Question
C++ Inventory Class. Design an Inventory class. The older textbook lists 4 private variables and 10 public member functions. It is a good start, however,
C++
Inventory Class.
Design an Inventory class.
The older textbook lists 4 private variables and 10 public member functions. It is a good start, however, one of the private variables is redundant - it is dependent on two other related private variables. This allows the data to become out-of-sync or "stale". This is not good design practice "Avoiding Stale Data". Identify the redundant variable, and eliminate it. Eliminate and/or modify related member functions to avoid the creation of stale or redundant data in the class. Implement the Inventory Class and use it as described in the textbook.
The newer textbooks corrected the problem of the redundant private data variable, and only lists 3 private variables (itemNumber, quantity, cost) and 9 public member functions. The textbook, "Avoiding Stale Data" explains why is it not good design practice to have dependencies between member variables. You should implement the Inventory Class and use it as described in the textbook. Despite removing the redundant private variable, the 8ed and 9ed still say to initialize the removed variable in constructor #2 (last sentence on page 499, 8ed; page 501, 9ed: "Then calls the setTotalCost function." which does not exist.). This is an error in the textbook as the missing variable cannot be initialized. (max 25 points).
Write some driver code which tests this class. Test each member function. Your driver code should create at least 2 instances of this class, load them with valid data, and display the contents of each instance. Do not create the class in a separate file.
If your C++ is stronger, add a string to the private variable portion of the class. The string describes the item. Add appropriate getter/setter methods (accessor/mutator) to utilize the string. Extend the driver program to ask: "How many items in inventory?" (Allow for up to 5 maximum). Loop as many times specified by the user (1 to 5) to load up the inventory with items. You can use an array to store the items, or have 5 variables: item1, item2, ... item5. Provide another loop to display each item, while keeping a subtotal of cost. At the end, output the total cost of the inventory. Test with 3 items. (Although the capacity is up to 5 items, your test output, pasted at the bottom, should demonstrate using at least 3 different inventory items, or "instances" of class Inventory.) Submit just one .cpp file. Do not create the class in a separate file.
If your C++ is really good, add to the previous step: Provide the option to use FileIO (in addition to optional manual keyboard entry) to read and load the inventory items from a datafile of your making. This saves you the trouble of manually entering data; testing will be less work! Echo the data as it is read in for verification. Submit two files: one .cpp file with the class and main; and another data file.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
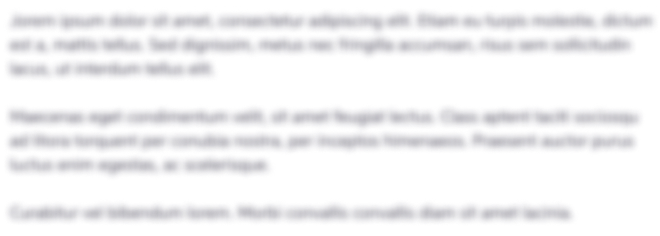
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started