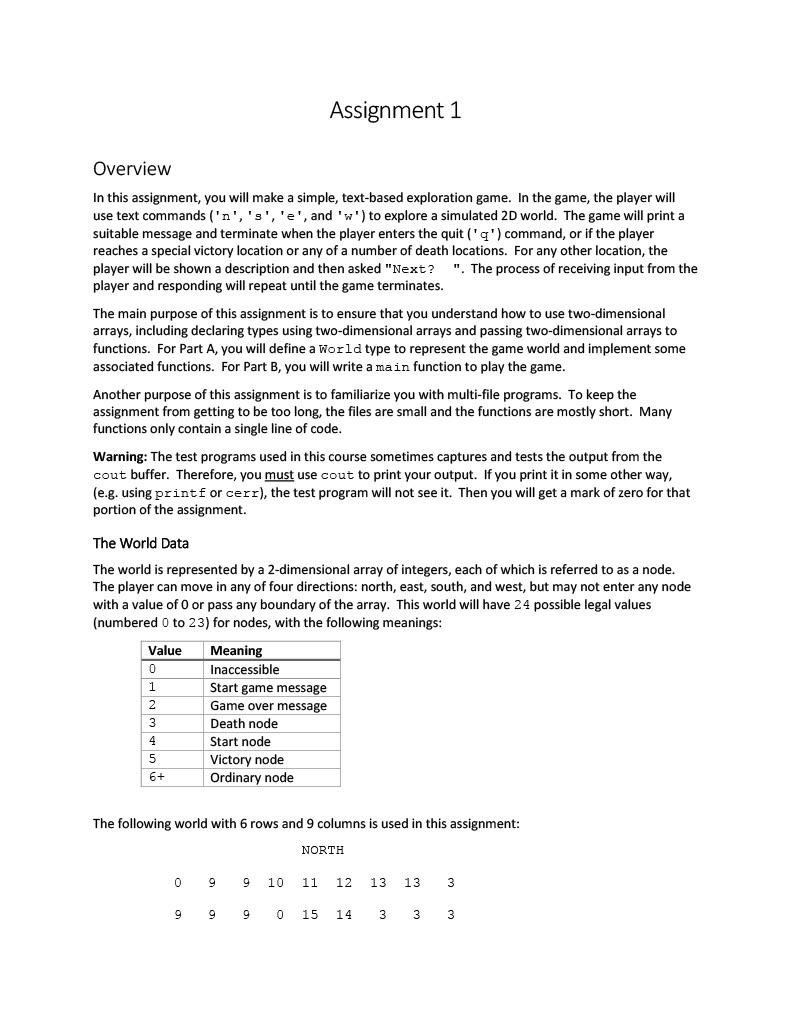
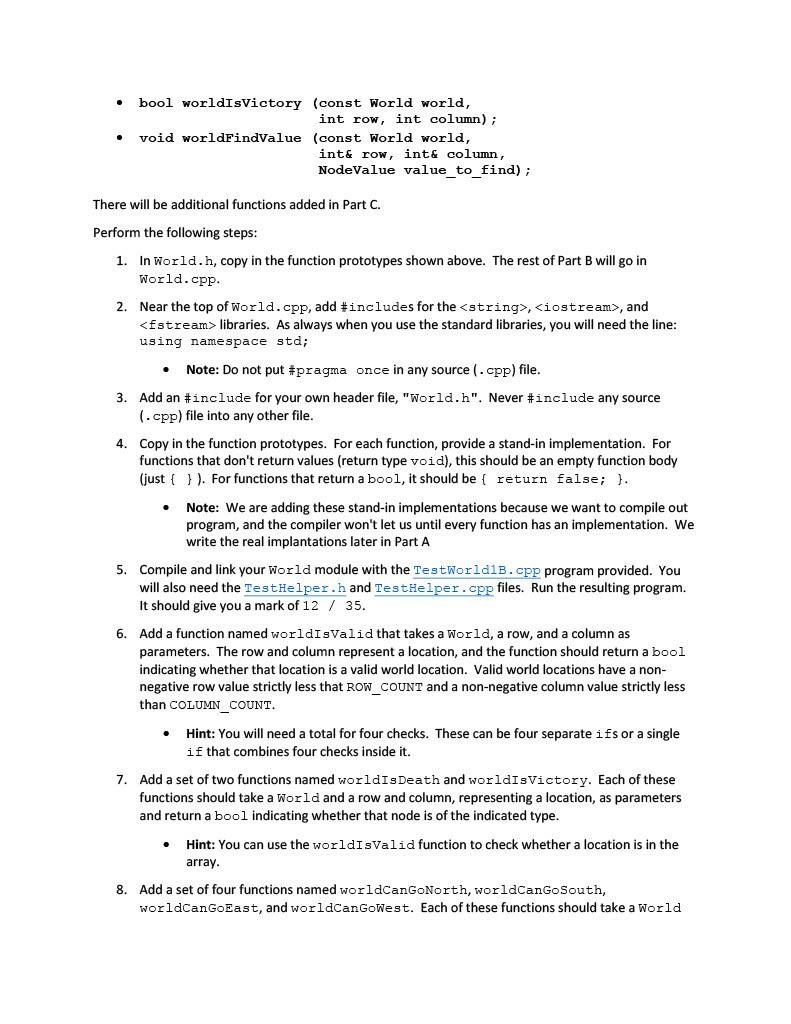
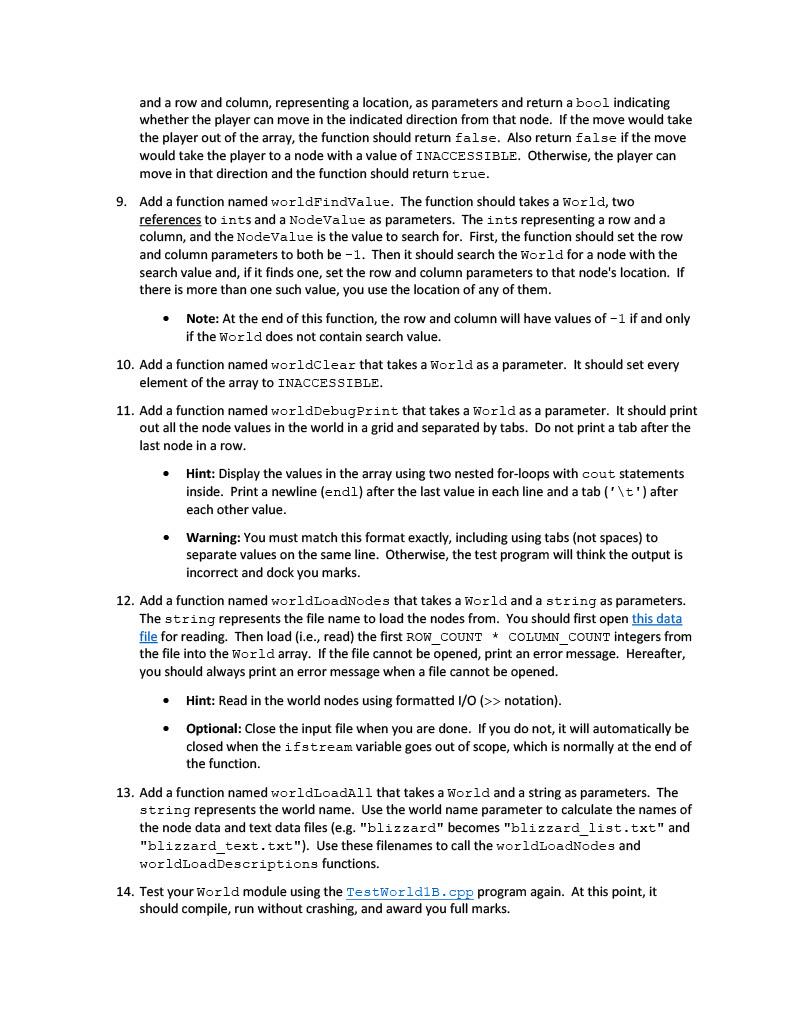
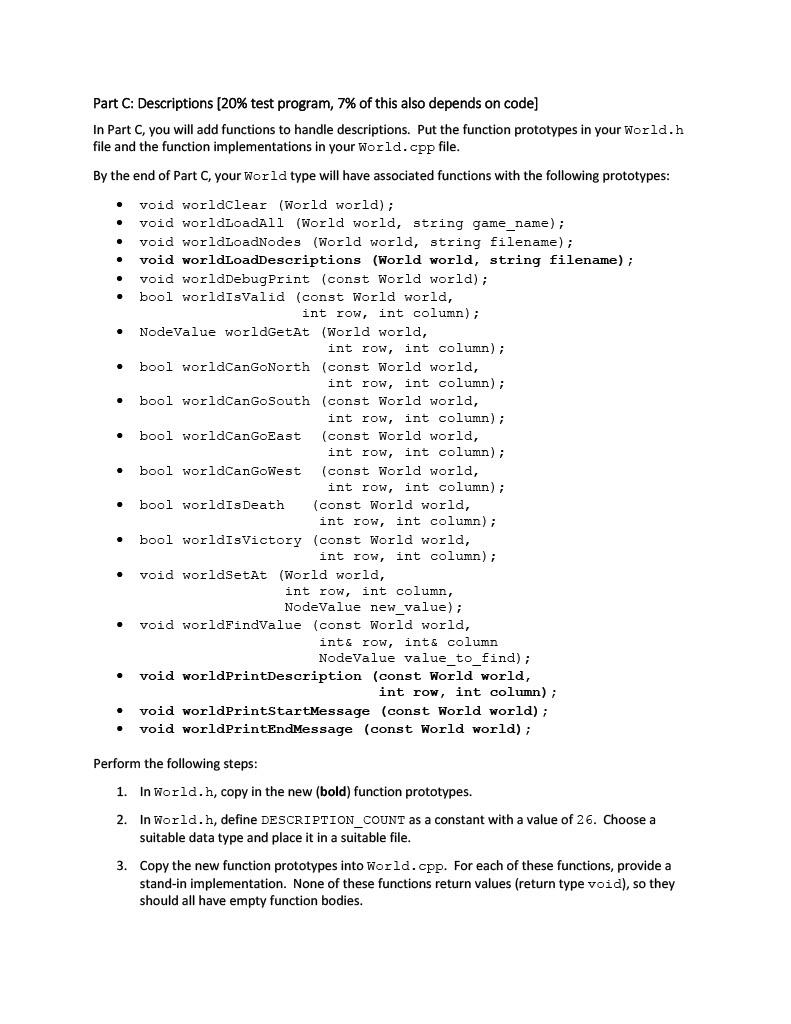
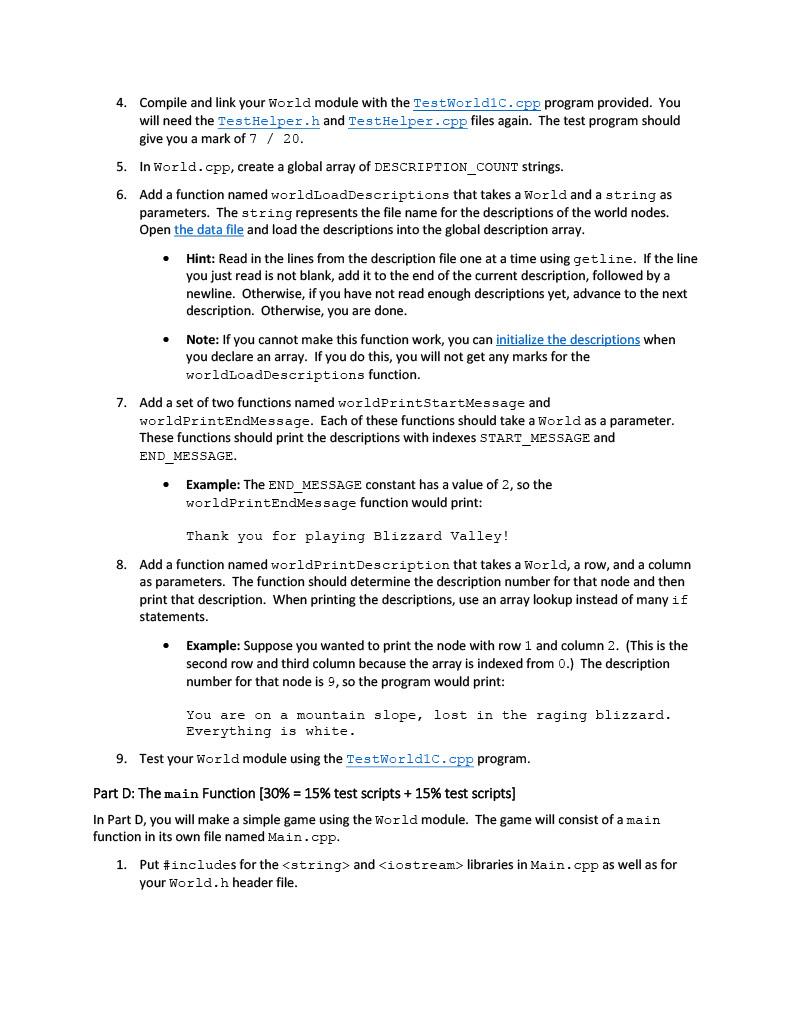
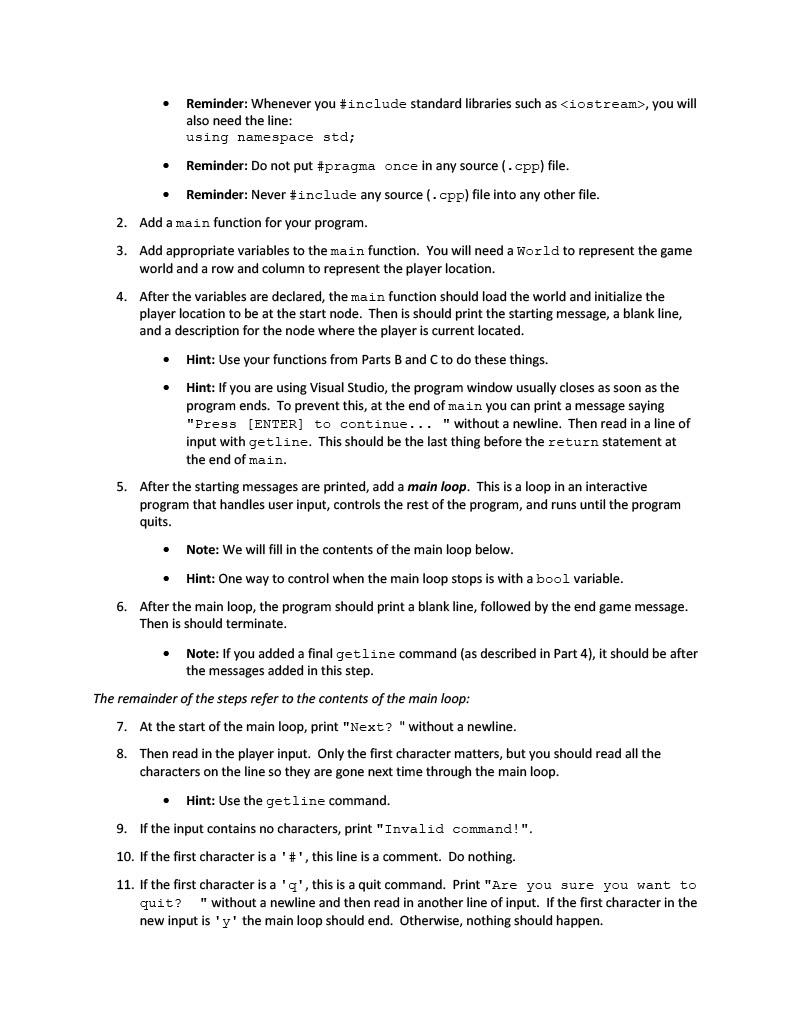
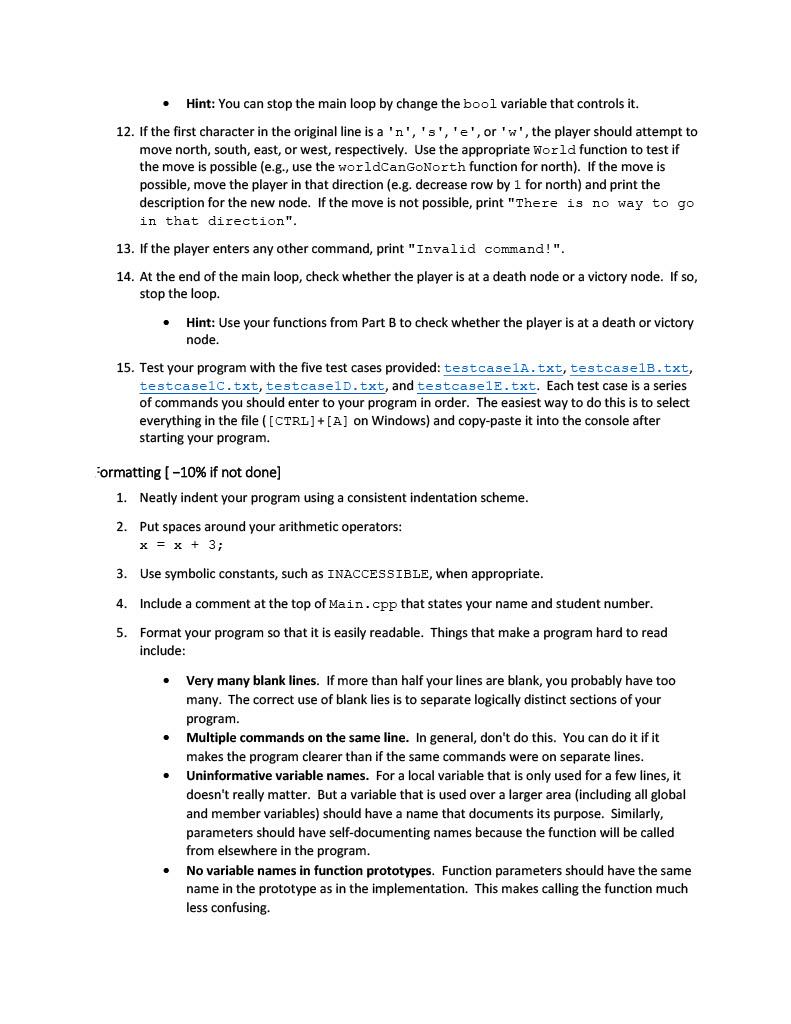
C++ ..............please..........I know how to do Part A and Part B upto 6 but do know the next steps also I cannot do C and D. Please please do it. Just right the code syntax
Assignment 1 Overview In this assignment, you will make a simple, text-based exploration game. In the game, the player will use text commands ('n', 's', 'e', and 'w') to explore a simulated 2D world. The game will print a suitable message and terminate when the player enters the quit ('') command, or if the player reaches a special victory location or any of a number of death locations. For any other location, the player will be shown a description and then asked "Next? ". The process of receiving input from the player and responding will repeat until the game terminates. The main purpose of this assignment is to ensure that you understand how to use two-dimensional arrays, including declaring types using two-dimensional arrays and passing two-dimensional arrays to functions. For Part A, you will define a World type to represent the game world and implement some associated functions. For Part B, you will write a main function to play the game. Another purpose of this assignment is to familiarize you with multi-file programs. To keep the assignment from getting to be too long, the files are small and the functions are mostly short. Many functions only contain a single line of code. Warning: The test programs used in this course sometimes captures and tests the output from the cout buffer. Therefore, you must use cout to print your output. If you print it in some other way, (e.g. using printf or cerr), the test program will not see it. Then you will get a mark of zero for that portion of the assignment. The World Data The world is represented by a 2-dimensional array of integers, each of which is referred to as a node. The player can move in any of four directions: north, east, south, and west, but may not enter any node with a value of 0 or pass any boundary of the array. This world will have 24 possible legal values (numbered 0 to 23) for nodes, with the following meanings: Value Meaning 0 Inaccessible 1 Start game message 2 Game over message 3 Death node Start node 5 Victory node 6+ Ordinary node The following world with 6 rows and 9 columns is used in this assignment: 9 NORTH 0 9 9 10 11 12 13 13 3 9 9 9 0 15 14 3 3 3 . bool worldIsVictory (const World world, int row, int column); void worldFindValue (const World world, int& row, int & column, NodeValue value_to_find); There will be additional functions added in Part C. . Perform the following steps: 1. In World.h, copy in the function prototypes shown above. The rest of Part B will go in World.cpp. 2. Near the top of World.cpp, add #includes for the
, , and libraries. As always when you use the standard libraries, you will need the line: using namespace std; Note: Do not put #pragma once in any source (.cpp) file. 3. Add an #include for your own header file, "World.h". Never #include any source (.cpp) file into any other file. 4. Copy in the function prototypes. For each function, provide a stand-in implementation. For functions that don't return values (return type void), this should be an empty function body (just { } ). For functions that return a bool, it should be { return false; ). Note: We are adding these stand-in implementations because we want to compile out program, and the compiler won't let us until every function has an implementation. We write the real implantations later in Part A 5. Compile and link your World module with the TestWorld18.cpp program provided. You will also need the TestHelper.h and TestHelper.cpp files. Run the resulting program. It should give you a mark of 12 / 35. 6. Add a function named worldIsValid that takes a World, a row, and a column as parameters. The row and column represent a location, and the function should return a bool indicating whether that location is a valid world location. Valid world locations have a non- negative row value strictly less that ROW_COUNT and a non-negative column value strictly less than COLUMN COUNT. Hint: You will need a total for four checks. These can be four separate ifs or a single if that combines four checks inside it. 7. Add a set of two functions named worldIsDeath and worldIsVictory. Each of these functions should take a World and a row and column, representing a location, as parameters and return a bool indicating whether that node is of the indicated type. . Hint: You can use the worldIsValid function to check whether a location is in the array. 8. Add a set of four functions named worldCanGoNorth, worldCanGoSouth, worldCanGoEast, and worldCanGoWest. Each of these functions should take a World and a row and column, representing a location, as parameters and return a bool indicating whether the player can move in the indicated direction from that node. If the move would take the player out of the array, the function should return false. Also return false if the move would take the player to a node with a value of INACCESSIBLE. Otherwise, the player can move in that direction and the function should return true. 9. Add a function named worldFindvalue. The function should takes a World, two references to ints and a NodeValue as parameters. The ints representing a row and a column, and the NodeValue is the value to search for. First, the function should set the row and column parameters to both be -1. Then it should search the world for a node with the search value and, if it finds one, set the row and column parameters to that node's location. If there is more than one such value, you use the location of any of them. Note: At the end of this function, the row and column will have values of -1 if and only if the World does not contain search value. 10. Add a function named worldclear that takes a World as a parameter. It should set every element of the array to INACCESSIBLE. 11. Add a function named worldDebugPrint that takes a World as a parameter. It should print out all the node values in the world in a grid and separated by tabs. Do not print a tab after the last node in a row. Hint: Display the values in the array using two nested for-loops with cout statements inside. Print a newline (endl) after the last value in each line and a tab ('\t') after each other value. Warning: You must match this format exactly, including using tabs (not spaces) to separate values on the same line. Otherwise, the test program will think the output is incorrect and dock you marks. 12. Add a function named worldLoadNodes that takes a world and a string as parameters. The string represents the file name to load the nodes from. You should first open this data file for reading. Then load i.e., read) the first ROW_COUNT * COLUMN_COUNT integers from the file into the World array. If the file cannot be opened, print an error message. Hereafter, you should always print an error message when a file cannot be opened. Hint: Read in the world nodes using formatted I/O (>> notation). Optional: Close the input file when you are done. If you do not, it will automatically be closed when the ifstream variable goes out of scope, which is normally at the end of the function. 13. Add a function named worldLoadAll that takes a world and a string as parameters. The string represents the world name. Use the world name parameter to calculate the names of the node data and text data files (e.g. "blizzard" becomes "blizzard_list.txt" and "blizzard_text.txt"). Use these filenames to call the worldLoadNodes and worldLoadDescriptions functions. 14. Test your World module using the TestWorld1b.cpp program again. At this point, it should compile, run without crashing, and award you full marks. Part C: Descriptions (20% test program, 7% of this also depends on code] In Part C, you will add functions to handle descriptions. Put the function prototypes in your world.h file and the function implementations in your World.cpp file. . . . . . By the end of Part C, your World type will have associated functions with the following prototypes: void worldclear (World world); void worldLoadAll (World world, string game_name); void worldLoadNodes (World world, string filename); void worldLoadDescriptions (World world, string filename); void worldDebugPrint (const World world); bool worldIsValid (const World world, int row, int column); NodeValue worldGetAt (World world, int row, int column); bool worldCanGoNorth (const World world, int row, int column); bool worldCanGoSouth (const world world, int row, int column); bool worldCanGoEast (const World world, int row, int column); bool worldCanGoWest (const World world, int row, int column); bool worldIs Death (const World world, int row, int column); bool worldIsVictory (const World world, int row, int column); void worldSetAt (World world, int row, int column, NodeValue new_value); void worldFindvalue (const World world, int& row, int& column NodeValue value_to_find); void worldPrintDescription (const World world, int row, int column); void worldPrintStartMessage (const World world); void worldPrintEndMessage (const World world); . . . . Perform the following steps: 1. In World.h, copy in the new (bold) function prototypes. 2. In World.h, define DESCRIPTION_COUNT as a constant with a value of 26. Choose a suitable data type and place it in a suitable file. 3. Copy the new function prototypes into World.cpp. For each of these functions, provide a stand-in implementation. None of these functions return values (return type void), so they should all have empty function bodies. 4. Compile and link your world module with the TestWorldic.cpp program provided. You will need the TestHelper.h and TestHelper.cpp files again. The test program should give you a mark of 7 / 20. 5. In World.cpp, create a global array of DESCRIPTION_COUNT strings. 6. Add a function named worldLoadDescriptions that takes a world and a string as parameters. The string represents the file name for the descriptions of the world nodes. Open the data file and load the descriptions into the global description array. . Hint: Read in the lines from the description file one at a time using getline. If the line you just read is not blank, add it to the end of the current description, followed by a newline. Otherwise, if you have not read enough descriptions yet, advance to the next description. Otherwise, you are done. . Note: If you cannot make this function work, you can initialize the descriptions when you declare an array. If you do this, you will not get any marks for the worldLoadDescriptions function. 7. Add a set of two functions named worldPrintStartMessage and worldPrintEndMessage. Each of these functions should take a World as a parameter. These functions should print the descriptions with indexes START_MESSAGE and END_MESSAGE. Example: The END_MESSAGE constant has a value of 2, so the worldPrintEndMessage function would print: Thank you for playing Blizzard Valley! 8. Add a function named worldPrint Description that takes a world, a row, and a column as parameters. The function should determine the description number for that node and then print that description. When printing the descriptions, use an array lookup instead of many if statements. Example: Suppose you wanted to print the node with row 1 and column 2. (This is the second row and third column because the array is indexed from 0.) The description number for that node is 9, so the program would print: You are on a mountain slope, lost in the raging blizzard. Everything is white. 9. Test your World module using the TestWorldic.cpp program. Part D: The main Function (30% = 15% test scripts + 15% test scripts] In Part D, you will make a simple game using the world module. The game will consist of a main function in its own file named Main.cpp. 1. Put #includes for the and libraries in Main.cpp as well as for your World.h header file. Reminder: Whenever you #include standard libraries such as , you will also need the line: using namespace std; Reminder: Do not put #pragma once in any source (.cpp) file. Reminder: Never #include any source (.cpp) file into any other file. 2. Add a main function for your program. 3. Add appropriate variables to the main function. You will need a world to represent the game world and a row and column to represent the player location. 4. After the variables are declared, the main function should load the world and initialize the player location to be at the start node. Then is should print the starting message, a blank line, and a description for the node where the player is current located. Hint: Use your functions from Parts B and C to do these things. Hint: If you are using Visual Studio, the program window usually closes as soon as the program ends. To prevent this, at the end of main you can print a message saying "Press [ENTER] to continue... " without a newline. Then read in a line of input with getline. This should be the last thing before the return statement at the end of main. 5. After the starting messages are printed, add a main loop. This is a loop in an interactive program that handles user input, controls the rest of the program, and runs until the program quits. . Note: We will fill in the contents of the main loop below. . Hint: One way to control when the main loop stops is with a bool variable. 6. After the main loop, the program should print a blank line, followed by the end game message. Then should terminate. Note: If you added a final getline command (as described in Part 4), it should be after the messages added in this step. The remainder of the steps refer to the contents of the main loop: 7. At the start of the main loop, print "Next? " without a newline. 8. Then read in the player input. Only the first character matters, but you should read all the characters on the line so they are gone next time through the main loop. Hint: Use the getline command. 9. If the input contains no characters, print "Invalid command!". 10. If the first character is a '#',this line is a comment. Do nothing. 11. If the first character is a 'q', this is a quit command. Print "Are you sure you want to quit? " without a newline and then read in another line of input. If the first character in the new input is 'y' the main loop should end. Otherwise, nothing should happen. . Hint: You can stop the main loop by change the bool variable that controls it. 12. If the first character in the original line is a 'n', 's', 'e', or 'w', the player should attempt to move north, south, east, or west, respectively. Use the appropriate World function to test if the move is possible (e.g., use the worldCanGoNorth function for north). If the move is possible, move the player in that direction (e.g. decrease row by 1 for north) and print the description for the new node. If the move is not possible, print "There is no way to go in that direction". 13. If the player enters any other command, print "Invalid command!". 14. At the end of the main loop, check whether the player is at a death node or a victory node. If so, stop the loop. . Hint: Use your functions from Part B to check whether the player is at a death or victory node. 15. Test your program with the five test cases provided: testcase1A.txt, testcaselB.txt, testcase1c.txt, testcaselD.txt, and testcaselE.txt. Each test case is a series of commands you should enter to your program in order. The easiest way to do this is to select everything in the file ([CTRL]+[A] on Windows) and copy-paste it into the console after starting your program. Formatting ( -10% if not done] 1. Neatly indent your program using a consistent indentation scheme. 2. Put spaces around your arithmetic operators: x = x + 3; 3. Use symbolic constants, such as INACCESSIBLE, when appropriate. 4. Include a comment at the top of Main.cpp that states your name and student number. 5. Format your program so that it is easily readable. Things that make a program hard to read include: . Very many blank lines. If more than half your lines are blank, you probably have too many. The correct use of blank lies is to separate logically distinct sections of your program. Multiple commands on the same line. In general, don't do this. You can do it if it makes the program clearer than if the same commands were on separate lines. Uninformative variable names. For a local variable that is only used for a few lines, it doesn't really matter. But a variable that is used over a larger area (including all global and member variables) should have a name that documents its purpose. Similarly, parameters should have self-documenting names because the function will be called from elsewhere in the program. No variable names in function prototypes. Function parameters should have the same name in the prototype as in the implementation. This makes calling the function much less confusing