Question
C++ Objectives: Reviewing overloaded relational operators as member and nonmember functions. Overloading relational operators when the left operand is a different type. Instructions: Download Lab14.cpp
C++
Objectives:
- Reviewing overloaded relational operators as member and nonmember functions.
- Overloading relational operators when the left operand is a different type.
Instructions:
Download Lab14.cpp from Canvas. Read and understand the given code. There is a class called Flight and a class called Movie. A class for airline flights and a class for movies are unlikely to inherit from a common base class. However, a movie and a flight both have a duration. One might want to compare the length of a specific movie with the length of a specific flight to determine whether that movie can be played on that flight.
The Movie class must remain unchanged. We can (and are expected to) make changes to the Flight class. Overload the greater than, greater than or equal to, less than, and less than or equal to operators to support comparing the length of a flight with the length of a movie.
- Overload operator>, operator>=, operator< and operator<=.
- Choose between overloading these as member functions or as nonmember functions.
- As indicated by the test code, the class must support comparing Flight objects to Flight objects, Flight objects to Movie objects, and Movie objects to Flight objects. In other words, a Flight object may appear on either or both sides of the operator.
if (flight1 > flight2)
if (flight1 > movie1)
if (movie1 > flight1)
if (flight1 < flight2)
if (flight1 < movie1)
if (movie1 < flight1)
if (flight1 >= flight2)
if (flight1 >= movie1)
if (movie1 >= flight1)
if (flight1 <= flight2)
if (flight1 <= movie1)
if (movie1 <= flight1)
These are all valid comparisons.
- Please dont change the Movie class, and please dont change the if-conditions in main. Write all of your overloaded operator functions in the Flight class. You may, however, update the movie name and change the minutes for further testing.
- When the overloaded operator functions are implemented, these test cases provided in main will work.
main.cpp
#include #include using namespace std;
class Movie { public: Movie(string name = " ", int min = 0) { setData(name, min); }
void setData(string name, int min) { movieName = name; length = min; } void printData() const { cout << "Movie: " << movieName << ", Movie Duration: " << length << " minutes "; }
string getMovieName() const { return movieName; }
int getMinutes() const { return length; }
private: string movieName; int length; };
// TODO: Overload the operators >, >=, <, and <= in the Flight class. // Ensure support for comparing Flight objects to Flight objects, // Flight objects to Movie objects, and Movie objects to Flight objects. // 12 functions in total
class Flight { public:
//only thing that needs to be added to Flight(int flightNum = 0, int min = 0) { setData(flightNum, min); }
void setData(int flightNum, int min) { flightNumber = flightNum; length = min; }
void printData() const { cout << "Flight Number: " << flightNumber << " Flight Duration: " << length << " minutes "; }
int getMinutes() const { return length; }
int getFlightNumber() const { return flightNumber;
}
private: int flightNumber; int length; };
int main() { Flight f1(1298, 101); Flight f2(9821, 125); Movie m1("The Avengers", 101); f1.printData(); f2.printData(); m1.printData(); if (m1 > f1) cout << m1.getMovieName() << " is longer than Flight " << f1.getFlightNumber() << endl; if (m1 < f1) cout << m1.getMovieName() << " is shorter than Flight " << f1.getFlightNumber() << endl; if (m1 < f2) cout << f2.getFlightNumber() << " is shorter than Flight " << m1.getMovieName() << endl; if (m1 > f2) cout << m1.getMovieName() << " is longer than Flight " << f2.getFlightNumber() << endl; if (f1 >= m1) cout << "Flight " << f1.getFlightNumber() << " is longer than or equal to " << m1.getMovieName() << endl; if (f1 <= m1) cout << "Flight "<< f1.getFlightNumber() << " is shorter than or equal to " << m1.getMovieName() << endl; if (f2 <= m1) cout << "Flight " << f2.getFlightNumber() << " is shorter than or equal to " << m1.getMovieName() << endl; if (f2 >= m1) cout << "Flight "<< f2.getFlightNumber() << " is longer than or equal to " << m1.getMovieName() << endl; if (f1 >= f2) cout << "Flight " << f1.getFlightNumber() << " is longer than or equal to Flight " << f2.getFlightNumber() << endl; return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
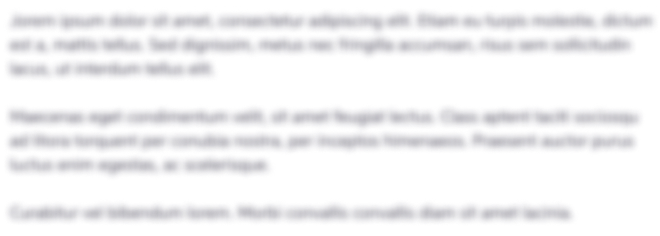
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started