Question
****C++*********** My code is below: In this problem you will change the StringOfCars class so it has an array of pointers to objects, rather than
****C++***********
My code is below:
In this problem you will change the StringOfCars class so it has an array of pointers to objects, rather than an array of objects themselves. This will allow us to have a string of cars that contains Car, FreightCar, and PassengerCar objects all in the same string of cars. This works because a pointer of type Car * can be made to point to Car objects as well as point to the child FreightCar and PassengerCar objects.
Remove the call to the output member function from the three build functions: buildCar, buildFreightCar, and buildPassengerCar.
Because you have pointers of type Car * that may point to any one of the three types of objects, there is a problem. The system does not know what type object will be encountered until execution time. That means a system is needed so the functions that are overridden need to have a mechanism to select the correct version of the function at execution time, rather than having it fixed at compile time. This is done with the virtual declaration. To do this make the declaration of the setKind and the declaration of the ~Car functions virtual in the Car class. This is only done in the declaration, not the definition of the function. This is only done in the parent class, not the children classes.
To change the class StringOfCars, comment out all the code in the member functions of the StringOfCars class and fix them one or two at a time in the following order. These are similar to the previous functions, but changed to allow for the fact that we are putting pointers to cars in the array.
Build the default constructor first. Create a default string of cars in main.
Build an output function, similar to the old one, but dereferrencing the pointers.
Write a push function which adds a car to the string of cars. It takes a Car by constant reference, allocates space in the heap, makes a copy of the Car, and puts the pointer to the Car in the array.
Write a copy constructor similar to the old one, but it gets space for each car and copies each one, as well as getting space for the array.
omit the pop member function.
Add to the build functions a call to push the objects onto the string of cars.
Remove the output from the build functions.
Test the copy constructor by making stringOfCars2 in the stack for main that is a copy of stringOfCars1.
Print stringOfCars2.
..........................................................
#include
#include
#include
#include
#include
using namespace std;
enum Kind {business, maintenance, other, box, tank, flat, otherFreight, chair, sleeper, otherPassenger};
class Car
{
protected:
//all private variables
string reportingMark;
int carNumber;
Kind kind;
string kindInit;
bool loaded;
string destination;
public:
//default constructor
Car()
{
setUp("",0,"other",false,"NONE");
}
//copy constructor
Car(const Car &obj)
{
setUp(obj.reportingMark,obj.carNumber,obj.kindInit,obj.loaded,obj.destination);
}
//third constructor
Car(const string reportingMarkX, const int carNumberX, const string kindX, const bool loadedX, const string destinationX)
{
setUp(reportingMarkX, carNumberX, kindX, loadedX, destinationX);
}
//deconstructor
~Car()
{
}
/* **********setUp*********
* takes in string, int, string, bool, and string
* sets teh private values accoridng to the data input
*
*/
void setUp(string reportingMarkOut, int carNumberOut, string kindInit, bool loadedOut, string destinationOut);
/* **********output**********
* simply outputs the variables in a formatted fashion
*
*/
void output();
virtual void setKind(const string &kindS);
/* **********operator=**********
* overloads the = operator for car objects
*
*/
Car& operator=(const Car & carB);
//friend function
friend bool operator==(const Car&, const Car&);
};
class FreightCar : public Car
{
public:
FreightCar()
{
setUp("",0,"other",false,"NONE");
}
//copy constructor
FreightCar(const FreightCar &obj)
{
setUp(obj.reportingMark,obj.carNumber,obj.kindInit,obj.loaded,obj.destination);
}
FreightCar(const string reportingMarkX, const int carNumberX, const string kindX, const bool loadedX, const string destinationX)
{
setUp(reportingMarkX, carNumberX, kindX, loadedX, destinationX);
}
void setKind(const string &kindS);
};
class PassengerCar : public Car
{
public:
PassengerCar()
{
setUp("",0,"other",false,"NONE");
}
//copy constructor
PassengerCar(const PassengerCar &obj)
{
setUp(obj.reportingMark,obj.carNumber,obj.kindInit,obj.loaded,obj.destination);
}
PassengerCar(const string reportingMarkX, const int carNumberX, const string kindX, const bool loadedX, const string destinationX)
{
setUp(reportingMarkX, carNumberX, kindX, loadedX, destinationX);
}
void setKind(const string &kindS);
};
class StringOfCars
{
private:
const static int ARRAY_MAX_SIZE = 10;
int size;
Car *AOCPointer[ARRAY_MAX_SIZE];
public:
//default constructor
StringOfCars()
{
size = 0;
for(int i = 0; i < ARRAY_MAX_SIZE; i++)
{
//AOCPointer[i] = new Car[ARRAY_MAX_SIZE];
Car *myPointer = new Car;
AOCPointer[i] = myPointer;
}
}
//copy constructor
StringOfCars(const StringOfCars &thing)
{
int counter = thing.size;
Car *myPointer = new Car;
for(counter = 0; counter >= (thing.size - 1); counter++)
{
AOCPointer[counter] = new Car[ARRAY_MAX_SIZE];
push(*thing.AOCPointer[counter]);
}
}
//destructor
~StringOfCars()
{
for(int i = 0; i < size; i++)
{
delete AOCPointer[i];
}
}
void push(const Car &carA);
void pop(Car *carx);
void output();
};
//empty constructors
void input(StringOfCars *myCar);
void buildCar(string reportingMarkOut, int carNumberOut, string kindOut, bool loadedOut, string destinationOut);
void buildFreightCar(string reportingMarkOut, int carNumberOut, string kindOut, bool loadedOut, string destinationOut);
void buildPassengerCar(string reportingMarkOut, int carNumberOut, string kindOut, bool loadedOut, string destinationOut);
const string KIND_ARRAY[10] = {"business", "maintenance", "other", "box", "tank", "flat", "otherFreight", "chair", "sleeper", "otherPassenger"};
//bool isEqual(const Car carA, const Car carB);
/* ****************main******************
*
*/
int main()
{
StringOfCars string1;
input(&string1);
return 0;
}
void input(StringOfCars *myCar)
{
ifstream inputFile;
string type;
string order;
string reportingMark;
string carNumberX;
int carNumber;
string kind;
//Kind kind;
string loadedX;
bool loaded;
string destination = "problem";
inputFile.open("cardata5.txt");
if(inputFile.fail())
{
fprintf(stderr, "Error in reading file ");
exit(0);
}
while(inputFile.peek() != EOF)
{
string templine;
inputFile >> type;
inputFile >> order;
cout << order << endl;
inputFile >> reportingMark;
inputFile >> carNumber;
inputFile >> kind;
inputFile >> loadedX;
if(loadedX == "true")
{
loaded = 1;
}
else
{
loaded = 0;
}
while(inputFile.peek() == ' ')
inputFile.get();
getline(inputFile,destination);
if(type == "Car")
{
buildCar(reportingMark, carNumber, kind, loaded, destination);
}
else if(type == "FreightCar")
{
buildFreightCar(reportingMark, carNumber, kind, loaded, destination);
}
else if(type == "PassengerCar")
{
buildPassengerCar(reportingMark, carNumber, kind, loaded, destination);
}
}
inputFile.close();
}
//************************************************************************
void StringOfCars::push(const Car &carA)
{
Car *point = new Car(carA);
AOCPointer[size] = point;
size++;
}
void StringOfCars::pop(Car *carx)
{
size--;
Car newCar(*AOCPointer[size]);
*carx = newCar;
}
void StringOfCars::output()
{
int printCheck;
for(printCheck = 0; printCheck < size; printCheck++)
{
cout << "This is car number " << (printCheck+1) << endl;
AOCPointer[printCheck]->output();
}
}
//declaired as part of the Car function - see above
void Car::setUp(string reportingMarkOut, int carNumberOut, string kindOut, bool loadedOut, string destinationOut)
{
reportingMark = reportingMarkOut;
carNumber = carNumberOut;
setKind(kindOut);
loaded = loadedOut;
destination = destinationOut;
}
//declaired as part of the Car function - see above
void Car::output()
{
cout << "The Reporting Mark is: " << reportingMark << endl;
cout << "The Car Number is: " << carNumber << endl;
cout << "The Kind is: " << KIND_ARRAY[kind] << endl;
cout << "The Loaded status is: ";
if(loaded == true)
{
cout << "true" << endl;
}
else
{
cout << "false" << endl;
}
cout << "The destination is: " << destination << endl << endl;
}
void Car::setKind(const string &kindS)
{
if(kindS == "business")
{
kind = business;
}
else if(kindS == "maintenance")
{
kind = maintenance;
}
else
{
kind = other;
}
}
void FreightCar::setKind(const string &kindS)
{
if(kindS == "box")
{
kind = box;
}
else if(kindS == "tank")
{
kind = tank;
}
else if(kindS == "flat")
{
kind = flat;
}
else
{
kind = otherFreight;
}
}
void PassengerCar::setKind(const string &kindS)
{
if(kindS == "chair")
{
kind = chair;
}
else if(kindS == "sleeper")
{
kind = sleeper;
}
else
{
kind = otherPassenger;
}
}
/* **********operator==**********
*
* friend function of Car which overloads == to check if two cars are equal only using their reportingMark and their carNumber
*
*/
bool operator==(const Car &a, const Car &b)
{
if((a.reportingMark == b.reportingMark) && (a.carNumber == b.carNumber))
{
return true;
}
else
{
return false;
}
}
/* ********** Car operator= **********
*/
Car & Car::operator=(const Car & carB)
{
setUp(carB.reportingMark, carB.carNumber, carB.kindInit, carB.loaded, carB.destination);
return * this;
}
void buildCar(string reportingMarkOut, int carNumberOut, string kindOut, bool loadedOut, string destinationOut)
{
Car CarThing(reportingMarkOut,carNumberOut, kindOut, loadedOut,destinationOut);
CarThing.output();
}
void buildPassengerCar(string reportingMarkOut, int carNumberOut, string kindOut, bool loadedOut, string destinationOut)
{
PassengerCar passengerCarThing(reportingMarkOut,carNumberOut, kindOut, loadedOut,destinationOut);
passengerCarThing.output();
}
void buildFreightCar(string reportingMarkOut, int carNumberOut, string kindOut, bool loadedOut, string destinationOut)
{
FreightCar FreightCarThing(reportingMarkOut,carNumberOut, kindOut, loadedOut,destinationOut);
FreightCarThing.output();
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
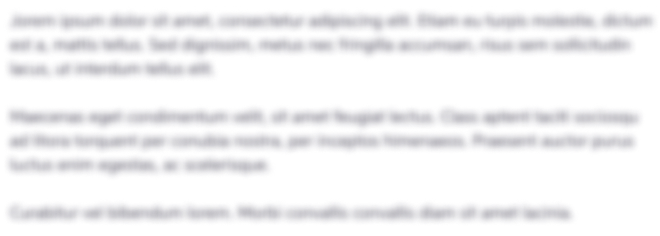
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started