Question
C++ Please CS132 PROGRAMMING ASSIGNMENT #3 OVERVIEW; This activity is designed to have you work with strings as a data type, as well as having
C++ Please
CS132 PROGRAMMING ASSIGNMENT #3 OVERVIEW;
This activity is designed to have you work with strings as a data type, as well as having you create a class and read from a data file and use polymorphic data types.
INSTRUCTIONS;
In this program you will be creating a program to play a trivia game. You will be provided with a data file that contains a number of trivia questions, and you will need to create a game that allows the user to pick a number of trivia questions and then determine if they are correct or now. Each question will be stored internally as an object, and it will take control of the ability to determine if the chosen answer is correct or not. It will also have a constructor to parse the input string and break it into its component parts. Your main program will take care of reading the file, creating the storage for all the questions, and asking the user how many questions.
Your basic plan will be to do the following:
Create the base TriviaQuestion class
Create the 5 specialized classes that are subclasses of TriviaQuestion
In the main program get the file
Read one whole line from the data file
o Determine the type of question that it will be from the first three letters.
o Create/New the object with the remainder of the string and allow the data object to create itself.
o Add the new item to the container
Repeat all the above for the entire file
Ask the user how many questions.
Shuffle the container
Starting at 0 to the number of questions, ask the questions.
Get the score
Give them their result.
SAMPLE OUTPUT;
Your final program should look something like this:
Please type in the name of the question bank you would like to open. Questions.txt
How long would you like the quiz to be? 3
This is a multiple choice question worth 2 points, please type in your answer as a value
Question : Peter Mayhew played the part of who in the original Star Wars Trilogy?
1: Chewbacca
2: C-3P0
3: Darth Vader
4: Wicket
--->1
CORRECT
This is a fill in the blank question worth 1 points, please type in your answer as a value
Question : What is the name of Han Solo's ship Millennium _____
--->Eagle
I am sorry, that is incorrect, the correct answer was FALCON.
This is a multiple choice question worth 3 points, please type in your answer as a value
Question : The planet Kashyyyk is home to who?
1: Yoda
2: Chewbacca
3: Ren
4: Maul --->2 CORRECT
Your final score is 5/6 or 83.33% .
----
THE MAIN PROGRAM;
Your main program should do the following:
It should ask the user the name for the file that they want to open up.
It will then attempt to open that file and take care of the strings in the file.
o If the file doesnt exist/cant be found/some other error, the program should state the problem and then quit without crashing.
In the file, each line is a single question with the answers following it.
Your program will then create a storage device to keep track of all the possible questions in some sort of STL container. Note that this needs to be a container of TrivaQuestion objects.
o NOT container of strings, making this container is part of the grade for this assignment.
Once the data file is read and the questions are created it will ask the user how many questions they want to try.
It should then ask the shuffle the container and then ask the first x question from the container based upon their prior answer.
o Note that the main program only starts the question, the question class will take care of itself.
The main program should record the number of points they got right and determine the final score
THE TRIVAQUESTION CLASS;
For this assignment, you will create a base question class that handles all the actions for an individual question. But it will also be polymorphic for each type of question. The methods that you will need are the following
TRIVAQUESTION();
You will want a default constructor that doesnt do anything, but does allow the object to be created. INT GETMAX() This method is a simple method that should return the number of points that a question is worth.
INT ASKQUESTION() = 0;
This is the method that will print out the question, any possible answers, get the user input, and respond if the answer is correct or not. It should then return either zero if they got it wrong, or the max points possible if they get it correct. This method is entirely self-contained. It should ask the question and wait for the result. The main program will not deal with the question at all.
VALUES;
Your program may have need of various other attributes/fields. But most of the time I would assume that you would have at least the following fields
Int points
String question
THE OTHER CLASSES;
The other classes you will need will be:
5 Subclasses of TriviaQuestion
o A Fill in the Blank question
o A 3 part multiple choice question
o A 4 part multiple choice question
o A True/False question
o A numeric type question.
THE DATA;
Each line of the data file except for the first is a single line that looks something like the following: FIB 1 What is the name of Han Solo's ship Millennium _____ : Falcon. Every line of the data file starts with a 3 letter (all caps) symbol that tells what kind of question you will have, then it has a number for the point value of the question. These two symbols are followed by the question itself with a colon : at the end. The symbols after the : depend on the type of question.
FIB Fill in the blank
o There is one symbol after the : which is the correct answer
MC3 Multiple choice with 3 possible answers
o There are 3 additional strings separated by colons. The correct answer is always first, followed by two incorrect answers.
o Make sure to shuffle these before printing them. You dont want the correct answer to always be A MC4 Multiple choice with 4 possible answers
o There are 4 additional strings separated by colons. The correct answer is always first, followed by three incorrect answers.
o Make sure to shuffle these before printing them. You dont want the correct answer to always be A
NUM Numeric with an number answer
o The correct answer as a number .
TOF True or False question
o There is one symbol after the : which is the correct answer True or False
----
Questions.txt:
MC3 1 Which actress starred as Queen Amidala's decoy and hand-maiden Sab? : Kiera Knightley : Natalie Portman : Daisy Ridley FIB 3 What planet does Obi-Wan Kenobi travel to where he discovers an army of clones created for the Republic? _____ : Kamino MC3 2 Who is the Sith leader of the Separatist movement? : Count Dooku : General Grevious : Palpatine MC3 1 What does the Emperor say is Luke's weakness? : His faith in his friends : His youth : His lack of foresight NUM 2 How many arms does the alien diner owner, Dex Jettster, have? : 4 MC4 1 What do Han and Luke ride on in the snow on Hoth? : Tauntaun : Dewback : Wookie : Kyrat FIB 1 Who is Jango Fett's son? ____ Fett : Boba TOF 1 Anakin's cuts off Count Dooku right hand? : True MC4 1 What is Chancellor Palpatine's malicious Sith alter-ego? : Darth Sidious :Darth Maul : Darth Plagus : Darth Vader NUM 2 In what detention block was Princess Leia being held in, in the first Death Star? AA-__ : 23 MC3 1 What does TIE stand for in TIE Fighter? : Twin Ion Engines : Twin Ionic Emitters : Two Interior Exahaust FIB 3 Who is the senator that adopts Leia, and what planet is he from? Senator Bail Organa, from _______ : Alderaan MC3 2 "You were right," were whose last words? : Darth Vader : Darth Sidious : Han Solo NUM 1 What is the name of the order Darth Sidious gives the clone troopers, which means they will kill all Jedi? Order __ : 66 NUM 2 How many people does Darth Vader kill in The Empire Strikes Back? : 2 FIB 3 What's the name of the planet where Anakin Skywalker loses the duel against Obi-Wan, which leaves him mangled and burned? ________ : Mustafar MC4 1 What do they call the children being trained as Jedi? : Younglings : Earlies : Childern : Force Wannabe's MC3 2 Who was the composer for every film in the Star Wars Saga? : John Williams : Michael Giacchino : Ludwig Gransson MC4 1 What are the short junk-collecting aliens on Tatooine called? : Jawas : Ewoks : Yoda's : Wookiees FIB 3 What are Luke's aunt and uncle last name? Owen and Beru ____ : Lars NUM 1 How many meters big is the exhaust port that is the target for Rebel Alliance Starfighters in A New Hope? : 2 NUM 2 Which planet holds the hidden rebel base? Yavin __ : 4 FIB 2 What planet does the Rebel Alliance find refuge on at the beginning of The Empire Strikes Back? ____ : Hoth MC4 1 Who is Han's friend who runs the cloud city of Bespin? : Lando Calrissian : Chewbacca : Nunb Nunb : Lobot MC4 1 In what substance is Han Solo frozen for delivery to Jabba the Hutt? : Carbonite : Water : Carbon : Gold MC4 2 What race of alien is Admiral Ackbar? : Mon Calamari : Fish Stick : Rodian : Mirialan NUM 2 Which year was the film Return of the Jedi released? : 1983 NUM 3 How old is Yoda when he dies? : 900 MC4 1 Who infiltrates Jabba's palace in disguise to save Han Solo? : Princess Leia : Luke : Chewbacca : R2-D2 MC3 1 What are the miniature aliens that help the Rebels destroy the shield generator on Endor? : Ewoks : Whills : Jawas MC4 3 What is the name of Lando Calrissian's co-pilot in Return of the Jedi? : Nien Nunb : Nunb Nunb : Lobot : Wedge MC4 1 George Lucas' original last name for Luke was what? : Starkiller : Starfinder : Skyguy : Skyseeker NUM 1 Han Solo's trusty blaster is what type of blaster? DL-__ : 44 MC4 3 What planet does Rey live on in The Force Awakens? : Jakku : Tatooine : Endor : Korriban FIB 2 What is Poe Dameron's droid called? ____ : BB-8 NUM 3 How old was Padm when she became Queen? : 14
Step by Step Solution
There are 3 Steps involved in it
Step: 1
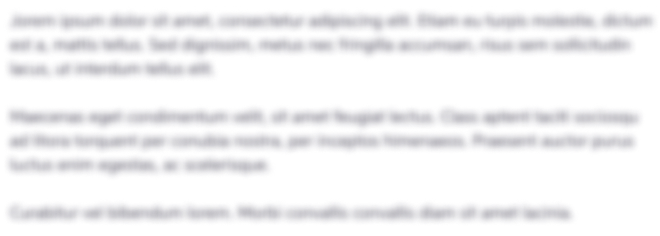
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started