Question
C++ Problem Make the following extensions to the Chapter #9, Problem #6 solution found in the following 3 source files located below. -the 2-file implementation
C++ Problem
Make the following extensions to the Chapter #9, Problem #6 solution found in the following 3 source files located below.
-the 2-file implementation of normalized, signed-magnitude rational numbers that separates the class RATIONAL interfacethe class definition found in Rational.hfrom its implementation (that is, the definitions of member functions) found in Rational.cpp
-Problem6.cpp, a simple application program used to drive the functionality of RATIONAL
Make copies of Rational.h, and Rational.cpp, call them Rational2.h and Rational2.cpp, respectively. Add the new functionality required by Items 1-to-3 below to the class RATIONAL, then use Problem6-2.cpp to drive the new functionality youve added.
Please post screenshot of it compiled and post source code
1. Add a public non-static member function to the class RATIONAL that returns the multiplicative inverse of (*this) without side effect[2].
RATIONAL Inv() const;
The multiplicative inverse of the signed magnitude rational number (*this) is defined S*N/D when N 0 but is undefined when N = 0.
2. Add a public non-static member function to the class RATIONAL that returns the additive inverse of (*this) without side effect.
RATIONAL Neg() const;
The additive inverse of (*this) is defined (-1*S)*N/D.
3a. Add a public non-static member function to the class RATIONAL that returns the quotient (*this) divided-by RHS without side effect.
RATIONAL Div(const RATIONAL RHS) const;
The quotient of (*this) = S1*N1/D1 and RHS = S2*N2/D2, N2 0, is defined
S1*N1/D1 S2*N2/D2 = (S1*S2)*(N1*D2)/(D1*N2)
but is undefined when N2 = 0.
3b. Add a public non-static member function to the class RATIONAL that returns the quotient LHS divided-by RHS as result (as an OUTy-ness side effect), but without side effects to either LHS or RHS.
void Div(const RATIONAL RHS,RATIONAL &result) const;
3c. Add a public static member function to the class RATIONAL that returns the quotient LHS divided-by RHS without side effects to either LHS or RHS.
versions. So, all that said, the Item 3c version must have a different function name, hence, Div2().
static RATIONAL Div2(const RATIONAL LHS,const RATIONAL RHS);
3d. Add a global-scoped non-member function (is there any other kind of scoping for non-member functions?!) that returns the quotient LHS divided-by RHS without side effects to either LHS or RHS.
RATIONAL Div(const RATIONAL LHS,const RATIONAL RHS)
//-------------------------------------------------------
// Mr Smith
// Chapter #9, Problem #6
// Rational.h
//-------------------------------------------------------
#ifndef RATIONAL_H
#define RATIONAL_H
#define SHOWLIFETIME
//-------------------------------------------------------
class RATIONAL
//-------------------------------------------------------
{
private:
int S; // { +1,-1 }
int N; // (N)umerator N >= 0
int D; // (D)enominator D > 0
private:
void Normalize();
static int GCD(int x,int y)
{
if ( y == 0 )
return( x );
else
return( GCD(y,x%y) );
}
public:
static RATIONAL Mul(const RATIONAL LHS,const RATIONAL RHS);
public:
RATIONAL(int N = 0,int D = 1);
~RATIONAL();
RATIONAL Add(const RATIONAL RHS) const;
void Sub(const RATIONAL RHS,RATIONAL &result) const;
void Print() const;
void Input();
// Added to answer some Quiz Questions
// RATIONAL(const RATIONAL &LHS): S(LHS.S),N(LHS.N),D(LHS.D)
// {
// cout
// }
};
#endif
//-------------------------------------------------------
// Mr Smith
// Chapter #9, Problem #6
// Rational.cpp
//-------------------------------------------------------
#include
#include
#include
using namespace std;
#include ".\Rational.h"
//-------------------------------------------------------
//private
void RATIONAL::Normalize()
//-------------------------------------------------------
{
S = +1;
if ( N
{
S *= -1;
N = -N;
}
if ( D
{
S *= -1;
D = -D;
}
int gcd = GCD(N,D);
N /= gcd;
D /= gcd;
}
//-------------------------------------------------------
RATIONAL::RATIONAL(int N,int D)
//-------------------------------------------------------
{
this->N = N;
this->D = D;
Normalize();
#ifdef SHOWLIFETIME
cout
#endif
}
//-------------------------------------------------------
RATIONAL::~RATIONAL()
//-------------------------------------------------------
{
#ifdef SHOWLIFETIME
cout
#endif
}
//-------------------------------------------------------
RATIONAL RATIONAL::Add(const RATIONAL RHS) const
//-------------------------------------------------------
{
RATIONAL r;
r.N = this->S*this->N*RHS.D + RHS.S*RHS.N*this->D;
r.D = D*RHS.D;
r.Normalize();
return( r );
}
//-------------------------------------------------------
void RATIONAL::Sub(const RATIONAL RHS,RATIONAL &result) const
//-------------------------------------------------------
{
RATIONAL r = RHS;
r.S *= -1;
result = this->Add(r);
}
//-------------------------------------------------------
//static
RATIONAL RATIONAL::Mul(const RATIONAL LHS,const RATIONAL RHS)
//-------------------------------------------------------
{
RATIONAL r;
r.N = LHS.S*LHS.N*RHS.S*RHS.N;
r.D = LHS.D*RHS.D;
r.Normalize();
return( r );
}
//-------------------------------------------------------
void RATIONAL::Print() const
//-------------------------------------------------------
{
if ( N == 0 )
cout
else
{
if ( S == -1 ) cout
cout
if ( D != 1 ) cout
}
}
//-------------------------------------------------------
void RATIONAL::Input()
//-------------------------------------------------------
{
cin >> N;
cin.ignore(11,'/');
cin >> D;
Normalize();
}
//-------------------------------------------------------
// Mr Smith
// Chapter #9, Problem #6
// Problem6.cpp
//-------------------------------------------------------
#include
#include
#include
using namespace std;
#include ".\Rational.h"
RATIONAL GLOBAL1(100,1);
//-------------------------------------------------------
int main()
//-------------------------------------------------------
{
void MyMain();
static RATIONAL STATIC1(200,1);
RATIONAL *HEAP1 = new RATIONAL (300,1);
MyMain();
RATIONAL AUTO1(400,1);
delete HEAP1;
system("PAUSE");
return( 0 );
}
RATIONAL GLOBAL2(500,1);
//-------------------------------------------------------
void MyMain()
//-------------------------------------------------------
{
cout
static RATIONAL STATIC2(600,1);
RATIONAL AUTO2(700,1);
RATIONAL *HEAP2 = new RATIONAL (800,1);
cout
{
cout
RATIONAL r;
cout
cout
}
{
cout
RATIONAL r,LHS(-23,75),RHS(-77,-150);
r = LHS.Add(RHS);
cout
cout
}
{
cout
RATIONAL r,LHS(-23,75),RHS(-77,-150);
LHS.Sub(RHS,r);
cout
}
{
cout
RATIONAL rs[] =
{
RATIONAL( -1, 2),
// If this RATIONAL constructor reference causes a compile time error, delete
// the *& operator pair and make the constructor reference "look like" the one above.
*&RATIONAL( 2, -4),
*(new RATIONAL( 4, 10))
};
RATIONAL *product = new RATIONAL(-1,-1);
for (int i = 0; i
{
*product = RATIONAL::Mul(rs[i],*product);
cout Print(); cout
}
delete product;
}
delete HEAP2;
cout
}
//-------------------------------------------------------
// Dr. Art Hanna
// Chapter #9, Problem #6
// Problem6-2.cpp
//-------------------------------------------------------
#include
#include
#include
using namespace std;
#include ".\Rational2.h"
//-------------------------------------------------------
int main()
//-------------------------------------------------------
{
{
cout
RATIONAL r(-3,2);
cout
cout
}
{
cout
RATIONAL r(-3,2);
cout
cout
}
{
//-------------------------------------------------------
// RATIONAL RATIONAL::Div(const RATIONAL RHS) const;
//-------------------------------------------------------
cout
RATIONAL LHS(-3,-2),RHS(-4,3);
cout
}
{
//-------------------------------------------------------
// void RATIONAL::Div(const RATIONAL RHS,RATIONAL &result) const;
//-------------------------------------------------------
cout
RATIONAL r,LHS(-3,-2),RHS(-4,3);
LHS.Div(RHS,r);
cout
}
{
//-------------------------------------------------------
// static RATIONAL RATIONAL::Div2(const RATIONAL LHS,const RATIONAL RHS);
//-------------------------------------------------------
cout
RATIONAL LHS(-3,2),RHS(4,3);
cout
}
{
//-------------------------------------------------------
// global RATIONAL Div(const RATIONAL LHS,const RATIONAL RHS);
//-------------------------------------------------------
cout
RATIONAL LHS(-3,2),RHS(4,3);
cout
}
system("PAUSE");
return( 0 );
}
Problem6.cpp Sample Dialog (with SHOWLIFETIME macro not defined EACOURSESICS2313 eginning HyHai . sizeof RATIONAL12 Test Input and Output Test Add i -0, partial product --1/2 product-1/ partial product 1/18 Press any key to continue . . Problem6-2.cpp Expected Output (with SHOWLIFETIME macro not defined ENCOURSESCS231 Problem6-2.exe -3/2 Test Neg) Neg3/2 a. Test DivC b. Test Div> c. Test Div2) d. Test DivC> HS/RHS-9/8 ress any key to continue .. Problem6.cpp Sample Dialog (with SHOWLIFETIME macro not defined EACOURSESICS2313 eginning HyHai . sizeof RATIONAL12 Test Input and Output Test Add i -0, partial product --1/2 product-1/ partial product 1/18 Press any key to continue . . Problem6-2.cpp Expected Output (with SHOWLIFETIME macro not defined ENCOURSESCS231 Problem6-2.exe -3/2 Test Neg) Neg3/2 a. Test DivC b. Test Div> c. Test Div2) d. Test DivC> HS/RHS-9/8 ress any key to continueStep by Step Solution
There are 3 Steps involved in it
Step: 1
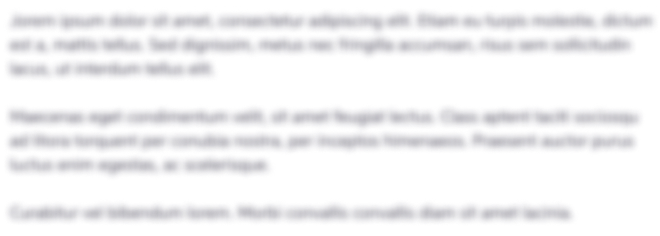
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started