Question
C, like many programming languages, has a library function (rand()) that can be used to generate a ``random'' sequence of numbers (quotes because it's not
C, like many programming languages, has a library function (rand()) that can be used to generate a ``random'' sequence of numbers (quotes because it's not truly random, as mentioned in class). Many languages have a similar function that generates ``random'' numbers in some specified range, useful if for example you're trying to simulate rolling a 6-sided die. C doesn't have such a function, but you can get the same effect using rand() and a little additional code. rand() itself generates a number between 0 and the library-defined constant value RAND_MAX, so to get a value in a smaller range you have to somehow map the larger range to the smaller one. The somewhat obvious way to do this is by computing a remainder (e.g., to map to two possible values, assign even values to 0 and odd values to 1). (I'll call this the ``remainder method''.) But with some implementations of rand() this gives results that aren't very good. The conventional wisdom is therefore to instead try to do a more-direct map (e.g., to map to two possible values, assign values from 0 through RAND_MAX/2 to 0 and the remaining values to 1). (I'll call this the ``quotient method''.)
Your mission for this problem is to complete a C program that, given a number of samples N and a number of ``bins'' B generates a sequence of N ``random'' numbers, uses both methods (remainder and quotient) to map each generated number to a number between 0 and B-1 inclusive, and counts for each method how many elements of the sequence fall into each bin (e.g., for each method bin 0 is how many elements of the sequence map to 0), and prints the result, as in the sample output below. To help you (I hope!) I'm providing a starter program, link below, which you should use as your starting point. Code at the bottom of the program shows how to apply both methods to something returned by rand(). The remainder method is straightforward; the quotient method is less so, but see the footnote1if you're curious.
One other thing to know about rand() is that by default it always starts with the same value (and produces the same sequence). To make it start with a different value, you can call srand() with an integer ``seed'', so your program should prompt for one of those too.
Here is a starter program that prompts for the seed, generates a few ``random'' numbers, and illustrates the two methods of mapping to a specified range:
#include#include /* has rand(), srand(), RAND_MAX */ int main(void) { int seed; printf("seed? "); if (scanf("%d", &seed) != 1) { printf("invalid input "); return 1; } if (seed <= 0) { printf("invalid input "); return 1; } srand(seed); /* FIXME: * replace the rest of this code with your code (but okay to keep * anything you think will help). */ printf("%d ", rand()); printf("%d ", rand()); printf("%d ", rand()); printf("%d ", rand()); puts(""); /* prints a newline */ int n = rand(); int num_bins = 6; printf("next element %d ", n); printf("one way to map to [0..5] %d: %d ", n, n % num_bins); printf("another way map to [0..5] %d: %d ", n, (int) ((((double) num_bins) * n)/(RAND_MAX+1.0))); return 0; }
Hints:
If you find the problem description confusing, maybe an example will clarify a bit: Suppose input specifies 100 samples and 2 bins. The program should generate a sequence of 100 ``random'' numbers. For the remainder method, bin 0 will be how many elements of this sequence are even, while bin 1 will be how many are odd. For the quotient method, bin 0 will be how many elements of this sequence are in the first half of the range from 0 through RAND_MAX, while bin 1 will be how many are in the second half.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
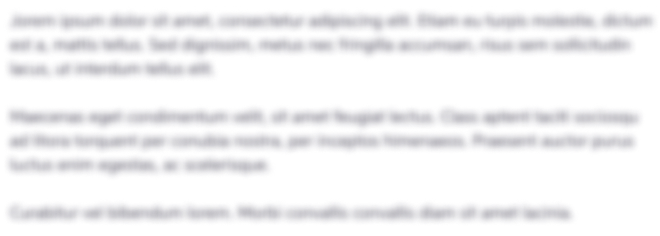
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started