c++ please can you please Implement a Graph class on the template below
Implement a Graph class on the template below and test your class for depth-first search, breadth-first search and in djikstras shortest path. All the public functions for Vertex, Edge and Graph have to be implemented. You can change the private variables and functions as needed. Your program should compile and run against assignment3.cpp provided. You should write your own expanded version of assignment3. 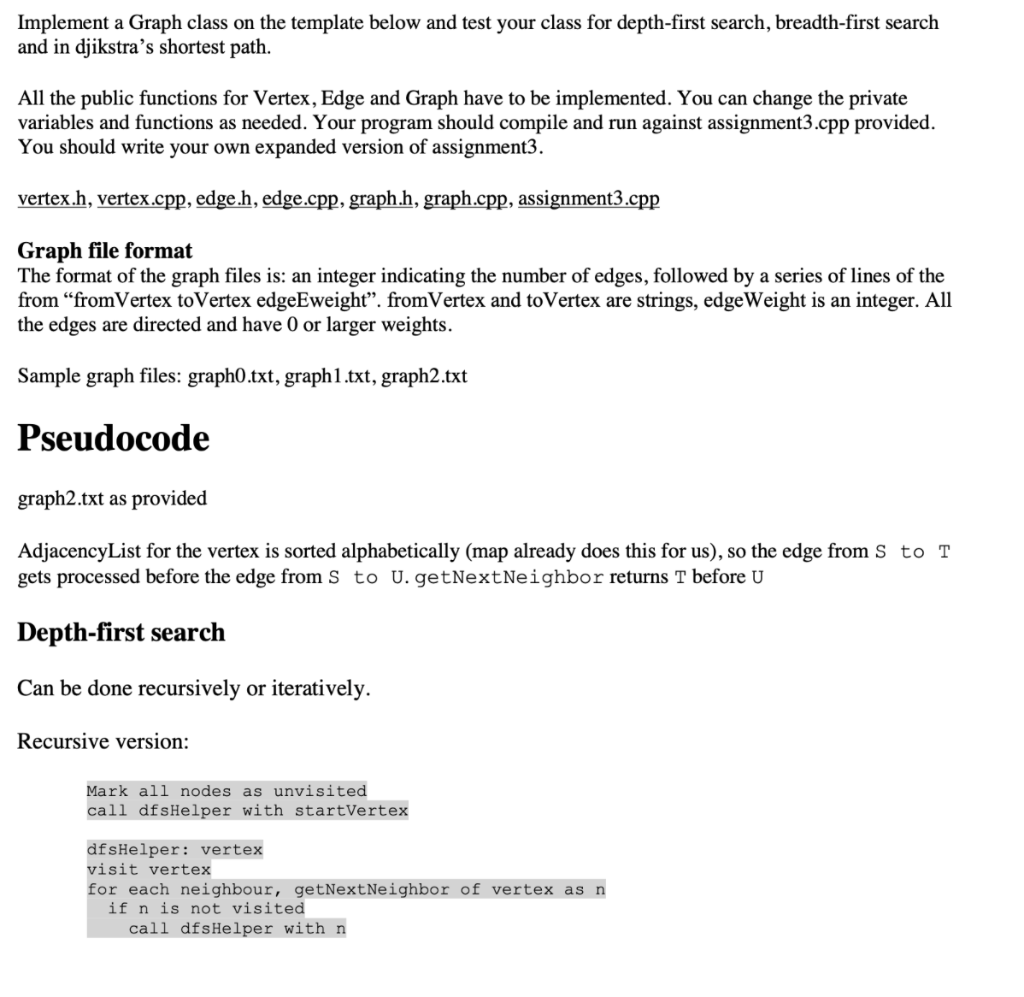
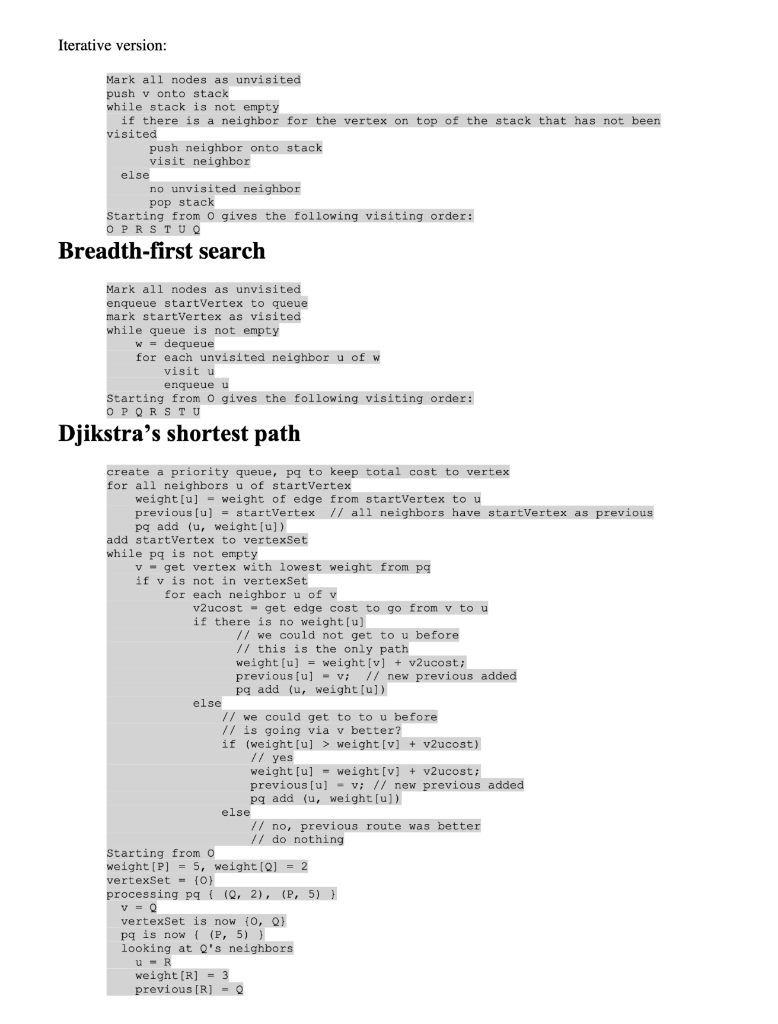
//assignment3.cpp 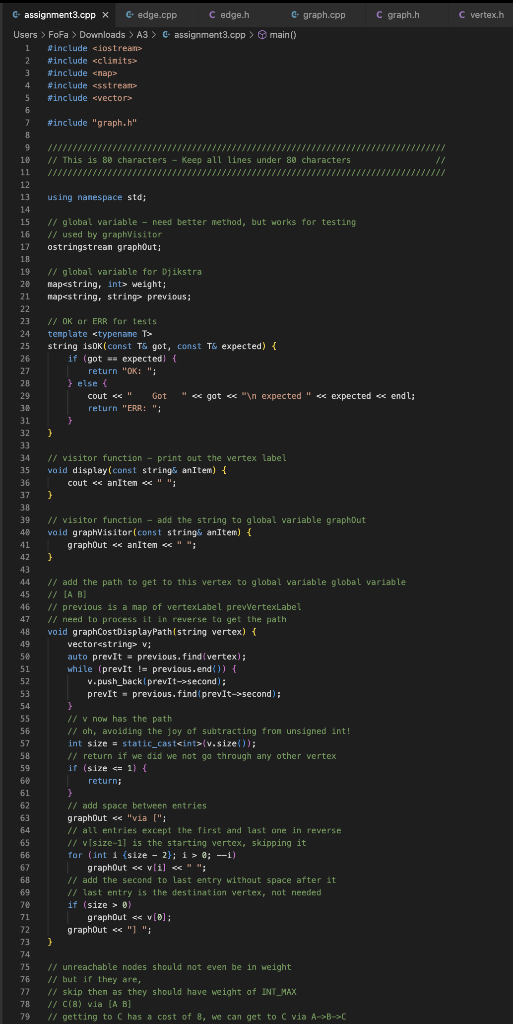
//edge.cpp
//edge.h
//graph.cpp
//graph.h
//graph0.txt
//graph1.txt
//graph2.txt
//vertex.cpp
//vertex.h 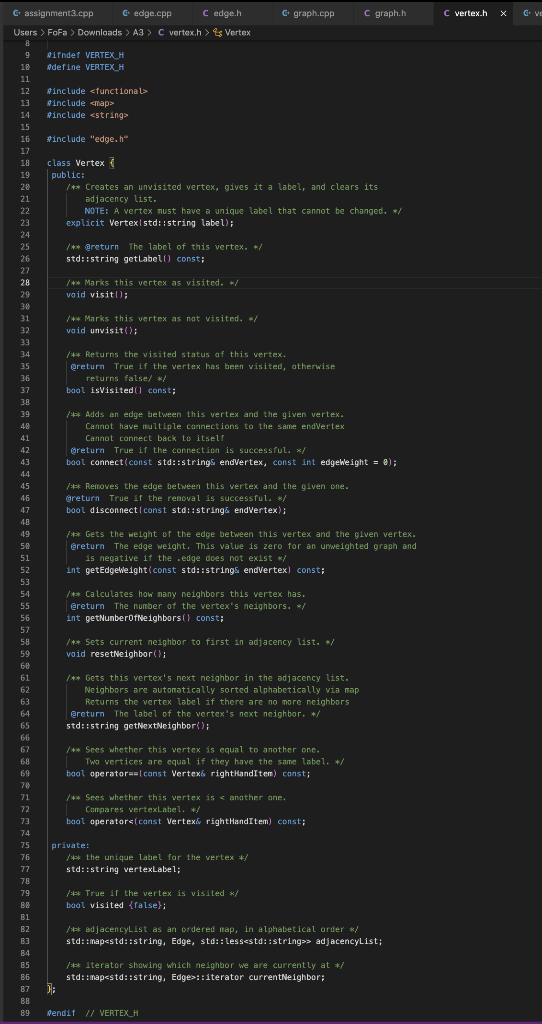
Implement a Graph class on the template below and test your class for depth-first search, breadth-first search and in djikstra's shortest path. All the public functions for Vertex, Edge and Graph have to be implemented. You can change the private variables and functions as needed. Your program should compile and run against assignment3.cpp provided. You should write your own expanded version of assignment 3 . vertex.h, vertex.cpp, edge.h, edge.cpp, graph.h, graph.cpp, assignment 3.cpp Graph file format The format of the graph files is: an integer indicating the number of edges, followed by a series of lines of the from "fromVertex toVertex edgeEweight". fromVertex and toVertex are strings, edgeWeight is an integer. All the edges are directed and have 0 or larger weights. Sample graph files: graph0.txt, graph 1.txt, graph 2.txt Pseudocode graph 2.txt as provided AdjacencyList for the vertex is sorted alphabetically (map already does this for us), so the edge from S to T gets processed before the edge from S to U. getNextNeighbor returns T before U Depth-first search Can be done recursively or iteratively. Recursive version: Mark all nodes as unvisited call dfsHelper with startVertex dfsHelper: vertex visit vertex for each neighbour, getNextNeighbor of vertex as n if n is not visited call dfsHelper with n Iterative version: Mark all nodes as unvisited push v onto stack while stack is not empty if there is a neighbor for the vertex on top of the stack that has not been visited push neighbor onto stack visit neighbor else no unvisited neighbor pop stack Starting from 0 gives the following visiting order: Breadth-first search Mark all nodes as unvisited enqueue startVertex to queue mark startvertex as visited while queue is not empty w= dequeue for each unvisited neighbor u of visit u enqueue u Starting from 0 gives the following visiting order: O P Q R S T U Djikstra's shortest path create a priority queue, pq to keep total cost to vertex for all neighbors u of startvertex weight [u]= weight of edge from startVertex to u previous[u] = startVertex // all neighbors have startvertex as previous pq add (u, weight [u]) add startVertex to vertexset while pq is not empty v = get vertex with lowest weight from pq if v is not in vertexset for each neighbor u of v v2ucost = get edge cost to go from v to u if there is no weight [u] // we could not get to u before // this is the only path weight [u]= weight [v]+v2ucost; previous [u]=v;// new previous added pq add (u, weight [u]) else // we could get to to u before // is going via v better? if (weight [u]> weight [v]+v2ucost) / / yes weight [u]= weight [v]+ v 2 ucost; previous [u]=v;// new previous added pq add (u, weight [u]) else // no, previous route was better // do nothing Starting from 0 vertexset ={0} processing pq ((Q,2),(P,5)) v=Q vertexset is now {0,2} pq is now {(P,5)) looking at Q s neighbors u=R weight [R]=3 previous [R]=0 Submission As always expected when programming, comment clearly and thoroughly. Clearly state any assumptions you make in the beginning comment block of the appropriate place, e.g., the class definition. Comments in the class definition file should describe the ADT, all functionality, and assumptions so someone could use the class and understand behavior and restrictions. Pre and post conditions are fine, but not required. See the example on Assignments page for a well-documented program. You do NOT need to handle data type errors due to bad input. I will run my own main to test your code. The main function provided doesn't test your program fully, so you need to supplement it. Write one function at a time. Test it before moving on to the next function. I suggest starting with add Use valgrind to check for memory leaks as you develop the program. Much easier to fix things early on. Submit a single zip file, assignment 3 . zip with the following files: Class names start with capital letters, but file names are all lowercase for compatibility vertex.h, vertex.cpp, edge.h, edge.cpp, graph.h, graph.cpp assignment 3.cpp your own testing functions and main output.txt - the script file. README. txt (or PDF) - your comments. Includes several bits of information about your implementation, any assignments you made, and any compiler instructions. \#include \#include "edge. h " 1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/ // This is 80 characters - Keep all lines under 80 characters // 1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/ Edge:: Edge( ){} /** constructor with label and weight / Edge: : Edge(const std: : string\& end, int weight) \{\} / return the vertex this edge connects to / std::string Edge: :getEndVertex() const { return "XXX"; \} /** return the weight/cost of travlleing via this edge / int Edge::getWeight () const { return 0; } C+ assignment3.cpp grapho.txt Users > FoFa > Downloads > A3 > grapho 123453AABCBC813 Implement a Graph class on the template below and test your class for depth-first search, breadth-first search and in djikstra's shortest path. All the public functions for Vertex, Edge and Graph have to be implemented. You can change the private variables and functions as needed. Your program should compile and run against assignment3.cpp provided. You should write your own expanded version of assignment 3 . vertex.h, vertex.cpp, edge.h, edge.cpp, graph.h, graph.cpp, assignment 3.cpp Graph file format The format of the graph files is: an integer indicating the number of edges, followed by a series of lines of the from "fromVertex toVertex edgeEweight". fromVertex and toVertex are strings, edgeWeight is an integer. All the edges are directed and have 0 or larger weights. Sample graph files: graph0.txt, graph 1.txt, graph 2.txt Pseudocode graph 2.txt as provided AdjacencyList for the vertex is sorted alphabetically (map already does this for us), so the edge from S to T gets processed before the edge from S to U. getNextNeighbor returns T before U Depth-first search Can be done recursively or iteratively. Recursive version: Mark all nodes as unvisited call dfsHelper with startVertex dfsHelper: vertex visit vertex for each neighbour, getNextNeighbor of vertex as n if n is not visited call dfsHelper with n Iterative version: Mark all nodes as unvisited push v onto stack while stack is not empty if there is a neighbor for the vertex on top of the stack that has not been visited push neighbor onto stack visit neighbor else no unvisited neighbor pop stack Starting from 0 gives the following visiting order: Breadth-first search Mark all nodes as unvisited enqueue startVertex to queue mark startvertex as visited while queue is not empty w= dequeue for each unvisited neighbor u of visit u enqueue u Starting from 0 gives the following visiting order: O P Q R S T U Djikstra's shortest path create a priority queue, pq to keep total cost to vertex for all neighbors u of startvertex weight [u]= weight of edge from startVertex to u previous[u] = startVertex // all neighbors have startvertex as previous pq add (u, weight [u]) add startVertex to vertexset while pq is not empty v = get vertex with lowest weight from pq if v is not in vertexset for each neighbor u of v v2ucost = get edge cost to go from v to u if there is no weight [u] // we could not get to u before // this is the only path weight [u]= weight [v]+v2ucost; previous [u]=v;// new previous added pq add (u, weight [u]) else // we could get to to u before // is going via v better? if (weight [u]> weight [v]+v2ucost) / / yes weight [u]= weight [v]+ v 2 ucost; previous [u]=v;// new previous added pq add (u, weight [u]) else // no, previous route was better // do nothing Starting from 0 vertexset ={0} processing pq ((Q,2),(P,5)) v=Q vertexset is now {0,2} pq is now {(P,5)) looking at Q s neighbors u=R weight [R]=3 previous [R]=0 Submission As always expected when programming, comment clearly and thoroughly. Clearly state any assumptions you make in the beginning comment block of the appropriate place, e.g., the class definition. Comments in the class definition file should describe the ADT, all functionality, and assumptions so someone could use the class and understand behavior and restrictions. Pre and post conditions are fine, but not required. See the example on Assignments page for a well-documented program. You do NOT need to handle data type errors due to bad input. I will run my own main to test your code. The main function provided doesn't test your program fully, so you need to supplement it. Write one function at a time. Test it before moving on to the next function. I suggest starting with add Use valgrind to check for memory leaks as you develop the program. Much easier to fix things early on. Submit a single zip file, assignment 3 . zip with the following files: Class names start with capital letters, but file names are all lowercase for compatibility vertex.h, vertex.cpp, edge.h, edge.cpp, graph.h, graph.cpp assignment 3.cpp your own testing functions and main output.txt - the script file. README. txt (or PDF) - your comments. Includes several bits of information about your implementation, any assignments you made, and any compiler instructions. \#include \#include "edge. h " 1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/ // This is 80 characters - Keep all lines under 80 characters // 1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/1/ Edge:: Edge( ){} /** constructor with label and weight / Edge: : Edge(const std: : string\& end, int weight) \{\} / return the vertex this edge connects to / std::string Edge: :getEndVertex() const { return "XXX"; \} /** return the weight/cost of travlleing via this edge / int Edge::getWeight () const { return 0; } C+ assignment3.cpp grapho.txt Users > FoFa > Downloads > A3 > grapho 123453AABCBC813