Question
C++ Please only answer of you're fluent in c++, you are to complete the methods as specified in the minpriorityqueu.h, and it must pass all
C++ Please only answer of you're fluent in c++, you are to complete the methods as specified in the minpriorityqueu.h, and it must pass all the tests in the main.cpp file. This is essentially a min heap. An implementation of priority queues using heaps, that is a simple data structure that looks tree-based, but is actually array-based.
//////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
//minpriorityqueue.h
#ifndef MINPRIORITYQUEUE_H #define MINPRIORITYQUEUE_H // NOTE: You may not include any other libraries! #include #include #include // Has pair and swap using namespace std; template class MinPriorityQueue { // For the mandatory running times below: // // n is the number of elements in the MinPriorityQueue. // // Assume that the operations of unordered_map are O(1) time // (they are average case, but not worst-case). public: // Creates an empty MinPriorityQueue MinPriorityQueue() { // TODO } // Returns the number of elements in the MinPriorityQueue. // // Must run in O(1) time. int size() { // TODO } // Pushes a new value x with priority p // into the MinPriorityQueue. // // Must run in O(log(n)) time. void push(T x, int p) { // TODO } // Returns the value at the front of the MinPriorityQueue. // Undefined behavior if the MinPriorityQueue is empty. // // Must run in O(1) time. T front() { // TODO } // Removes the value at the front of the MinPriorityQueue. // Undefined behavior if the MinPriorityQueue is empty. // // Must run in O(log(n)) time. void pop() { // TODO } // If x is in the MinPriorityQueue // with current priority at least new_p, // then changes the priority of x to new_p. // Undefined behavior otherwise. // // Must run in O(log(n)) time. void decrease_key(T x, int new_p) { // TODO } private: // You don't need any other instance variables, // but you can add some if you want to. vector H; // The heap. unordered_map I; // Maps values to their indices in H. }; #endif
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
//main.cpp
#include #include #include #include "minpriorityqueue.h" using namespace std; inline void _test(const char* expression, const char* file, int line) { cerr 90000; --l) { string s = Q4.front(); int p = priority(s); test(Q4.size() == l); Q4.pop(); test(p >= prev_p); prev_p = p; } // Test decrease_key() in a very simple case MinPriorityQueue Q5; Q5.push("hare", 5); Q5.push("tortoise", 10); test(Q5.front() == "hare"); test(Q5.size() == 2); Q5.decrease_key("tortoise", 3); test(Q5.front() == "tortoise"); test(Q5.size() == 2); Q5.pop(); test(Q5.front() == "hare"); test(Q5.size() == 1); // Test decrease_key() in more complex cases MinPriorityQueue Q6; Q6.push("e", 50); Q6.push("d", 40); Q6.push("c", 30); Q6.push("b", 20); Q6.push("a", 10); // [(e, 50), (d, 40), (c, 30), (b, 20), (a, 10)] test(Q6.front() == "a"); test(Q6.size() == 5); Q6.decrease_key("e", 35); // [(d, 40), (e, 35), (c, 30), (b, 20), (a, 10)] Q6.decrease_key("c", 15); // [(d, 40), (e, 35), (b, 20), (c, 15), (a, 10)] Q6.decrease_key("d", 8); // [(e, 35), (b, 20), (c, 15), (a, 10), (d, 8)] Q6.decrease_key("b", 5); // [(e, 35), (c, 15), (a, 10), (d, 8), (b, 5)] test(Q6.front() == "b"); test(Q6.size() == 5); // [(e, 35), (c, 15), (a, 10), (d, 8)] Q6.pop(); test(Q6.front() == "d"); test(Q6.size() == 4); // [(e, 35), (c, 15), (a, 10)] Q6.pop(); test(Q6.front() == "a"); test(Q6.size() == 3); // [(e, 35), (c, 15)] Q6.pop(); test(Q6.front() == "c"); test(Q6.size() == 2); // [(e, 35)] Q6.pop(); test(Q6.front() == "e"); test(Q6.size() == 1); // Another test of decrease_key() in more complex cases MinPriorityQueue Q7; string s; for (char c = 'a'; c 90000; --l) { string s = Q8.front(); int p = priority2(s); test(Q8.size() == l); Q8.pop(); test(p >= prev_p); prev_p = p; } // Small test of everything with a different type (char) MinPriorityQueue Q9; test(Q9.size() == 0); Q9.push('a', 10); test(Q9.size() == 1); Q9.push('c', 6); test(Q9.size() == 2); Q9.push('f', 8); test(Q9.size() == 3); Q9.push('m', 7); test(Q9.size() == 4); Q9.push('z', 15); test(Q9.size() == 5); Q9.push('q', 11); test(Q9.size() == 6); Q9.push('d', 3); test(Q9.size() == 7); test(Q9.front() == 'd'); Q9.pop(); test(Q9.size() == 6); test(Q9.front() == 'c'); Q9.pop(); test(Q9.size() == 5); test(Q9.front() == 'm'); Q9.pop(); test(Q9.size() == 4); test(Q9.front() == 'f'); Q9.decrease_key('q', 2); test(Q9.front() == 'q'); Q9.pop(); test(Q9.size() == 3); test(Q9.front() == 'f'); Q9.pop(); test(Q9.size() == 2); test(Q9.front() == 'a'); Q9.pop(); test(Q9.size() == 1); test(Q9.front() == 'z'); Q9.pop(); test(Q9.size() == 0); cout 1 Introduction The goal of this homework is to build a min priority queue that can be used as part of the solution to a later homework, hwMZ2. This homework is unlike previous homeworks in that you'll implement a template class. If you recall, a template class is both declared and implemented in a common header file, so the implementation will be done by modify a provided header file.1 vector > H "Tiger" 65eopard "l4Elephant "Jr "Lemur" 2000er "Otter" 250nda" 4000er r""Leopard""Elephant" pair6500 45000 unordered map I 5 0 2 3 int "Elephant" "Lemur"Leopard"Panda""Otter""Tiger" Figure 1: A MinPriorityQueue for a set of 6 strings 2 Instructions The following files have been given to you: 1. A C++ header file (minpriorityqueue.h incorrectly implementing the MinPriorityQueue template class. 2. A C source file (main.cpp) containing a main function with tests. Correct the implementation of the template class in minpriorityqueue.h so that the provided files compile into a program that runs with no failed tests. Submit the header file minpriorityqueue.h. 1 Introduction The goal of this homework is to build a min priority queue that can be used as part of the solution to a later homework, hwMZ2. This homework is unlike previous homeworks in that you'll implement a template class. If you recall, a template class is both declared and implemented in a common header file, so the implementation will be done by modify a provided header file.1 vector > H "Tiger" 65eopard "l4Elephant "Jr "Lemur" 2000er "Otter" 250nda" 4000er r""Leopard""Elephant" pair 6500 45000 unordered map I 5 0 2 3 int "Elephant" "Lemur"Leopard"Panda""Otter""Tiger" Figure 1: A MinPriorityQueue for a set of 6 strings 2 Instructions The following files have been given to you: 1. A C++ header file (minpriorityqueue.h incorrectly implementing the MinPriorityQueue template class. 2. A C source file (main.cpp) containing a main function with tests. Correct the implementation of the template class in minpriorityqueue.h so that the provided files compile into a program that runs with no failed tests. Submit the header file minpriorityqueue.h
Step by Step Solution
There are 3 Steps involved in it
Step: 1
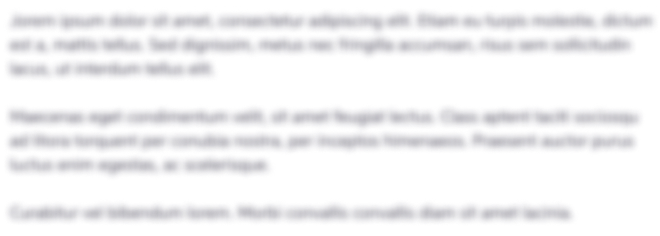
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started