Answered step by step
Verified Expert Solution
Question
1 Approved Answer
C++ Multimap So here's the catch. All the things down below that says linkedlist in C code. Change them to use C++ and a multimap.
C++ Multimap
So here's the catch. All the things down below that says linkedlist in C code. Change them to use C++ and a multimap. No linkedlist required. Just use a multimap. And you can use a class if youwant.
So you are gonna take a title, artist and rating and store in amultimap. Down below are just what are you are going to have to dowith a multimap.
that keeps track of ratings for MP3 files and displaysinformation about them.
Changed Functional Requirements
- Along with the artist and title for each song, also get aninteger from the user that represents the rating for the song. Theorder of input for each song should be artist, title, and rating,all on separate lines.
- You can assume that the artist and title are least 1 characterlong and no longer than 30 (this is different from theMini-Assignment).
- Valid ratings are 1 to 5 inclusive.
- No other input is allowed.
- Put the input into a sorted doubly-linked list (instead of theunsorted singly-linked list from Mini #2), where the sorting isdone by ascending by rating (e.g. 1 comes before 4). If two songshave the same rating, you can arbitrarily choose which song to putfirst.
- The sorting must be done such that each element is insertedinto the proper location in the list in turn.Do not create an unsorted list and thensort the list afterwards (doing this would result in a failing markfor the assignment).
- At the same time, put the song into another separate sortedlinked list, where the sorting is done ascending by artist (e.g.Coldplay comes before U2). If two songs have the same artist, youcan arbitrarily choose which song to put first.
- The user will never enter a song with an artist and title thatis already in the list (e.g. if "Beautiful Day" by "U2" is in thelist already, the user will not ask you to enter it again).
- This means that you will have two separate head pointervariables and two separate tail pointer variables.
- Similar to Mini #2, stop getting user input once an invalidartist of "." is entered by the user.
- You should ignore the song that had the invalid artist (i.e.don't put it into the linked list).
- After the input is complete, traverse the ratings-sorted linkedlist, displaying one song per line with artist (left-justified witha width of 35 characters), title (left-justified with a width of 35characters), and rating (left-justified).
- Next, traverse the artist-sorted linked list, displaying withthe same output format as the previous traversal.
- Next, obtain one song as additional user input in the sameformat as before. Search for that song in the artist-sorted linkedlist.
- If there is a match of artist, title, and rating, prompt for anew rating.
- If the rating is changed, update the artist-sorted linked listwith the new rating. Then delete the song from the rating-sortedlinked list and re-insert it.
- If the rating is unchanged, do nothing.
- The rating will always be a valid rating between 1 and 5inclusive (e.g. I won't test your code by entering a rating of-4).
- If there is not an exact match, simply ignore the change andcontinue with the next step (i.e. just redisplay both linkedlists).
- If there is a match of artist, title, and rating, prompt for anew rating.
- Redisplay both linked lists as before.
- Once completely done, you mustfree all allocated memory.
Additional Functions
- Create the findSong() function. It returns NULLif a song is not found or it returns a pointer to the nodecontaining a song, if both the title and artist are matched (with acase-sensitive match, so you can use strcmp for this). It takesthree parameters:
- songNode *head: head of list
- char *artist: pointer to null-terminated string containingartist
- char *title: pointer to null-terminated string containingtitle
- If only the artist or the title are found but both are notfound in the same node, the song is not found.
- Create the deleteNode() function. It deletesa node, using three parameters:
- songNode *node: node to delete
- songNode **head: pointer to head of list
- songNode **tail: pointer to tail of list
- The key to this function is relinking pointers around the nodebefore deleting.
- If node is NULL, it returns immediately.
- It returns nothing.
Step by Step Solution
★★★★★
3.42 Rating (165 Votes )
There are 3 Steps involved in it
Step: 1
Implement a stack using single linked list concept all the single linked list operations perform bas...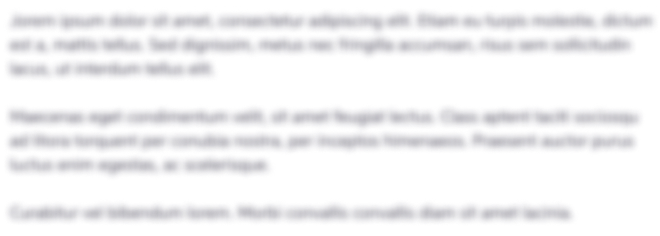
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started