Question
C++ PLEASE HELP!!! Please implement the recursive functions (except #3 and 7) with the function prototypes: //Function prototypes int binarySearch(const int anArray[], int first, int
C++ PLEASE HELP!!!
Please implement the recursive functions (except #3 and 7) with the function prototypes:
//Function prototypes
int binarySearch(const int anArray[], int first, int last, int target);
int fact(int n);
int iterativeRabbit(int n);
int rabbit(int n);
void writeArrayBackward(const char anArray2[], int first, int last);
void writeBackward(string s);
void writeBackwardIterative(string s);
int sumUpTo(int n);
int binarySearch(const int anArray[], int first, int last, int target)
/** Searches the array anArray[first] through anArray[last]
for a given value by using a binary search.
@pre 0 <= first, last <= SIZE - 1, where SIZE is the
maximum size of the array, and anArray[first] <=
anArray[first + 1] <= ... <= anArray[last].
@post anArray is unchanged and either anArray[index] contains
the given value or index == -1.
@param anArray The array to search.
@param first The low index to start searching from.
@param last The high index to stop searching at.
@param target The search key.
@return Either index, such that anArray[index] == target, or -1.
*/
int fact(int n)
/** Computes the factorial of the nonnegative integer n.
@pre n must be greater than or equal to 0.
@post None.
@return The factorial of n; n is unchanged. */
int iterativeRabbit(int n)
/** Iterative solution to the rabbit problem. */
int rabbit(int n)
/** Computes a term in the Fibonacci sequence.
@pre n is a positive integer.
@post None.
@param n The given integer.
@return The nth Fibonacci number. */
void writeArrayBackward(const char anArray2[], int first, int last)
/** Writes the characters in an array backward.
@pre The array anArray contains size characters, where size >= 0.
@post None.
@param anArray The array to write backward.
@param first The index of the first character in the array.
@param last The index of the last character in the array. */
void writeBackward(string s)
/** Writes a character string backward.
@pre The string s to write backward.
@post None.
@param s The string to write backward. */
void writeBackwardIterative(string s)
/** Iterative version. */
int sumUpTo(int n)
/** Computes the sum of the integers from 1 through n.
@pre n > 0.
@post None.
@param n A positive integer.
@return The sum 1 + 2 + . . . + n. */
Test your functions with followings:
int anArray[SIZE] = {1, 5, 9, 12, 15, 21, 29, 31};
char anArray2[3] ={'c','a','t'};
char A, B, C;
string s = "cat";
SAMPLE RUN
1. Binary search with anArray
Search for 9 return 2
Search for 6 returns - 1
2. fact(3) reutrns 6
3. iterativeRabbit(6) returns 8
4. rabbit(6) returns 8
5. writeArrayBackward(anArray2, 0, 2) returns tac
6. writeBackward(s) returns tac
7. writeBackwardIterative(s) returns tac
8. sumUpTo<10> returns 55
Step by Step Solution
There are 3 Steps involved in it
Step: 1
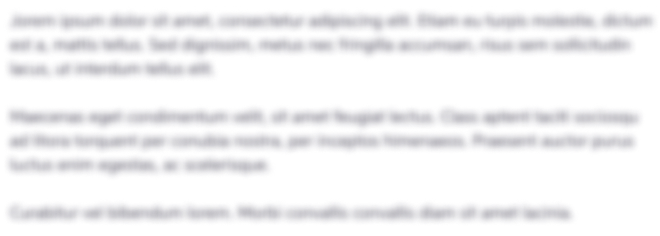
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started