Question
C++ Modify the program of Programming Challenge 1 to allow the user to enter name-score pairs. For each student taking a test, the user types
C++
Modify the program of Programming Challenge 1 to allow the user to enter name-score pairs. For each student taking a test, the user types a string representing the name of the student, followed by an integer representing the student's score. Modify both the sorting and average-calculating functions so they take arrays of structures, with each structure containing the name and score of a single student. In traversing the arrays, use pointers rather than array indices.
IMPORTANT:
Use arrays of objects not structures
Programming Challenge 1:
#include
#include
using namespace std;
//Function prototypes
void arrSelectSort(double *, int);
double arrAvgScore(double *, int);
int main()
{
//Define variables
double *TestScores,
total = 0.0,
average;
int numTest,
count;
//Get the number of test scores you wish to average and put in order
cout << "How many test scores do you wish to enter?";
cin >> numTest;
//Dynamically allocate an array large enough to hold that many scores
TestScores = new double[numTest];
//Get the test scores
cout << "Enter the test scores below. ";
for (count = 0; count < numTest; count++)
{
//Display score
cout << "Test Score " << (count + 1) << ": ";
cin >> TestScores[count];
// Input validation. Only numbers between 0-100
while (TestScores[count]<0 || testscores[count]>99)
{
cout << "You must enter a scores that non-negative" << endl;
cout << "Please enter again: ";
cin >> TestScores[count];
}
}
//Dsiplay the results
arrSelectSort(TestScores, numTest);
average = arrAvgScore(TestScores, numTest);
cout << fixed << showpoint << setprecision(2);
cout << "The test scores, sorted in ascending order, are: ";
for (count = 0; count < numTest; count++)
cout << TestScores[count] << " ";
cout << endl;
cout << "The average of all the test score is " << average << endl;
//Free dynamically allocated memory
delete [] TestScores;
TestScores = 0; //make TestScores point to null
//Display the Test Scores in ascending order
//system ("pause");
return 0;
}
//Ascending order selection sort
void arrSelectSort(double *arr, int size)
{
int startScan;
int minIndex;
double minElem;
for(startScan = 0; startScan < (size - 1); startScan++)
{
minIndex = startScan;
minElem = arr[startScan];
for(int index = startScan; index < size; index++)
{
if (arr[index] < minElem)
{
minElem = arr[index];
minIndex = index;
}
}
if(minIndex!=startScan)
{
double temp = arr[minIndex];
arr[minIndex] = arr[startScan];
arr[startScan] = temp;
}
}
}
double arrAvgScore (double *arr, int size)
{
double total = 0,average;
int numTest;
for (int count = 0; count < size; count++)
{
total += arr[count];
}
average = total / size;
return average;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
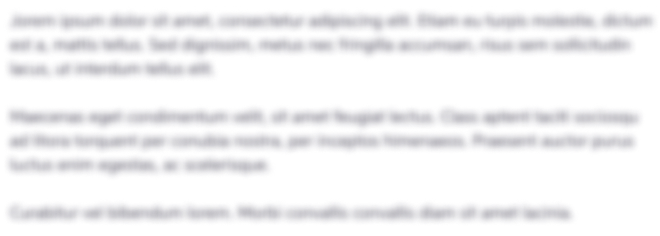
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started