Question
C++ Points Inside Rectangles Use the Vec.h and Rect.h classes. Write a program to do the following: - First, your program will continuously read as
C++
Points Inside Rectangles
Use the "Vec.h" and "Rect.h" classes.
Write a program to do the following: - First, your program will continuously read as input lines with 4 floats. Each set of 4 floats will define a 2D rectangle using your Rect class. Add each Rect to a container data structure of your choice, such as std::vector. Stop reading rectangles when your program reads 4 negative float values (do not create the rectangle with 4 negative float values). - Next, continuously read as input lines with 2 floats. Each pair of floats here will define a 2D point using your Vec class. For each point, print out its classification (whether it is inside or outside) with respect to all previously read rectangles. Stop reading points and end the program when you read (-99.0f,-99.0f).
EXAMPLE RUN: Type 4 floats to define a rectangle: -3 3 6 6 Type 4 floats to define the next rectangle: -2 2 4 4 Type 4 floats to define the next rectangle: -1 1 2 2 Type 4 floats to define the next rectangle: -1 -1 -1 -1
Type 2 floats to define a point: 0 0 The point is INSIDE of rectangle 0 The point is INSIDE of rectangle 1 The point is INSIDE of rectangle 2
Type 2 floats to define the next point: 2 0 The point is INSIDE of rectangle 0 The point is INSIDE of rectangle 1 The point is OUTSIDE of rectangle 2
Type 2 floats to define the next point: 2.5 1 The point is INSIDE of rectangle 0 The point is OUTSIDE of rectangle 1 The point is OUTSIDE of rectangle 2
Type 2 floats to define the next point: 4 4 The point is OUTSIDE of rectangle 0 The point is OUTSIDE of rectangle 1 The point is OUTSIDE of rectangle 2
Type 2 floats to define the next point: -99 -99 Finished!
Vec.h
#ifndef VEC_H
#define VEC_H
using namespace std;
class Vec {
public:
float x, y;
Vec() : x(0.0f), y(0.0f) {}
Vec(float x_, float y_) : x(x_), y(y_) {}
void set(float x_, float y_) {
x = x_;
y = y_;
}
void add(const Vec& other) {
x += other.x;
y += other.y;
}
void print() const {
cout << "(" << x << ", " << y << ") ";
}
};
#endif
Rect.h
#ifndef RECT_H
#define RECT_H
#include "Vec.h"
class Rect {
private:
float x, y, width, height;
public:
Rect(float x_, float y_, float width_, float height_) :
x(x_), y(y_), width(width_), height(height_) {}
bool contains(const Vec& point) const {
return (point.x >= x && point.x <= x + width && point.y <= y && point.y >= y - height);
}
};
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
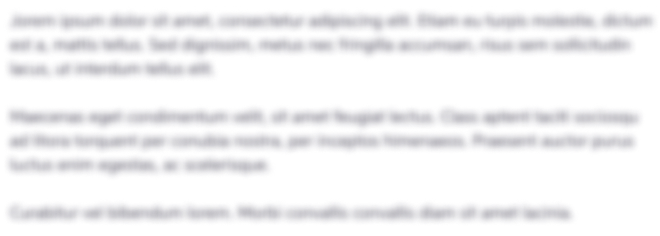
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started