Question
C++ Please help me with the GroceryInventory class #pragma once #include using namespace std; class GroceryItem { private: string _name; int _quantity; float _unitPrice; bool
C++
Please help me with the GroceryInventory class
#pragma once
#include
using namespace std;
class GroceryItem {
private:
string _name;
int _quantity;
float _unitPrice;
bool _taxable;
public:
GroceryItem();
GroceryItem(const string&, const int&, const float&, const bool&);
string getName() const;
void setName(const string&);
int getQuantity() const;
void setQuantity(const int&);
float getUnitPrice() const;
void setUnitPrice(const float&);
bool isTaxable() const;
void setTaxable(const bool&);
};
// Default Constructor
GroceryItem::GroceryItem() {
this->_name = "";
this->_quantity = 0;
this->_unitPrice = 0;
this->_taxable = false;
}
// Parameter Constructor
GroceryItem::GroceryItem(const string &name, const int &quantity, const float &unitPrice, const bool &taxable)
{
this->_name = name;
this->_quantity = quantity;
this->_unitPrice = unitPrice;
this->_taxable = taxable;
}
// setter functions
void GroceryItem::setName(const string &name) {
this->_name = name;
}
void GroceryItem::setQuantity(const int &quantity) {
this->_quantity = quantity;
}
void GroceryItem::setUnitPrice(const float &unitprice) {
this->_unitPrice = unitprice;
}
void GroceryItem::setTaxable(const bool &taxable) {
this->_taxable = taxable;
}
// getter functions
string GroceryItem::getName() const {
return _name;
}
int GroceryItem::getQuantity() const {
return _quantity;
}
float GroceryItem::getUnitPrice() const {
return _unitPrice;
}
bool GroceryItem::isTaxable() const {
return _taxable;
}
// GrocerInventory
#pragma once
#include
#include
#include
#include
#include "GroceryItem.h"
using namespace std;
class GroceryInventory {
private:
vector _inventory;
float _taxRate;
public:
GroceryInventory();
GroceryItem& getEntry(const string&);
void addEntry(const string&, const int&, const float&, const bool&);
float getTaxRate() const;
void setTaxRate(const float&);
void createListFromFile(const string&);
float calculateUnitRevenue() const;
float calculateTaxRevenue() const;
float calculateTotalRevenue() const;
GroceryItem& operator[](const int&);
};
//Total Revenue
float GroceryInventory::calculateTotalRevenue() const {
}
//Tax Revenue
float GroceryInventory::calculateTaxRevenue() const {
}
//Unit Revenue
float GroceryInventory::calculateUnitRevenue() const {
}
//assignment operator
GroceryItem& GroceryInventory::operator[](const int &other) {
}
GroceryItem& GroceryInventory::getEntry(const string &Name)
{
}
GroceryInventory::GroceryInventory() {
}
void GroceryInventory::addEntry(const string &name, const int &quantity, const float &price, const bool &taxable) {
_inventory.push_back(GroceryItem(name, quantity, price, taxable));
}
float GroceryInventory::getTaxRate() const {
return _taxRate;
}
void GroceryInventory::setTaxRate(const float& taxrate) {
this->_taxRate = taxrate;
}
void GroceryInventory::createListFromFile(const string& filename) {
ifstream input_file(filename);
if (input_file.is_open()) {
cout << "Successfully opened file " << filename << endl;
string name;
int quantity;
float unit_price;
bool taxable;
while (input_file >> name >> quantity >> unit_price >> taxable) {
addEntry(name, quantity, unit_price, taxable);
}
input_file.close();
}
else {
throw invalid_argument("Could not open file " + filename);
}
}
Here is the main function to test
#include
#include "GroceryItem.h"
#include "GroceryInventory.h"
using namespace std;
template
bool testAnswer(const string&, const T&, const T&);
template
bool testAnswerEpsilon(const string&, const T&, const T&);
///////////////////////////////////////////////////////////////
// DO NOT EDIT THIS FILE (except for your own testing) //
// CODE WILL BE GRADED USING A MAIN FUNCTION SIMILAR TO THIS //
///////////////////////////////////////////////////////////////
int main() {
// test only the GroceryItem class
GroceryItem item("Apples", 1000, 1.29, true);
testAnswer("GroceryItem.getName() test", item.getName(), string("Apples"));
testAnswer("GroceryItem.getQuantity() test", item.getQuantity(), 1000);
testAnswerEpsilon("GroceryItem.getPrice test", item.getUnitPrice(), 1.29f);
testAnswer("GroceryItem.isTaxable test", item.isTaxable(), true);
// test only the GroceryInventory class
GroceryInventory inventory;
inventory.addEntry("Apples", 1000, 1.99, false);
inventory.addEntry("Bananas", 2000, 0.99, false);
testAnswerEpsilon("GroceryInventory.getEntry() 1", inventory.getEntry("Apples").getUnitPrice(), 1.99f);
testAnswerEpsilon("GroceryInventory.getEntry() 2", inventory.getEntry("Bananas").getUnitPrice(), 0.99f);
string i;
cout << "Passed";
cin >> i;
return 0;
// test copy constructor
GroceryInventory inventory2 = inventory;
testAnswer("GroceryInventory copy constructor 1", inventory2.getEntry("Apples").getUnitPrice(), 1.99f);
inventory.addEntry("Milk", 3000, 3.49, false);
inventory2.addEntry("Eggs", 4000, 4.99, false);
// Expect the following to fail
string nameOfTest = "GroceryInventory copy constructor 2";
try {
testAnswerEpsilon(nameOfTest, inventory.getEntry("Eggs").getUnitPrice(), 3.49f);
cout << "FAILED " << nameOfTest << ": expected to recieve an error but didn't" << endl;
}
catch (const exception& e) {
cout << "PASSED " << nameOfTest << ": expected and received error " << e.what() << endl;
}
// test assignment operator
GroceryInventory inventory3;
inventory3 = inventory;
testAnswerEpsilon("GroceryInventory assignment operator 1", inventory3.getEntry("Apples").getUnitPrice(), 1.99f);
inventory.addEntry("Orange Juice", 4500, 6.49, false);
inventory3.addEntry("Diapers", 5000, 19.99, false);
// Expect the following to fail
nameOfTest = "GroceryInventory assignment operator 2";
try {
testAnswerEpsilon(nameOfTest, inventory.getEntry("Diapers").getUnitPrice(), 19.99f);
cout << "FAILED " << nameOfTest << ": expected to recieve an error but didn't" << endl;
}
catch (const exception& e) {
cout << "PASSED " << nameOfTest << ": expected and received error " << e.what() << endl;
}
// test the GroceryInventory class
GroceryInventory inventory4;
inventory4.createListFromFile("shipment.txt");
inventory4.setTaxRate(7.75);
testAnswer("GroceryInventory initialization", inventory4.getEntry("Lettuce_iceberg").getQuantity(), 9541);
testAnswerEpsilon("GroceryInventory.calculateUnitRevenue()", inventory4.calculateUnitRevenue(), 1835852.375f);
testAnswerEpsilon("GroceryInventory.calculateTaxRevenue()", inventory4.calculateTaxRevenue(), 11549.519531f);
testAnswerEpsilon("GroceryInventory.calculateTotalRevenue()", inventory4.calculateTotalRevenue(), 1847401.875f);
return 0;
}
template
bool testAnswer(const string& nameOfTest, const T& received, const T& expected) {
if (received == expected) {
cout << "PASSED " << nameOfTest << ": expected and received " << received << endl;
return true;
}
cout << "FAILED " << nameOfTest << ": expected " << expected << " but received " << received << endl;
return false;
}
template
bool testAnswerEpsilon(const string& nameOfTest, const T& received, const T& expected) {
const double epsilon = 0.0001;
if ((received - expected < epsilon) && (expected - received < epsilon)) {
cout << fixed << "PASSED " << nameOfTest << ": expected and received " << received << endl;
return true;
}
cout << "FAILED " << nameOfTest << ": expected " << expected << " but received " << received << endl;
return false;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
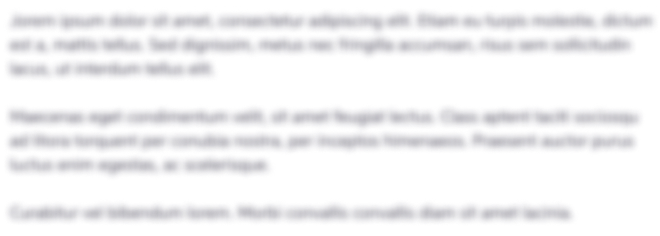
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started