Question
C++ Program A company wants to organize its inventory by cost from least to greatest . If the price of two items is the same,
C++ Program
A company wants to organize its inventory by cost from least to greatest. If the price of two items is the same, either one can be displayed first. You must use a member function of the appropriate corresponding struct to perform the comparison. Lastly, print all items in order by cost. You will need to save the output into a text file named SequencedOrders.txt. You must use constructors to initialize all data variables in structs with default values at the beginning of the program. This assignment needs the structs employee, condiment, food, plastic item, and date. NO global variables.
Below is the code that needs to be modified and the .txt files that go with it:
main.cpp
#include#include #include using namespace std; struct Date{ int day; int month; int year; }; struct Food{ string name; int numberLeft; Date sellByDate; double cost; }; struct Employee{ string name; int id; double salary; Date hireDate; int rating; }; struct Condiment{ string name; double ouncesLeft; Date sellByDate; double cost; }; struct PlasticItem{ string name; int numberLeft; double cost; }; /** These four functions are mandatory as instructed in the Assignment **/ void getFoodData(ifstream& inFile); void getEmployeeData(ifstream& inFile); void getCondimentData(ifstream& inFile); void getPlasticItemsData(ifstream& inFile); /** Optional Helper Functions **/ void displayFood(Food food[], int totalFood); void displayEmployees(Employee employee[], int totalEmployees); void displayCondiments(Condiment condiment[], int totalCondiments); void displayPlasticItems(PlasticItem plasticItem[], int totalPlastiicItems); void printHorizontalLine( int width, char border_char); void printHeading( string title, int width ); int main() { cout << "This is Assignment 1 (Structs) - Restaurant" << endl; /** Declaring the input streams for each file **/ ifstream inFileFood; ifstream inFileEmployee; ifstream inFileCondiment; ifstream inFilePlasticItem; inFileFood.open("Food.txt"); inFileEmployee.open("Employee.txt"); inFileCondiment.open("Condiment.txt"); inFilePlasticItem.open("PlasticItem.txt"); /** If the any of the file cannot be opened then the program terminates displaying the error message **/ if (!inFileFood) { cout << "The Food input file does not exist. Program terminates!" << endl; return 1; } if (!inFileEmployee) { cout << "The Employee input file does not exist. Program terminates!" << endl; return 1; } if (!inFileCondiment) { cout << "The Condiment input file does not exist. Program terminates!" << endl; return 1; } if (!inFilePlasticItem) { cout << "The PlasticItem input file does not exist. Program terminates!" << endl; return 1; } inFileFood.close(); inFileEmployee.close(); inFileCondiment.close(); inFilePlasticItem.close(); /** Display the prompt and do the requested action. Keep repeating the prompt until exit. If the number entered is not an option, just repeat the prompt. **/ int chosenOption; do{ cout << " Select which option you would like to see " << "1. Print all Food " << "2. Print all Employees " << "3. Print all Condiments " << "4. Print all Plastic Items " << "5. Exit " << " " << "Enter Option (1-5): "; cin >> chosenOption; /** Do the correct action according to the chosenOption **/ switch(chosenOption) { case 1: /** Food Function call to read data from input file. That function then calls a print Function **/ getFoodData(inFileFood); break; case 2: /** Employees Function call to read data from input file. That function then calls a print Function **/ getEmployeeData(inFileEmployee); break; case 3: /** Condiments Function call to read data from input file. That function then calls a print Function **/ getCondimentData(inFileCondiment); break; case 4: /** Plastic Items Function call to read data from input file. That function then calls a print Function **/ getPlasticItemsData(inFilePlasticItem); break; default: break; } }while(chosenOption != 5); return 0; } void printHorizontalLine( int width, char border_char){ cout.fill( border_char ); cout << setw( width ) << border_char << " "; cout.fill(' '); } void printHeading( string title, int width ){ //Declare Variables int magic_width = 0; magic_width = (width/2) - (title.length()/2) + title.length(); cout << " "; cout << left << setfill('=') << setw( width ) << "=" << " "; cout << right << setfill(' ') << setw( magic_width ) << title << " "; cout << right << setfill('=') << setw( width ) << "=" << endl; //reset count cout.clear(); cout.fill(' '); //VOID functions do NOT return a value } /** Using the input stream sent as parameter we are reading the content from the Food.txt and storing it in the food struct array **/ void getFoodData(ifstream& inFile){ inFile.open("Food.txt"); int totalFood; inFile >> totalFood; Food food[totalFood]; char decimal; for(int i = 0; i < totalFood; i++){ inFile >> food[i].name; inFile >> food[i].numberLeft; inFile >> food[i].sellByDate.month >> decimal >> food[i].sellByDate.day >> decimal >> food[i].sellByDate.year; inFile >> food[i].cost; } inFile.close(); printHeading("Food", 60); displayFood(food,totalFood); } /** Using the input stream sent as parameter we are reading the content from the condiments.txt and storing it in the condiments struct array **/ void getCondimentData(ifstream& inFile){ inFile.open("Condiment.txt"); int totalCondiments; inFile >> totalCondiments; Condiment condiments[totalCondiments]; char decimal; for(int i = 0; i < totalCondiments; i++){ inFile >> condiments[i].name; inFile >> condiments[i].ouncesLeft; inFile >> condiments[i].sellByDate.month >> decimal >> condiments[i].sellByDate.day >> decimal >> condiments[i].sellByDate.year; inFile >> condiments[i].cost; } inFile.close(); printHeading("Condiments", 60); displayCondiments(condiments,totalCondiments); } /** Using the input stream sent as parameter we are reading the content from the Employee.txt and storing it in the employees struct array **/ void getEmployeeData(ifstream& inFile){ inFile.open("Employee.txt"); int totalEmployees; inFile >> totalEmployees; Employee employees[totalEmployees]; char decimal; for(int i = 0; i < totalEmployees; i++){ inFile >> employees[i].name; inFile >> employees[i].id; inFile >> employees[i].salary; inFile >> employees[i].hireDate.month >> decimal >> employees[i].hireDate.day >> decimal >> employees[i].hireDate.year; inFile >> employees[i].rating; } inFile.close(); printHeading("Employees", 60); displayEmployees(employees,totalEmployees); } /** Using the input stream sent as parameter we are reading the content from the Personnel.txt and storing it in the plasticItem struct array **/ void getPlasticItemsData(ifstream& inFile){ inFile.open("PlasticItem.txt"); int totalPlasticItems; inFile >> totalPlasticItems; PlasticItem plasticItem[totalPlasticItems]; for(int i = 0; i < totalPlasticItems; i++){ inFile >> plasticItem[i].name; inFile >> plasticItem[i].numberLeft; inFile >> plasticItem[i].cost; } inFile.close(); printHeading("Plastic Items", 60); displayPlasticItems(plasticItem,totalPlasticItems); } /** Displaying the content from the food struct array on the monitor **/ void displayFood(Food food[], int totalFood){ cout << setw(10) << "Name" << " | " << "Number left" << " | " << "Sell By Date" << " | " << "Cost" << endl; printHorizontalLine(80,'-'); for(int i = 0; i < totalFood; i++){ cout << left << setw(9) << food[i].name << " | " << right << setw(6) << setfill(' ') << food[i].numberLeft << " | " << right < PlasticItem.txt 10 Forks 1000 .20 Spoons 215 .65 TrashBags 15 1.60 Napkins 5000 .20 Cups 60 .95 Plates 101 1.26 Bowls 15 2.10 Vincent 96 .55 Straws 180 1.05 Containers 280 1.32
Food.txt
15 Tomato 20 07:02:2015 1.95 Cucumber 89 06:15:2014 .89 Bread 4 06:25:2017 2.69 Eggs 11 08:29:2015 2.33 Turkey 56 05:01:2010 1.72 Orange 16 04:19:2011 .12 Lettuce 40 09:05:2002 .30 HotDog 22 05:20:2010 .99 Chicken 9 03:11:2016 1.01 Bun 1 06:24:2013 1.89 Burger 0 03:29:2016 66.60 Rib 16 06:15:2013 1.23 Apple 18 08:21:2012 1.87 Banana 44 10:22:2013 1.95 Carrot 21 09:22:2013 1.99
Condiment.txt
14 Ketchup 20 01:16:2000 1.00 Mustard 40 05:14:2006 4.20 Relish 15 10:21:2015 5.50 SoySauce 98 09:08:2011 2.70 Mayonnaise 52 06:03:2013 1.60 BBQSauce 11 11:10:2004 2.00 HotSauce 70 12:06:2014 .95 Salsa 700 06:12:2003 8.00 Wasabi 15 07:18:2006 1.00 OysterSauce 12 08:22:2007 2.60 Horseradish 200 08:23:2015 1.95 TarterSauce 90 06:25:2016 2.10 FishSauce 33 04:23:2015 3.23 BrownSauce 20 08:26:2014 2.00
Employee.txt
16 Ellis 209 32750 04:01:2010 10 Patrick 676 55549 03:10:2013 3 Tommy 257 53383 06:21:2000 3 Alfie 694 27177 06:01:2005 8 Harley 971 27040 07:06:2006 2 Jeffery 690 63713 02:26:2008 3 Bently 769 70142 02:27:2015 2 Kellen 468 10978 07:13:2012 1 Ryan 894 95825 08:17:2015 7 Alexander 633 41944 06:06:2016 1 Zak 619 9499 03:22:2015 5 Ashton 987 72388 06:13:2013 9 Mason 275 63900 04:14:2017 8 Alex 460 39871 07:23:2016 7 Emery 601 51050 03:20:2010 3 Wade 748 29487 02:28:2014 5
Step by Step Solution
There are 3 Steps involved in it
Step: 1
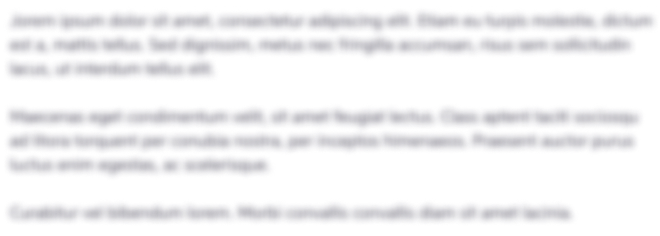
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started