Question
C++ Inheritance Help Need help understanding what is going on when I comment out // ovals[i].draw(); and replace it with Shape *s = &ovals[i]; s
C++ Inheritance Help
Need help understanding what is going on when I comment out // ovals[i].draw(); and replace it with Shape *s = &ovals[i]; s -> draw(); in the display() function in the main.cpp. I see the ovals are gone when I run the program but why is it happening?
Shape.h
#pragma once
#include
using std::string;
class Shape
{
protected:
string name;
int x, y, width, height;
public:
Shape(string name, int x, int y, int width, int height);
Shape();
~Shape();
void draw();
string getName();
int getX();
int getY();
int getWidth();
int getHeight();
bool pointInShape(int pointX, int pointY);
};
Shape.cpp
#include "Shape.h"
#include "gl/glut.h"
#include "gl/gl.h"
#include "gl/glu.h"
Shape::Shape()
{
name = " ";
x = 0;
y = 0;
width = 10;
height = 10;
}
Shape::Shape(string name, int x, int y, int width, int height) :
name(name), x(x), y(y), width(width), height(height)
{
}
Shape::~Shape()
{
}
void Shape::draw()
{
glColor3f(1, 0, 0);
glBegin(GL_LINES);
glVertex2f(108, 135);
glVertex2f(140, 157);
glVertex2f(145, 135);
glVertex2f(100, 157);
glEnd();
}
string Shape::getName()
{
return name;
}
int Shape::getX()
{
return x;
}
int Shape::getY()
{
return y;
}
int Shape::getWidth()
{
return width;
}
int Shape::getHeight()
{
return height;
}
bool Shape::pointInShape(int pointX, int pointY)
{
if ((pointX >= x) && (pointX < x + width) && (pointY >= y) && (pointY <= y + height))
return true;
return false;
}
Rectangle.h
#pragma once
#include "Shape.h"
#include
using std::string;
class Rectangle: public Shape
{
public:
Rectangle(string name, int x, int y, int width, int height);
Rectangle();
~Rectangle();
void draw();
};
Rectangle.cpp
#include "Rectangle.h"
#include "gl/glut.h"
#include "gl/gl.h"
#include "gl/glu.h"
Rectangle::Rectangle() : Shape()
{
name = "Rectangle";
x = 0;
y = 0;
width = 10;
height = 10;
}
Rectangle::Rectangle(string name, int x, int y, int width, int height) : Shape(name, x, y, width, height)
{
}
Rectangle::~Rectangle()
{
}
void Rectangle::draw()
{
glColor3f(0, 0, 1);
glBegin(GL_QUADS);
glVertex2f(x, y);
glVertex2f(x + width, y);
glVertex2f(x + width, y + height);
glVertex2f(x, y + height);
glEnd();
}
Oval.h
#pragma once
#include "Shape.h"
#include
using std::string;
class Oval: public Shape
{
public:
Oval(string name, int x, int y, int width, int height);
Oval();
~Oval();
void draw();
};
Oval.cpp
#include "Oval.h"
#include "gl/glut.h"
#include "gl/gl.h"
#include "gl/glu.h"
Oval::Oval() : Shape()
{
name = "Oval";
x = 0;
y = 0;
width = 10;
height = 10;
}
Oval::Oval(string name, int x, int y, int width, int height) : Shape(name, x, y, width, height)
{
}
Oval::~Oval()
{
}
void Oval::draw()
{
int centerX, centerY;
centerX = x + width / 2;
centerY = y + height / 2;
glColor3f(1, 0, 0);
glBegin(GL_TRIANGLE_FAN);
glVertex2f(centerX, centerY);
for (float angle = 0; angle < 360; angle += 1)
{
glVertex2f(centerX + sin(angle) * width / 2, centerY + cos(angle) * height / 2);
}
glEnd();
}
Main.cpp
#include "gl/glut.h"
#include "gl/gl.h"
#include "gl/glu.h"
#include
#include
#include
#include "Oval.h"
#include "Rectangle.h"
using std::string;
void init();
void display();
void handleButton(int button, int state, int x, int y);
void printText(int x, int y, string str);
void *font = GLUT_BITMAP_TIMES_ROMAN_24;//GLUT_STROKE_ROMAN;
const int WIDTH = 720;
const int HEIGHT = 480;
static int screenx = 0;
static int screeny = 0;
Oval ovals[3];
Rectangle rects[3];
int main(int argc, char** argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB | GLUT_MULTISAMPLE | GLUT_DEPTH);
glutInitWindowSize(WIDTH, HEIGHT);
glutInitWindowPosition(0, 0);
glutCreateWindow("Just A Window");
init();
glutDisplayFunc(display);
glutMouseFunc(handleButton);
glutMainLoop();
return 0;
}
void init()
{
glClearColor(0.0, 0.0, 0.0, 0.0);
glColor3f(1.0, 1.0, 1.0);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(screenx, screenx + WIDTH, screeny + HEIGHT, screeny);
ovals[0] = Oval("Oval 1", 10, 10, 100, 100);
ovals[1] = Oval("Oval 2", 200, 10, 150, 50);
ovals[2] = Oval("Oval 3", 10, 200, 200, 100);
rects[0] = Rectangle("Rect 1", 400, 50, 100, 75);
rects[1] = Rectangle("Rect 2", 200, 300, 75, 100);
rects[2] = Rectangle("Rect 3", 10, 320, 120, 40);
}
void display()
{
glClear(GL_COLOR_BUFFER_BIT);
for (int i = 0; i < 3; i++)
{
//ovals[i].draw();
Shape *s = &ovals[i];
s->draw();
rects[i].draw();
}
Shape s("Shape", 150, 150, 50, 50);
s.draw();
glutSwapBuffers();
glFlush();
return;
}
void handleButton(int button, int state, int x, int y)
{
static int index = -1;
if (button == GLUT_LEFT_BUTTON)
{
if (state == GLUT_DOWN)
{
for (int i = 0; i < 3; i++)
{
if (ovals[i].pointInShape(x, y))
{
glClear(GL_COLOR_BUFFER_BIT);
glColor3f(1, 1, 1);
printText(x + 30, y + 30, "You clicked " + ovals[i].getName());
glutSwapBuffers();
glFlush();
}
else if (rects[i].pointInShape(x, y))
{
glClear(GL_COLOR_BUFFER_BIT);
glColor3f(1, 1, 1);
printText(x + 30, y + 30, "You clicked " + rects[i].getName());
glutSwapBuffers();
glFlush();
}
}
}
if (state == GLUT_UP)
{
display();
}
}
}
void printText(int x, int y, string str)
{
int i, length;
glRasterPos2f(x, y);
length = str.length();
for (i = 0; i < length; i++)
{
glutBitmapCharacter(font, str[i]);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
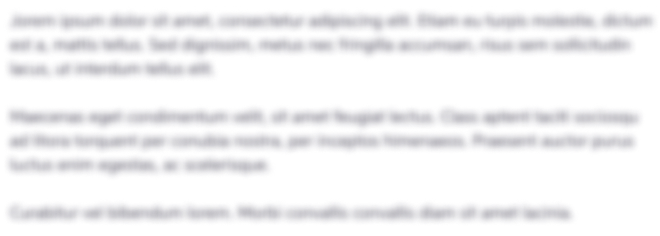
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started