Question
C++ Pointer Problem: Segmentation Fault Error I wrote this program to create a circular doubly linked list, however when I compile I continuously get a
C++ Pointer Problem: Segmentation Fault Error
I wrote this program to create a circular doubly linked list, however when I compile I continuously get a segmentation fault error and I cannot figure out why. Apologies for the long code!
#include
#include
#include
#include
using namespace std;
struct entry_QB{
string firstName, lastName;
int years[5];
int wins;
entry_QB *next;
entry_QB *prev;
};
void addEntry(string, string, int);
void removeEntry(string, string);
void printEntry();
void deleteList();
entry_QB * searchList(string, string );
entry_QB *HEAD = NULL;
int main(){
ifstream infile("Lab 6_QB_List.txt");
string firstN, lastN;
int year = 0;
while(!infile.eof()){
infile >> firstN;
infile >> lastN;
infile >> year;
addEntry(firstN, lastN, year);
}
int choice = 0;
while(1){
cout << "What function would you like? ";
cout << "Enter 1, to add an entry ";
cout << "Enter 2, to delete an entry ";
cout << "Enter 3, to search for an entry ";
cout << "Enter 4, to print list ";
cout << "Enter 5, to clear list and quit ";
cin >> choice;
if(choice == 1){
cout << "Enter the first name, last name, and year each separated by a space ";
cin >> firstN >> lastN;
cout << "Enter year ";
cin >> year;
addEntry(firstN, lastN, year);
cout << "New entry added/n";
break;
}
else if(choice == 2){
cout << "Enter the first name and last name separated by a space ";
cin >> firstN >> lastN;
removeEntry(firstN, lastN);
cout << "Entry deleted ";
break;
}
else if(choice == 3){
cout << "Enter the first name and last name separated by a space ";
cin >> firstN >> lastN;
searchList(firstN, lastN);
cout << "Entry found ";
break;
}
else if(choice == 4){
cout << "Enter the first name and last name separated by a space ";
cin >> firstN >> lastN >> year;
searchList(firstN, lastN);
break;
}
else if(choice == 5){
deleteList();
break;
}
else{
cout << "Invalid Input ";
}
}
deleteList();
return 0;
}
void addEntry(string firstN, string lastN, int year){ //put the entry at the end of the line
entry_QB *newEntry = new entry_QB;
entry_QB *temp;
entry_QB *TAIL;
if(HEAD == NULL){
HEAD = newEntry;
HEAD -> firstName = firstN;
HEAD -> lastName = lastN;
HEAD -> years[0] = year;
HEAD -> wins = 1;
HEAD -> next = HEAD;
HEAD -> prev = HEAD;
}
else{
temp = searchList(firstN, lastN);
if(temp){
temp -> years[newEntry -> wins] = year;
temp -> wins++;
}
else{
temp = newEntry;
temp -> firstName = firstN;
temp -> lastName = lastN;
temp -> years[0] = year;
temp -> wins = 1;
//for(temp = HEAD; temp != NULL; temp = temp -> next){
for(TAIL = HEAD; TAIL != temp; TAIL = TAIL -> next){
if(temp -> next = HEAD){
//newEntry -> next = HEAD;
//HEAD -> prev = newEntry;
TAIL -> next = temp;
temp -> prev = TAIL;
}
}
temp -> next = HEAD;
HEAD -> prev = temp;
}
}
}
void removeEntry(string firstName, string lastName){
entry_QB *temp = searchList(firstName, lastName);
temp->prev->next = temp->next;
temp->next->prev = temp->prev;
delete temp;
}
void printEntry(){
//
}
void deleteList(){
entry_QB *temp;
temp = HEAD->next;
while(temp){
entry_QB *deleted = temp;
temp = temp->next;
delete deleted;
}
}
entry_QB * searchList(string firstN, string lastN){
entry_QB *temp;
temp = HEAD;
if((temp->firstName == firstN) && (temp->lastName == lastN)){
return temp;
}
for(temp = HEAD->next; temp != HEAD; temp = temp->next){
if((temp->firstName == firstN) && (temp->lastName == lastN)){ //Check if the passed in first name and last name are equal to the current temp entry
return temp; //Returns the address of the correct entry
}
}
temp = NULL;
return temp;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
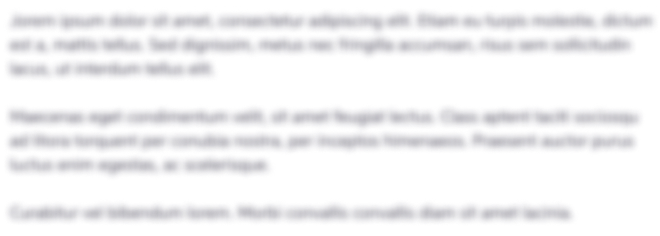
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started