Question
C++ PLease edit this below main.cpp to the requirement // Assignment #2 - Sparse Matrices (Part A) // // ------------------------- CLIENT FILE ---------------------- #include using
C++
PLease edit this below main.cpp to the requirement
// Assignment #2 - Sparse Matrices (Part A) // // ------------------------- CLIENT FILE ----------------------
#include using namespace std; #include "FHsparseMat.h"
#define MAT_SIZE 100000 typedef SparseMat SpMat;
// --------------- main --------------- int main() { int row=0, col=0; SpMat mat(MAT_SIZE, MAT_SIZE, 0); // 100000 x 100000 filled with 0 cin >> row >> col; // test mutators mat.set(row, col, 77); mat.set(2, 5, 10); mat.set(2, 5, 35); // should overwrite the 10 mat.set(3, 9, 21); mat.set(MAT_SIZE, 1, 5); // should fail silently mat.set(9, 9, mat.get(3, 9)); // should copy the 21 here mat.set(4,4, -9); mat.set(4,4, 0); // should remove the -9 node entirely mat.set(MAT_SIZE-1, MAT_SIZE-1, 99);
// test accessors and exceptions try { cout
// show top left 15x15 mat.showSubSquare(0, 15);
// show bottom right 15x15 mat.showSubSquare(MAT_SIZE - 15, 15); // code provided by student // Test the first part above, make sure it works as you expected // then uncomment the following line to enable the second part #define SECOND_PART #ifdef SECOND_PART // additional test for option A cout
// test optoin A mutators cout
// show top left 12x12 cout
cout
// show bottom right 12x12 cout 13.1 Sparse Matrices (Part A) Part A is required. Part B is optional but is worth 2 points extra credit (must be submitted in addition to Part A). Make sure you have read and understood both modules A and B of this week, before submitting this assignment. Part A (required) - Sparse Matrices This is going to be a fun assignment. Imagine trying to allocate a 100000 x 100000 matrix of objects on any computer in a resident program (without using disk storage). Can't be done. But with your knowledge of vectors and lists, you can create a template that you and your company can use to do just that. Design a class template SparseMat which implements a sparse matrix. Use FHvector and FHlist, only, as base ADTs on which to build your class. Your primary public instance methods will be: SparseMat( int r, int c, const Object & defaultVal) - A constructor that establishes a size (row size and column size both > 1) as well as a default value for all elements. const Object & get(int r, int c) const - An accessor that returns the object stored in row r and column c. It throws a user-defined exception if matrix bounds (row and/or column) are violated. bool set(int r, intc, const Object &x) - A mutator that places x in row r and column c. It returns false without an exception if bounds are violated. Also, if x is the default value it will remove any existing node (the internal data type used by SparseMat) from the data structure, since there is never a need to store the default value explicitly. Of course, if there is no node present in the internal data representation, set() will add one if x is not default and store x in it. void clear() - clears all the rows, effectively setting all values to the defaultVal (but leaves the matrix size unchanged) void showSubSquare(int start, int showsize) - a display method that will show a square sub-matrix anchored at (start, start) and whose size is showsize x showsize. In other words it will show the rows from start to start + showsize -1 and the columns from start to start + show_size - 1. This is mostly for debugging purposes since we obviously cannot see the entire matrix at once. Note: you need to take care of the out boundary conditions and display only the contents within the matrix. Here is a sample main() that will test your template. However, this does not prove that you are correctly storing, adding and removing internal nodes as needed. You'll have to confirm that by stepping through your program carefully. You main should also print the upper left and lower right of the huge) matrix, so we can peek into it. #include
Step by Step Solution
There are 3 Steps involved in it
Step: 1
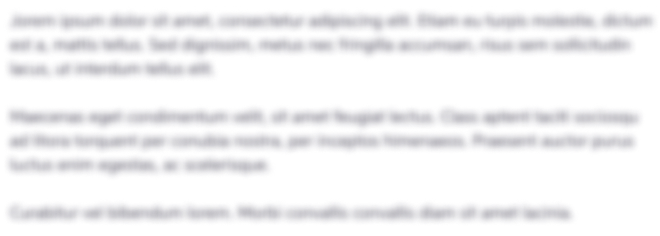
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started