Question
C++ OPTION A (Basic): Complex Numbers A complex number , c , is an ordered pair of real numbers ( doubles ). For example, for
C++
OPTION A (Basic): Complex Numbers
A complex number, c, is an ordered pair of real numbers (doubles). For example, for any two real numbers, s and t, we can form the complex number:
This is only part of what makes a complex number complex. Another important aspect is the definition of special rules for adding, multiplying, dividing, etc. these ordered pairs. Complex numbers are more than simply x-y coordinates because of these operations. Examples of complex numbers in this format are (3, 3), (-1, -5), (1.034, 77.5) and (99.9, -108.5). We will build a class of complex numbers called Complex.
One important property of every complex number, c, is its length, or modulus, defined as follows. If c = (s, t) then:
For example:
Create a class of Complex numbers.
Private Data
double real, double imag - These two doubles define the Complex number and are called therealandimaginary parts. Think of each Complex number as an ordered pair, (real, imag) of doubles.
Public Instance Methods
Constructors - Allow Complex objects to be constructed with zero, one or two double arguments. The zero-parameter constructor initializes the complex number to (0, 0). The one-parameter constructor should set the real member to the argument passed and set the imag member to 0. The two-parameter constructor should set both real and imag according to the parameters. DO ALL THREE VERSIONS IN A SINGLE CONSTRUCTOR USING DEFAULT PARAMETERS.
Accessors/Mutators - The usual -- two mutators, two accessors.
double modulus() - This will return the double |c|, i.e., the modulus, of the complex number. If the Complex object, c, represents the ordered pair (s, t), then the formula above will give the double value to return.
Complex reciprocal() - This defined in the division operator definition, below.
toString() - This will return a string like "(-23.4, 8.9)" to the client. Do not provide a show() or display()method. You will do this using your insertion operator as shown below.
Operators - We will describe several operators that you must implement for the class. Some will be defined externally (which I will call friend operators. However, you may, or may not really need to declare them to be friends - all you need to know is that if I say "friend" I mean implement the operator as a non-member. Otherwise, implement it as a member.
Exception Class -
Division By Zero
Create your own DivByZeroException as a nested class, just like we did when we learned exceptions. Make sure that both your reciprocal() and operator/() functions throw this exception (you can do this by only throwing it for reciprocal() if you do it correctly). Test it out in your client with a try/catch block, attempting both a normal, and a fatal division.
To test for division by zero, look at the reciprocal() and test for that being zero. However, don't test for == 0.0 exactly, because of inaccuracies in computer storage of doubles. Instead, pick a literal like .00000001 and proclaim the a complex object to be zero if its modulus (or modulus squared) is less than that literal value.
Description of the Operators
Operators +, -, * and /
Provide four overloaded operators, +, -, * and /. Implement these operators as friendmethods of the class, so that Complexobjects can be combined using these four operations. Also, allow a mixed mode operation containing a double and a Complex (which would return a Complex), by treating a double x, as if it were the complex number (x,0). This should come about naturally as a result of the Complex/Complex operators and the proper constructor definitions, not by creating 3 overloads for each operator.
The rules for adding complex numbers are:
(r,i) + (s,j) = (r + s, i + j).
Subtraction is defined analogously. Multiplication is defined by the rule:
(r,i) * (s,j) = (r*s - i*j, r*j + s*i).
To define division, first define the operation reciprocal of the complex number c = (r,i) as follows. c.reciprocal() should return the complex number = ( r / (r*r + i*i), -i / (r*r + i*i) ), if (r*r + i*i) is not zero. If (r*r + i*i) is zero, then reciprocal() throws an exception.
Then define division with the help of the reciprocal() function (informally):
(r,i) / (s,j) = (r,i) * reciprocal(s,j)
Notice that if you correspond a normal double number x, with its complex counterpart, (x,0), then you can think of the ordinary double numbers as a subset of the complex numbers. Also, note that, under this correspondence, these four operations result in ordinary addition, multiplication, etc. for the double subset. Try adding or dividing (6,0) and (3,0) if you don't believe me.
In summary, you should be able to handle the combinations below:
Complex a(3,-4), b(1.1, 2.1), c; double x=2, y= -1.7; c = a + b; c = x - a; c = b * y; // and also: c = 8 + a; c = b / 3.2;
To help you confirm your computations, here are some examples:
(1, 2) + (3, 4) = (4, 6) (1, 2) - (3, 4) = (-2, -2) (1, 2) * (3, 4) = (-5, 10) (1, 2) / (3, 4) = (0.44, 0.08) (1, 2) + 10 = (11, 2) 10 / (3, 4) = (1.2, -1.6)
Operators
Overload the insertion and assignment operators in the expected ways.
Operators
a should mean |a| that is, a.modulus() . a == b should mean (a.real == b.real) && (a.imag == b.imag). Define these two operators to make this so. (Note: Pass all Complex parameters as const & and return all values of functions that sensibly return complex numbers (like operator+(), e.g.) asComplex values (not & parameters). OPTION B-1 (Intermediate): Really Fun Complex Project In addition to demonstrating the above functionality, create a ComplexNode and ComplexStack inherited from Node and Stack or our modules or, reading ahead, using the stl stack template. Use it to do something fun, where you get to decide what "fun" means. In your client, be sure to first demonstrate all operators in Option A before demonstrating the stack aspect of your Option B. OUTPUT Something like this: /* --------- output ----------- (1, 2) + (3, 4) = (4, 6) (1, 2) - (3, 4) = (-2, -2) (1, 2) * (3, 4) = (-5, 10) (1, 2) / (3, 4) = (0.44, 0.08) (1, 2) / 0 = div by zero (1, 2) + 10 = (11, 2) 10 / (3, 4) = (1.2, -1.6) How many complex numbers should I generate? 10 pushing (18467, 41) pushing (26500, 6334) pushing (15724, 19169) pushing (29358, 11478) pushing (24464, 26962) pushing (28145, 5705) pushing (16827, 23281) push ing (491, 9961) pushing (11942, 2995) pushing (5436, 4827) 5.83095 vs. 5.83095 equal? 7269.8 vs. 12311.8 (5436, 4827) (15724, 19169) 27246.5 vs. 18467 (26500, 6334) > (18467, 41) Press any key to continue . . . ----------------------------- */
Step by Step Solution
There are 3 Steps involved in it
Step: 1
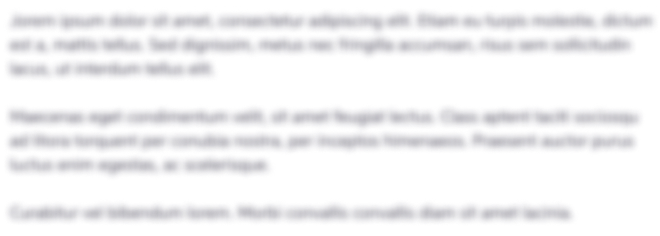
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started