Question
C++ Inventory Management System (using Object Oriented Design and Programming) ) In this assignment, you are required to build a professional inventory management system (using
C++
Inventory Management System (using Object Oriented Design and Programming)
)
In this assignment, you are required to build a professional inventory management system (using object oriented design and programming) with specifications given below. The inventory management system must have products, customers, and orders.
Products must have properties: product id, product name, product price.
Customers must have properties: customer id, customer full name, customer address.
Orders must have properties: order id, order description, product id (id of the product that was
bought as a part of this order), customer id (id of the customer who bought the product).
You must decide about appropriate data types of the properties of products, customers and orders.
Step 1: Create a class diagram. Class diagram must be detailed and have classes with attributes and functions clearly mentioned. Associations should be clearly specified between different classes. Include class diagram in JPG format with your submission.
Step 2: You'll be creating a command-line program that will display following top-banner (displaying Inventory Management System) and then a main-menu with following options to the users.
***************************** *Inventory Management System* *****************************
*Main-menu* Press P to open Products sub-menu Press C to open Customers sub-menu Press O to open Orders sub-menu Press E to exit from the Inventory Management System Please enter your input...
Based on the input of users as mentioned above, a sub-menu should open. If user enters P in the main-menu, it should open Products sub-menu:*Products sub-menu* Press A to add a Product
Press V to view all Products Press D to delete all Products Press B to go back to main-menu Please enter your input...
If user enters C in the main-menu, it should open Customers sub-menu:
*Customers sub-menu* Press A to add a Customer Press V to view all Customers
Press D to delete all Customers Press B to go back to main-menu Please enter your input...
If user enters O in the main-menu, it should open Orders sub-menu:
*Orders sub-menu* Press A to add an Order Press V to view all Orders Press D to delete all Orders Press B to go back to main-menu Please enter your input...
If user enters E in the main-menu, it should exit from the program.
For Products sub-menu: If user presses A, it should the program. If user presses V, it should If user presses D, it should If user presses B, it should
For Customers sub-menu: If user presses A, it should the program. If user presses V, it should If user presses D, it should If user presses B, it should
For Orders sub-menu: If user presses A, it should program. If user presses V, it should If user presses D, it should If user presses B, it should
ask for input of the properties (of a product) and add the new product into
display all the list of products (with all the properties) in the program. delete all the list of products (with all the properties) in the program. show the main-menu to the users and ask for input accordingly.
ask for input of the properties (of a customer) and add the new customer into
display all the list of customers (with all the properties) in the program. delete all the list of customers (with all the properties) in the program. show the main-menu to the users and ask for input accordingly.
ask for input of the properties (of an order) and add the new order into the
display all the list of orders (with all the properties) in the program. delete all the list of orders (with all the properties) in the program. show the main-menu to the users and ask for input accordingly.
Note that the top-banner should be displayed only once in the program.
Important notes:
You must code this assignment using object oriented programming and design as taught in the
lectures and tutorials of this course
Data types of prices must allow decimals too
You must define and implement associations (composition or aggregation) between different
classes as appropriate
Build different functionalities of the program in different functions and use modular approach
Your program must check for any invalid inputs where appropriate (e.g., if user enters an invalid
input)
At the time a new order is being added by users through command line, it should be associated with an existing product and customer record in the program
Your solution must not be limited to a fixed size of items (e.g., number of products, customers or orders etc.)
Think outside the box and be creative
The assignment must be completed using Microsoft Visual Studio 2015 or higher
This is an individual assignment. Each student is required to prepare assignment solution
individually. Please refer to plagiarism policy in the course outline by Carleton University.
Late submission penalty applies as mentioned the course outline.
All the students are expected to learn and develop self-sufficiency in programming by doing
these assignments. Therefore, students should do all the efforts to complete this assignment. In- case of any questions, students may ask the TAs or the instructor for a hint or resolving a particular problem that they may be facing while preparing their solutions.
Submission Submit all source code files from Microsoft Visual Studio project (header files, cpp files) and class diagram file in a single zip file with filename formatted as BIT2400_Assign3_FirstnameLastname.zip,through cuLearn. Do not submit the whole project. Use standard zip utility only. Do not use any other compression formats.
Notes about grading
For all assignments:
Completing the assignment correctly 90%
Good programming practices 10%
If you do all the work required, you get 90%. For producing good and neat code, you get another 10%. This means that your code is indented, commented, readable, and logical, with meaningful variable names. Note that this list is not exhaustive.
This assignment is an extension of the previous assignment. This assignment does not have any extra/bonus component or marks.
Sample output of your program should look like as follows (note, the inputs are examples only and may be different as entered by different users):
***************************** *Inventory Management System* *****************************
*Main-menu* Press P to open Products sub-menu Press C to open Customers sub-menu Press O to open Orders sub-menu Press E to exit from the Inventory Management System Please enter your input...
User entered P
*Products sub-menu* Press A to add a Product Press V to view all Products Press D to delete all Products Press B to go back to main-menu Please enter your input...
User entered V No products to show at the moment.
*Products sub-menu* Press A to add a Product Press V to view all Products Press D to delete all Products Press B to go back to main-menu Please enter your input...
User entered A
Adding a new Product: Enter Product ID: 123 Enter Product Name: Laptop Enter Product Price: 500
A new Product has been added successfully!
*Products sub-menu* Press A to add a Product Press V to view all Products Press D to delete all Products Press B to go back to main-menu Please enter your input...
User entered V Listing following Products:
*Product 1* Product ID: 123 Product Name: Laptop Product Price: 500
*Products sub-menu* Press A to add a Product Press V to view all Products Press D to delete all Products Press B to go back to main-menu Please enter your input...
User entered D All Products deleted successfully!
*Products sub-menu* Press A to add a Product Press V to view all Products Press D to delete all Products Press B to go back to main-menu Please enter your input...
User entered B
*Main-menu* Press P to open Products sub-menu Press C to open Customers sub-menu Press O to open Orders sub-menu Press E to exit from the Inventory Management System Please enter your input...
User entered C
*Customers sub-menu* Press A to add a Customer Press V to view all Customers Press D to delete all Customers Press B to go back to main-menu Please enter your input...
... ... ... ... ... ...
And the program continues based on inputs from user.
User entered O
*Orders sub-menu* Press A to add a Order Press V to view all Order Press D to delete all Order Press B to go back to main-menu Please enter your input...
User entered A
Adding a new Order: Enter Order ID: 968 Enter Order Description: Customer ordering Laptop Enter Product ID: 123 Product ID successfully matched with the products in the program. Enter Customer ID: 456 Customer ID successfully matched with the products in the program.
A new Order has been added successfully!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
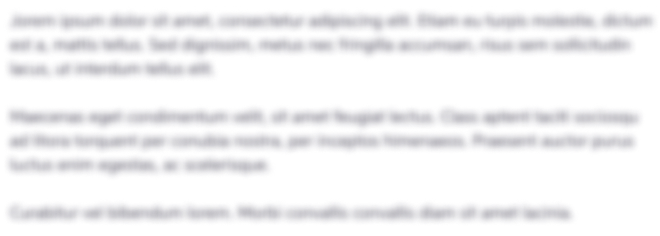
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started