Question
C PROGRAM: FIRST PART OF THE PROBLEM (DON'T NEED TO SOLVE IT, JUST NEED TO READ TO DO THE SECOND PART): Roller Coaster Design (coaster.c)
C PROGRAM:
FIRST PART OF THE PROBLEM (DON'T NEED TO SOLVE IT, JUST NEED TO READ TO DO THE SECOND PART):
Roller Coaster Design (coaster.c)
Problem C: Roller Coaster Design
Every great theme park has a signature roller coaster. In designing a roller coaster, we must decide how many trains of cars need to be placed on the track. It turns out that no more than 25% of the track can be occupied with these trains to adhere to safety regulations. Thus, if the track is 1000 feet long, and a train is 42 feet long, then up to 5 trains can fit on the track. (To see this, note that 5 trains have a total length of 210 feet, and this value is 21%, less than or equal to 25%, of the total track length of 1000 feet. Note that 6 trains would take up 252 feet, or 25.2% of the track, which is too much.)
In a train of cars, the first car is 10 feet long and all subsequent cars are 8 feet long. All cars seat up to 4 people. Since each of these values is constant, please use the following constants to store them:
#define FIRST_CAR_LENGTH 10
#define NORMAL_CAR_LENGTH 8
#define CAR_CAPACITY 4
For this program, the user will enter the total length of the track and the maximum length of the trains for the track. It is assumed that the trains formed will be as long as possible. For example, if the user enters 30 for the maximum length of the train, then the actual trains will have three cars and be of length 26, since a four car train would exceed 30 feet. Your program should calculate the number of people that can be supported on the track at one time. (Note: It may be the case that more people can be supported by making a shorter train than possible, but for this particular assignment, maximize the size of each train.)
Input Specification
1. The total length of the track will be a positive integer (in feet) less than 10000.
2. The maximum length of a train will be a positive integer in between 10 and 100.
Output Specification
Output the maximum number of passengers on the roller coaster at any one time with a single statement of the following format:
Your ride can have at most X people on it at one time.
Early Output Samples (later, Final outputs must look like Sample Runs 3 and 4)
At first, two sample outputs of running the program are included below. Note that these samples are NOT a comprehensive test. You should test your program with different data than is shown here based on the specifications given above.
Sample Run #1
What is the total length of the track, in feet?
1000
What is the maximum length of a train, in feet?
42
Your ride can have at most 100 people on it at one time.
(Note: Each train has 5 cars on it, and each car has 4 people, at most. Thus, 20 people can sit in one train. As previously established, 5 trains is the maximum for this track, so the maximum number of people this roller coaster can support is 100.)
Sample Run #2
What is the total length of the track, in feet?
1000
What is the maximum length of a train, in feet?
49
Your ride can have at most 100 people on it at one time.
Now, modify what you have written to do: If the Maximum Length exceeds the Actual Length print a message that "Maximum Train Length has surplus of %d feet" (and if no excess, print "Maximum Length fits exactly"); secondly, embed all of this (part C) code in a FOR loop that runs N times, where N is to be (prompted for and) read in at the beginning of the program. Thus, the user will input N and then will input the track_length and train_maximum N number of times. Given these new requirements, see Sample Runs #3 and #4. Your program must read the new input for the value of N, and must generate output similar to Sample Runs #3 and #4.
Sample Run #3
What is the value for N?
3
What is the total length of the track, in feet?
1000
What is the maximum length of a train, in feet?
49
Your ride can have at most 100 people on it at one time.
Maximum Train Length has surplus of 7 feet
What is the total length of the track, in feet?
1000
What is the maximum length of a train, in feet?
42
Your ride can have at most 100 people on it at one time.
Maximum Length fits exactly
What is the total length of the track, in feet?
1000
What is the maximum length of a train, in feet?
18
Your ride can have at most 104 people on it at one time.
Maximum Length fits exactly
Sample Run #4
What is the value for N?
1
What is the total length of the track, in feet?
1000
What is the maximum length of a train, in feet?
59
Your ride can have at most 112 people on it at one time.
Maximum Train Length has surplus of 1 feet
------------------------------------------------------------------------------------------------------------------------------------------------------------
SECOND PART OF THE PROBLEM (WRITE A CODE USING C):
Problem A: Roller Coaster Redesign (coaster2.c)
Your boss has noticed that maximizing the length of the train does not always maximize the number of passengers. Shes come up with a great idea for you to improve your program, so that you can calculate the actual maximum number of passengers the roller coaster can support. Her idea is as follows:
Lets say the maximum length of a train is 55. Then, we can simply start by trying out a train of length 10 (one car), and seeing how many passengers such a train would support. Then, we can try a train of length 18 (two cars) and recalculate the number of passengers this design would support. If this is better than the best design weve seen so far, simply save this new value. Continue in this fashion, until weve tried all possible trains. In this situation, we would try trains of lengths 10, 18, 26, 34, 42, and 50. (We stop here because the next train, of length 58, would be too long.) In each of these candidate lengths, compute the following quantity: total rollercoaster passengers divided by the total length of all the trains. Store each value in an array, so you will be storing as many values as there are lengths. Finally, compute the average of those values, and print it out as a final answer.
The user will input the same information as was inputted in assignment #1 part C. Note that this time you will not be inputting the number N, and you will not print the message about the surplus. This time, however, your program should output the actual number of maximum people the ride can support, the number of cars per train that achieves this maximum, and the final average of the passengers/length ratio.
Do the problem in small stages. First, ensure that you are getting the correct enumeration of the various train-lengths, then (for each train length) compute how many trains can fit on the track; that gives the number of people on the track simultaneously, check if this beats the max-so-far, if so, replace max. For each train length choice, you need to compute the ratio of people to cumulative car length, and (it is a good idea to) store this in a one-dimensional array that can store decimal values. Finally, compute the average of the ratios, and print that out.
Input Specification
1. The total length of the track will be a positive integer (in feet) less than 10000.
2. The maximum length of a train will be a positive integer in between 10 and 100, inclusive.
Output Specification
The output should consist of three lines. The first line outputs the maximum number of passengers on the roller coaster at any one time with a single statement of the following format:
Your ride can have at most X people on it at one time.
The second line should output the number of cars in the train that achieves this maximum with a single statement of the following format:
This can be achieved with trains of Y cars.
Note: If there are multiple ways in which the maximum number of people can be supported, output the smallest number of cars that achieves this maximum. (Thus, if both 2 cars and 4 cars lead to 100 people on the ride and this is the maximum, then your program should output 2 cars.)
The third line should simply output the average value of people to length, as:
AVG Ratio: XXXX.XXX
Output Samples
Two sample outputs of running the program are included below. Note that these samples are NOT a comprehensive test. You should test your program with different data than is shown here based on the specifications given above.
Sample Run #1
What is the total length of the track, in feet? 1000 What is the maximum length of a train, in feet? 42
Your ride can have at most 112 people on it at one time.
This can be achieved with trains of 4 cars.
AVG Ratio: 0.451
(Note: The maximum is achieved when each train has 4 cars on it, and each car has 4 people, at most. Thus, 16 people can sit in one train. This train has a length of 34 feet, and since 34 feet x 7 = 238 feet, which is less than 25% of the total track length, this means that exactly 7 trains can be placed on the track at the same time. Thus, 16 people/train x 7 trains = 112 people total.)
Sample Run #2
What is the total length of the track, in feet? 4025
What is the maximum length of a train, in feet? 89
Your ride can have at most 480 people on it at one time.
This can be achieved with trains of 6 cars.
AVG Ratio: 0.467
Step by Step Solution
There are 3 Steps involved in it
Step: 1
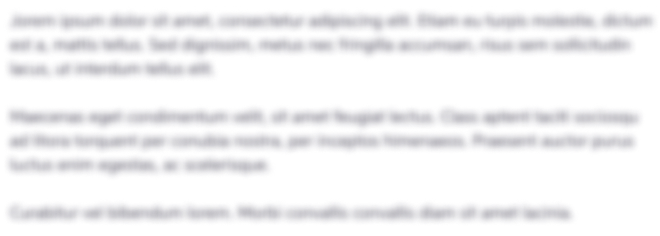
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started