Question
C. Progamming. Implement Actions and Functions I have to implement the function and action: tError bookTable_sortedAdd(tBookTable *tabBook, tBook book) so given a table of books
C. Progamming. Implement Actions and Functions
I have to implement the function and action:
tError bookTable_sortedAdd(tBookTable *tabBook, tBook book)
so given a table of books sorted according the criteria from the function book_cmp ( for the fields section, subsection, author and ISBN ) from lowest to highest and a book, if there is enough space in the table to displace all books higher then than the book we want to insert and to insert it in the right position.
void bookTable_sort(tBookTable tabBook, tBookTable *result)
so given a table of books, the program returns a table with the same books but sorted accoring to the criteria from the function book_cmp ( for the fields section, subsection, author and ISBN) lowest to highest. - I think we should use here a "while" algorithm in the original table and in the previous function in order to insert each book in a sorted manner in the table, that we will have previously emptied-
BOOKS.C
#include
BOOKS.H
#include "data.h" /* Get a textual representation of a book */ void getBookStr(tBook book, int maxSize, char *str); /* Get a book object from its textual representation */ tError getBookObject(const char *str, tBook *book); /* Compare two books by author name*/ int book_cmp(tBook t1, tBook t2); /* Copy the book data in src to dst*/ void book_cpy(tBook *dst, tBook src); /* Initialize the table of books */ void bookTable_init(tBookTable *bookTable); /* Add a new book to the table of books */ tError bookTable_add(tBookTable *table, tBook book); /* Find a book in the table */ int bookTable_find(tBookTable table, char *ISBN); /* Remove the first occurence of a book in the table */ void bookTable_del(tBookTable *table, tBook book); /* Load the table of books from a file */ tError bookTable_load(tBookTable *table, const char* filename); /* Save a table of books to a file */ tError bookTable_save(tBookTable table, const char* filename); void bookTable_filterBySection(
tBookTable tabBook, char sectionID, tBookTable *result); unsigned int bookTable_getOnLoanNumber(tBookTable tabBook); unsigned int bookTable_getAuthorNumber(tBookTable tabBook, char *author); tError bookTable_sortedAdd(tBookTable *tabBook, tBook book); void bookTable_sort(tBookTable tabBook, tBookTable *result); /* Release a books table */ void bookTable_release(tBookTable *table);
DATA.H
/* Uncomment the practice version you want to run */ #define SIMPLE_VERSION //#define COMPLETE_VERSION
/* This code ensures that this file is included only once */ #ifndef __DATA_H #define __DATA_H /* If the constant DATA_H is not defined (ifndef), the code is added, otherwise, this code is excluded. When the code is added, the constant is defined, therefore next time this file will be included it will be defined and no inclusion will be done. */
#define MAX_PATHNAME 256 #define MAX_LINE 512 #define MAX_SECTIONS 10 #define MAX_SECTION_NAME 100 #define MAX_BOOKS 300 #define MAX_SUB 10 #define MAX_BOOK_ISBN 14 #define MAX_BOOK_AUTHOR_CODE 4 #define MAX_BOOK_TITLE 101
/* Definition of a boolean type */ typedef enum {FALSE, TRUE} tBoolean;
/* Definition of the error type. */ typedef enum {OK=1, ERROR=0, ERR_CANNOT_READ=-1, ERR_CANNOT_WRITE=-2, ERR_MEMORY=-3, ERR_DUPLICATED_ENTRY=-4, ERR_INVALID_DATA=-5, ERR_ENTRY_NOT_FOUND=-6} tError;
/* Definition of a location */ typedef struct { char row; char column; char shelf; } tLocation;
/* Definition of a section */ typedef struct { char id; char name[MAX_SECTION_NAME]; tLocation init; } tSection;
/* Table of sections */ typedef struct { tSection table[MAX_SECTIONS]; int size; } tSectionTable;
/* Definition of a classification */ typedef struct { char secId; char subId; } tClass;
/* Definition of the book */ typedef struct { char ISBN[MAX_BOOK_ISBN]; unsigned short year; tBoolean avail; tClass clas; char author[MAX_BOOK_AUTHOR_CODE]; char title[MAX_BOOK_TITLE]; } tBook;
/* Table of books */ typedef struct { #ifdef SIMPLE_VERSION tBook table[MAX_BOOKS]; #endif #ifdef COMPLETE_VERSION /******************** PR2 - EX6B ********************/ #endif int size; } tBookTable;
/* Definition of the application data structure */ typedef struct { /* Path where data will be stored */ char path[MAX_PATHNAME]; /* sections table */ tSectionTable sections; /* Books table */ tBookTable books; } tAppData;
/******************** PR2 - EX2 *********************/ /* Books of a class */ typedef struct { char id; /* Table of books of the subsection */ #ifdef SIMPLE_VERSION #endif #ifdef COMPLETE_VERSION /******************** PR2 - EX6A ********************/ #endif } tSubInfo;
/* Classes of a section */ typedef struct { unsigned int totSecSubs; } tSectionInfo;
#endif /*__DATA_H*/
API.H
#include "data.h"
/* * Methods for application data management */
/* Initialize the application data */ void appData_init(tAppData *object);
/* Load the application data from file */ tError appData_load(tAppData *object);
/* Save the application data to a file */ tError appData_save(tAppData object);
/* Allow to assign a path to the application data */ void appData_setPath(tAppData *object, const char *path);
/* * API */
/* Return a table with the books */ tError getBooks(tAppData object, tBookTable *result);
/* Get the section information */ tError getBook(tAppData object, char *ISBN, tBook *book);
/* Add a new book */ tError addBook(tAppData *object, tBook book);
/* Remove a certain book */ tError removeBook(tAppData *object, tBook book);
/* Return the table of sections */ tError getSections(tAppData object, tSectionTable *result);
/* Get the section information */ tError getSection(tAppData object, char C1, tSection *section);
/* Add a new section */ tError addSection(tAppData *object, tSection section);
INFO.H
#include "data.h"
tError si_getSectionInfo(tBookTable tabB, tSectionTable tabS, char sectionId, tSectionInfo *si );
tBook si_getBook(tBookTable tabB, tSectionInfo si, unsigned int nSub, unsigned int nBook);
void si_listSectionInfo(tBookTable tabB, tSectionInfo si );
MENU.h
include "data.h" #include "api.h"
/* Request an option to the user and check its validity */ int getOption(int numOptions);
/* Define the main menu options type */ typedef enum {MAIN_MENU_LOAD, MAIN_MENU_SAVE, MAIN_MENU_BOOKS, MAIN_MENU_SECTIONS, MAIN_MENU_STATS, MAIN_MENU_EXIT} tMainMenuOptions;
/* Define the book management menu options type */ typedef enum {BOOK_MENU_LIST, BOOK_MENU_ADD, BOOK_MENU_DEL, BOOK_MENU_SORT, BOOK_MENU_EXIT} tBookMenuOptions;
/* Define the section management menu options type */ typedef enum {SEC_MENU_LIST, SEC_MENU_ADD, SEC_MENU_INFO, SEC_MENU_EXIT} tSectionMenuOptions;
/* Define the status menu options type */ typedef enum {STAT_MENU_ON_LOAN, STAT_MENU_AUTHOR, STAT_MENU_SECTION, STAT_MENU_EXIT} tStatsMenuOptions;
/* Print the main menu options */ void printMainMenuOptions();
/* Get the option for the main menu */ tMainMenuOptions getMenuOption();
/* Perform the actions for the main menu */ void mainMenu(tAppData *appData);
/* Print the book management menu options */ void printBookMenuOptions();
/* Get the option for the book management menu */ tBookMenuOptions getBooksMenuOption();
/* Perform the actions for the book management menu */ void bookMenu(tAppData *appData);
/* Print the section management menu options */ void printSectionMenuOptions();
/* Get the option for the section management menu */ tSectionMenuOptions getSectionMenuOption();
/* Perform the actions for the section management menu */ void secMenu(tAppData *appData);
/* Print the stats menu options */ void printStatsMenuOptions();
/* Get the option for the status menu */ tStatsMenuOptions getStatsMenuOption();
/* Perform the actions for the status menu */ void statsMenu(tAppData appData);
SECTIONS.H
#include "data.h"
/* Get a textual representation of a section */ void getSectionStr(tSection section, int maxSize, char *str);
/* Get a section object from its textual representation */ tError getSectionObject(const char *str, tSection *section);
/* Compare two sections */ int section_cmp(tSection s1, tSection s2);
/* Copy the section data in src to dst*/ void section_cpy(tSection *dst, tSection src);
/* Initialize the table of sections */ void secTable_init(tSectionTable *tabSec);
/* Add a new section to the table of sections */ tError secTable_add(tSectionTable *tabSec, tSection section);
/* Find a section in the table */ int secTable_find(tSectionTable tabSec, char sectionId);
/* Remove the first occurence of a section in the table */ void secTable_del(tSectionTable *tabSec, tSection section);
/* Load the table of sections from a file */ tError secTable_load(tSectionTable *tabSec, const char* filename);
/* Save a table of sections to a file */ tError secTable_save(tSectionTable tabSec, const char* filename);
Step by Step Solution
There are 3 Steps involved in it
Step: 1
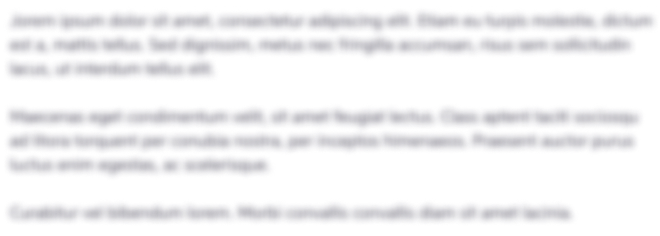
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started