Question
C PRGORAM: I implement and use a number of functions relating to file IO, pointer *array allocation/manipulation, and output formatting. NO USE OF GLOBAL VARIABLES
C PRGORAM: Iimplement and use a number of functions relating to file IO, pointer *array allocation/manipulation, and output formatting. NO USE OF GLOBAL VARIABLES #include#include #include #define MAX_LINE 100 #define MAX_NAME 30 int countLinesInFile(FILE* fPtr); int findPlayerByName(char** names, char* target, int size); int findMVP(int* goals, int* assists, int size); void printPlayers(int* goals, int* assists, char** names, int size); void allocateMemory(int** goals, int** assists, char*** names, int size); void sortPlayersByGoals(int* goals, int* assists, char** names, int size); void writeToFile(FILE* fPtr, int* goals, int* assists, char** names, int size); void readLinesFromFile(FILE* fPtr, int* goals, int* assists, char** names, int numLines); // Bonus function void bonusSortPlayersByBoth(int* goals, int* assists, char** names, int size); int main(int argc, char** argv) {
// Main function receives two command line arguments. The first is the name // of the input file; the second is the name of the output file. If either one // is missing, or the input file cannot be opened, the program should print an // error message and end.
} int countLinesInFile(FILE* fPtr) {
// Counts how many lines are in the file provided. After counting, // this function should rewind the file for future use. // // INPUT: fPtr - A pointer to a file opened in read mode. // RETURNS: The number of lines in the file.
} int findPlayerByName(char** names, char* target, int size) {
// Finds a player by their name. // // INPUT: names - A pointer to the strings containing the player names. // target - The name of the player to find. // size - The number of players to search through. // RETURNS: The index of the player if found; -1 otherwise.
} int findMVP(int* goals, int* assists, int size) {
// Finds the most valuable player (MVP) of the players. (The MVP has the greatest goals + assists total.) // // INPUT: goals - An array containing the number of goals scored per player. // assists - An array containing the number of assists per player. // size - The number of players in each array. // RETURNS: The index of the MVP. You may assume that size > 0 and therefore, there will always be // an MVP. In the event of a tie, you may return any of the players in the tie.
} void printPlayers(int* goals, int* assists, char** names, int size) {
// Prints the players names along with their goals and assists. // YOU MUST USE FORMATTED OUTPUT IN THIS FUNCTION. Failing to do so // will result in a loss of half of the points for this function. // Players should be printed out as follows: // // Name Goals Assists // Backes 4 2 // Pietrangelo 3 1 // // You must include the column headers in your output. THIS FUNCTION, // AND MAIN, SHOULD BE THE ONLY OUTPUT-PRODUCING FUNCTIONS IN YOUR PROGRAM. // If other functions produce output, you will lose half of the points for // those functions. It is very important not to produce errant output! // // INPUT: goals - An array containing the number of goals per player. // assists - An array containing the number of assists per player. // names - An array containing the names of each player. // size - The number of players in each array.
} void allocateMemory(int** goals, int** assists, char*** names, int size) {
// Allocates memory for the player names, their goals, and their assists. // HINT: Allocating the memory for the goals and assists is straightforward; // allocating memory for the names is going to be very similar to lab 2, with // an extra dereference to account for the fact that we are using a char***. // // INPUT: goals - A pointer to a variable which should contain the malloced memory for goals. // assists - A pointer to a variable which should contain the malloced memory for assists. // names - A pointer to a variable which should contain the malloced memory for names. // size - The number of players to malloc memory for.
} void sortPlayersByGoals(int* goals, int* assists, char** names, int size) {
// Sorts the players according to the number of goals they've scored, in descending order. // You must use insertion sort or selection sort for this function. // USE OF ANY OTHER SORTING FUNCTION WILL RESULT IN NO POINTS FOR THIS FUNCTION. // Keep in mind that moving an entry in one array will require you to // move entries in the other two arrays, to keep player information synchronized. // (There are a few ways to accomplish this with names, some easier than others.) // // INPUT: goals - An array containing the number of goals per player. // assists - An array containing the number of assists per player. // names - An array containing the names of each player. // size - The number of players in each array.
} void writeToFile(FILE* fPtr, int* goals, int* assists, char** names, int size) {
// Writes the player information to the given file, in the same order as the input file. // You should not call this function until after you have sorted the player information, // so that the information appears in sorted order in the output file. // // INPUT: fPtr - A pointer to a file to write to. // goals - An array containing the number of goals per player. // assists - An array containing the number of assists per player. // names - An array containing the names of each player. // size - The number of players in each array.
} void readLinesFromFile(FILE* fPtr, int* goals, int* assists, char** names, int numLines) {
// Reads the player information from the given file. Information is in the following format: // // [name] [goals] [assists] // Backes 1 5 // Pietrangelo 2 7 // // YOU MAY NOT USE FSCANF IN THIS FUNCTION! Doing so will result in no points. Instead you // must use fgets in combination with the strtok function in order to break the line into // pieces. You can read about strtok online or in your textbook; email your TA with any // questions. // // INPUT: fPtr - A pointer to a file to read from. // goals - An array to be filled with the number of goals per player. // assists - An array to be filled with the number of assists per player. // names - An array to be filled with the names of each player. // size - The number of players in each array.
} void bonusSortPlayersByBoth(int* goals, int* assists, char** names, int size) {
// BONUS FUNCTION - worth 5 extra points. You may only receive points for the bonus // if you have implemented all other functions with no major flaws. This is up to the // discretion of your TA. // // This function sorts the players according to two fields - goals and assists. // Players should be sorted according to their goals scored first, then they will // be sorted by the number of assists they have scored as a second criteria. Example: // // Tarasenko 5 5 // Berglund 5 2 // Pietrangelo 2 7 // Perron 2 6 // McDonald 2 4 // Redden 2 0 // Backes 1 5 // // Note that within each grouping of players with the same number of goals, // they are subsequently sorted by the number of assists. If two players have // the same number in each category, you may place them in any order. // // Unlike the other sort function, you may use any type of sort algorithm to // implement this function. Note that this does not excuse you from implementing // the other sort function with the proper algorithm. It will probably be easiest // to simply call the other sort function from within this one, and then perform // the sub-sort afterwards.
}
Sample outputs
$ ./a.out input.txt output.txt
Read the following 9 players from the file.
Name Goals Assists
Redden 2 0
Berglund 5 2
Stewart 4 0
Oshie 3 5
McDonald 2 4
Pietrangelo 2 7
Perron 2 6
Tarasenko 5 5
Sorted player list.
Name Goals Assists
Berglund 5 2
Tarasenko 5 5
Stewart 4 0
Oshie 3 5
Redden 2 0
McDonald 2 4
Pietrangelo 2 7
Perron 2 6
MVP was Tarasenko with 10 points
Enter a player to find: Perron
Perron has 2 goals and 6 assists
------ BONUS ------
Name Goals Assists
Tarasenko 5 5
Berglund 5 2
Stewart 4 0
Oshie 3 5
Pietrangelo 2 7
Perron 2 6
McDonald 2 4
Redden 2 0
Step by Step Solution
There are 3 Steps involved in it
Step: 1
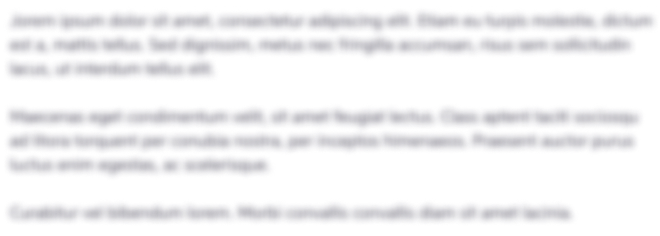
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started