Question
C++ Modify the Student 'class' files as follows: 1) Add a Major field to the Student class definition. Update the student.h and student.cpp files to
C++ Modify the Student 'class' files as follows: 1) Add a Major field to the Student class definition. Update the student.h and student.cpp files to include the additional field. Be sure to include a get and set method for the Major field.
2) Add several majors such as CIS, Math, English, etc. to the students in the array of students located in the main program.
3) When displaying the list of student ID numbers and student names, also display their major. For C++ use the setw( ) function to set the widths of each column so that the columns line up on the screen when displayed. For the C-language, set the parameters for %s in the format string.
4) Change some of the ID numbers and GPAs to illegal values. Have the program detect and display an error message when the illegal values are present.
-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
//student.h
#pragma once #include
class Student { private: string name; int IdNumber; double gpa; public: //constructors Student(); //default constructor Student(string n, int id, double g); //mutattors and accessors void setName(string n); string getName(); void setIdNumber(int id); int getIdNumber(); void setGpa(double g); double getGpa();
};
----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
//student.cpp
//Student.cpp - contains the code for the Student class member methods
#include "Student.h" #include
using namespace std;
//default Student Constructor Student::Student() { name = ""; //set name to an empty string IdNumber = 0; gpa = 0.0; } //Fully qualified Student Constructor Student::Student(string n, int id, double g) { setName(n); //use setName to validate data setIdNumber(id); //use setIdNumber to validate data setGpa(g); //use setGpa to validate data }
//mutators and accessors void Student::setName(string n) { if (isupper(n[0])) //1st character of name must be A-Z name = n; else name = "--Bad name entered"; }
string Student::getName() { return name; }
void Student::setIdNumber(int id) { if (id > 1 && id < 10000) //must be from 0 to 10000 IdNumber = id; else IdNumber = 0; //indicate an illegal selection }
int Student::getIdNumber() { return IdNumber; }
void Student::setGpa(double g) { if (g >= 0.0&&g <= 4.0) gpa = g; else gpa = 0; }
double Student::getGpa() { return gpa;
}
---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
//Student_class.cpp
/* Dat H Do, 0801873 Student_Class.cpp 07/17/17 */
#include
using namespace std;
//Define an array of students Student CIS054[] = { Student("Joe Williams", 44536, 3.4), Student("Sally Washington", 55458, 3.7), Student("Fred MacIntosh", 66587, 2.9), Student("Jose De La Cruz", 67892, 3.5), Student("777 Dan McElroy", 77777, 4.0), Student("Thinh Nguyen", 73657,3.6) };
int main(int argc, char* argv[]) { int NumberOfStudents = sizeof(CIS054) / sizeof(Student); //Display the header line //List all the students in the course
for (int i = 0; i < NumberOfStudents; i++) cout << " " << CIS054[i].getIdNumber() << " " << CIS054[i].getName() << endl; cout << endl; // blank line after displaying the student names
//compute the average gpa of all the students double total = 0; for (int i = 0; i < NumberOfStudents; i++) total += CIS054[i].getGpa(); double average = total / NumberOfStudents; cout << " " << "The average GPA of all the students is " << average << endl << endl; system("pause"); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
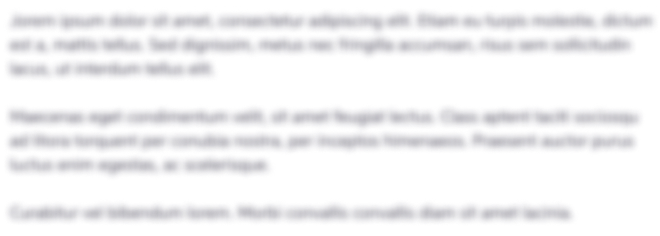
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started