Question
C++ only (please do not use anything more advanced than 2d Arrays while doing this) there is a picture of the prompt at the bottom
C++ only (please do not use anything more advanced than 2d Arrays while doing this)
there is a picture of the prompt at the bottom
In this project, there is a text file, data.txt. The file has students names, and grades for 10 quizzes. Use the function prototypes to write the functions DO NOT CHANGE THE PROTOTYPES. You may add as many functions a you like, but these should work correctly. Read this entire document for instructions.
If you want to try this without any hints, please do so. If you want major hints (really blocks of code) on how to open a file and read the arrays from a file, click on this link.
Tips For This Assignment
For the calculating lowest/highest/average, you should find that to be very similar to the worksheets you have done recently.
WARNING! I WILL CHECK YOUR CODE WITH A DIFFERENT TEXT FILE THAT USES MANY MORE NAMES! This means you must read the file using a while loop, since you do not know how many names are in the file.
Integer Constants
At the top of the file, create these three integer constants:
MAX_STUDENTS set to size 100. Defines the maximum number of students we can process.
MAX_NAME_LEN set to ize 30. Defines the maximum length of a student's name.
NUM_SCORES set to size 10. Holds the number of scores we can expect for each student.
Function prototypes:
-
int readData(ifstream &inFile, int scores[][NUM_SCORES], char names[][MAX_NAME_LEN]);
This functions takes the file stream parameter inFile by reference, after the file is open. It reads from the text file into the names array and the scores array. It essentially populates the array with data from the file and returns an int which is the count of how many students it has read data for from in the file.
4 pts
-
void findLowestEach(int scores[][NUM_SCORES], char names[][MAX_NAME_LEN], int numStudents);
This function takes the 2 parallel arrays and an int numStudents and prints each students names and lowest score on separate lines.
4 pts
-
void findHighestEach(int scores[][NUM_SCORES], char names[][MAX_NAME_LEN], int numStudents);
This function takes the 2 parallel arrays and an int numStudents and prints each students names and highest score on separate lines.
4 pts
-
void calcAverage(int scores[][NUM_SCORES], char names[][MAX_NAME_LEN], int numStudents);
This functions take the 2 parallel arrays and an int numStudents, and calculates the average score of each students and prints the avage. Print the students name and average at the end of this function.
MAKE SURE YOUR RESULTS DON'T ALL HAVE ZEROS AFTER THE DECIMAL POINT!! (Integer Division).
4 pts
-
int main()
Should declare two 2-dim arrays, one for students names, char names[MAX_STUDENTS][MAX_NAME_LEN]; and int scores[MAX_STUDENTS][[NUM_SCORES];
You should also declare a file stream variable inFile and open the file. Then, main should call readData, and load the data from the text file.
Then main should call findLowestEach(.....), and then findHighestEach(.........), and calcAverage(...).
4 pts.
Sample Output (The ... denotes where other lines for other students would appear):
Open Data.txt to view the database:
Lowest Score Summary:
Bob 56
John 34
Joe 45
Amy 54
Nick 64
...
Highest Score Summary:
Bob 94
John 104
Joe 101
...
Average Scores:
Bob 74.8
John 82.6
Joe 80.1
Amy 78.7
Nick 86.2
Alex 89.1
...
PIC OF PROMPT
Step by Step Solution
There are 3 Steps involved in it
Step: 1
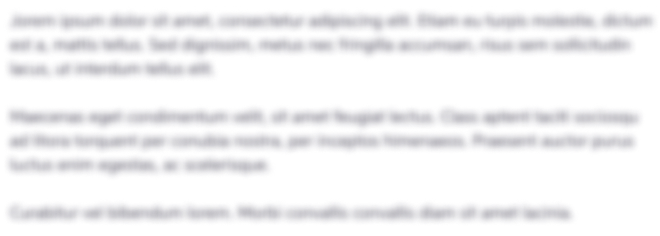
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started