >> firstName >> lastName; arrayData[index].SSN = personSSN; arrayData[index].fullName = firstName + " " + lastName; index++; insertCount++; break; } } }
//Deletes the entry with given SSN and name from the array. If SSN does not match any record in the array, //the software breaks. If there is a match in the array, delete the record from the array, increase the delete counter by one. void deleteInfo(struct PERSON *&arrayData, int &arraySize, fstream &input, int &index, int &deleteCount) { string personSSN; input >> personSSN; // Input the ssn of the entry that needs to be deleted from the array // Iterate through the array for (int indexOfArray = 0; indexOfArray < arraySize; indexOfArray++) { if (arrayData[indexOfArray].SSN.length() != 0) { // Check if element is not empty if (personSSN == arrayData[indexOfArray].SSN) { // Check if the inputed ssn matches any entries // Starting at the index of the entry to be deleted // shift all of the entries 1 to the left // which overwrites the entry to be deleted while(indexOfArray < arraySize && arrayData[indexOfArray].SSN.length() != 0) { if (indexOfArray == arraySize - 1) { arrayData[indexOfArray].SSN = ""; arrayData[indexOfArray].fullName = ""; } else { arrayData[indexOfArray].SSN = arrayData[indexOfArray + 1].SSN; arrayData[indexOfArray].fullName = arrayData[indexOfArray + 1].fullName; } indexOfArray++; } checkArraySize(arrayData, arraySize, 'd'); // Check to see if the array is more than 3/4 empty //and should be downsized // Update counter variables deleteCount++; index = indexOfArray - 1; break; } } else { break; } } } //The software searches the given SSN and name in the array. If the there is a match in the array, //increases the retrieval counter by one. void retrieveInfo(struct PERSON *&arrayData, int &arraySize, fstream &input, int &retrieveCount) { // Declare variables string personSSN; input >> personSSN; // Input ssn of entry to be retrieved // Iterate through the array for(int x = 0; x < arraySize; x++){ if(personSSN == arrayData[x].SSN){ // Check if the ssn matches an entry in the array retrieveCount++;// Update the counter }else if(arrayData[x].SSN.length() == 0){ break; } } }
//prints the insertion, deletion, and retrieval count as well as the array size and the number of items in that //array void printData(struct PERSON *arrayData, int arraySize, int insertCount, int deleteCount, int retrieveCount){ cout << "The Number of Valid Insertation: " << insertCount << endl; cout << "The Number of Valid Deletion: " << deleteCount << endl; cout << "The Number of Valid Retrieval: " << retrieveCount << endl; cout << "Item numbers in the array: " << (insertCount - deleteCount) << endl; cout << "Array Size is: " << arraySize << endl; }
int main(int argc, char *argv[]) { int recordSize = 1000; struct PERSON *record = new PERSON[recordSize]; int index = 0; int insertionCount = 0; int deletionCount = 0; int retrievalCount = 0; string option; // Possible values 'i', 'd', 'r' string personSSN; // SSN to search for string firstName, lastName; //Name of person
fstream input(argv[1]); while(!input.eof()){ input >> option; if(option == "i"){ insertInfo(record, recordSize, input, index, insertionCount); } else if(option == "d"){ deleteInfo(record, recordSize, input, index, deletionCount); } else if(option == "r"){ retrieveInfo(record, recordSize, input, retrievalCount); } if (!input){ break; } } input.close(); printData(record, recordSize, insertionCount, deletionCount, retrievalCount); }
Text file:
i 586412373 NICOLA EVANGELISTA i 177228167 MEAGAN LEKBERG i 586412373 JEFF DUTTER i 760846483 KITTY MANZANERO i 061899135 CATHERIN MCCREIGHT i 087300880 CARMA KULHANEK i 177264549 VALERY KOSAKOWSKI i 210044984 SHEILAH MONGES d 760846483 KITTY MANZANERO r 760846483 KITTY MANZANERO r 007980295 DELPHIA SIMISON i 493515916 VERONIKA TADENA d 401991909 MCKINLEY WESTERFELD i 793267575 TEMIKA MESHEW i 319373939 MARGIT EBLIN
Step by Step Solution
There are 3 Steps involved in it
Step: 1
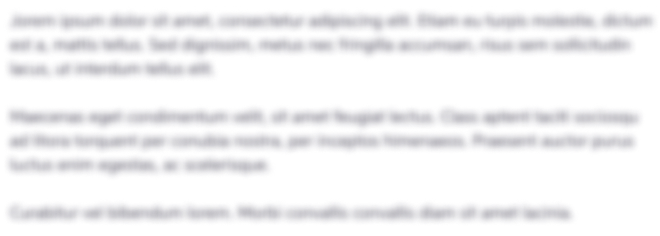
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started