Question
C++ Program Question, code below Hello - I completed Exercise 19.10 on page 815 of my C++ How to Program book. These are the instructions:
C++ Program Question, code below
Hello - I completed Exercise 19.10 on page 815 of my C++ How to Program book.
These are the instructions:
GOALS:
Implement a program in C++ using the STACK template class, LIST template class, and ListNode template class.
How to Use stack operations push and pop, and how to use implement a Stack with a List object.
To write a program that demonstrates how to use a Stack
Assignment:
Problem Description:
Write a program that inputs a line of text and uses a stack object to print the line reversed.
hint: your program could utilize list.h, listnode.h and stack.h class template definitions.
MY CODE:
#include "stdafx.h"
//Template ListNode class definition
#ifndef LISTNODE_H
#define LISTNODE_H
int _tmain(int argc, _TCHAR* argv[])
{
return 0;
}
//declaration of class List is required to announce that class
template class List;
template class ListNode
{
friend class List
public:
ListNode( const NODETYPE &); //constructor
NODETYPE getData() const; //return data
private:
NODETYPE data; //data
ListNode *nextPtr; /ext node in list
}; //end class ListNode
//constructor
template
ListNode
:data(info), nextPtr(0)
{
//empty body
} //end ListNode constructor
//return copy of data in code
template
NODETYPE ListNode :: getData() const
{
return data;
} //end function getData
#endif
#ifndef LIST_H
#define LIST_H
#include
using std::cout;
class list
{
public:
list(void);
~list(void);
};
#include "listnode.h" //ListNode class definition
template
class List
{
public:
List();
~List();
void insertAtFront( const NODETYPE &);
bool removeFromFront();
bool isEmpty() const;
void print() const;
private:
ListNode
ListNode
}; //end class list
//default constructor
template
List
:firstPtr(0)
{}
//destructor
template
List
{
if (!isEmpty()) //list not empty
{
ListNode
ListNode
while (currentPtr !=0) //delete node that remain
{
tempPtr = currentPtr;
currentPtr = currentPtr->nextPtr;
delete tempPtr;
} //end while
} //end if
cout
} //end destructor
//insert node at front of list
template
void List
{
ListNode
if (isEmpty()) //list is empty
firstPtr=newPtr; /ew list has only one node
else //list not empty
{
newPtr->nextPtr=firstPtr; //points new note to previous
firstPtr=newPtr; //aim firstPtr at new node
} //end else
} //end function insertAtFront
//delete node from front of the list
template
bool List
{
if (isEmpty()) //list empty
return false; //delete not successful
else
{
ListNode
firstPtr=firstPtr->nextPtr; //point to previous after removal
delete tempPtr; //claim previous front node
return true; //delete successful
} //end else
} //end function removeFromFront
//isList empty
template
bool List
{
return firstPtr == 0;
} //end function isEmpty
//return pointer to newly assigned node
template
ListNode
{
return new ListNode
} //end function getNewNode
//print elements of the list
template
void List
{
if (isEmpty())
{
cout
return;
} //end if
ListNode
//print new node
while (currentPtr !=0)
{
coutdata;
currentPtr=currentPtr=>nextPtr;
} //end while
cout
//end function print
#endif
#ifndef STACK_H
#define STACK_H
#include "list.h"
template
class Stack: private List
{
public:
//insert an element into the stack
void push(const STACKTYPE &data)
{
insertAtFront(data);
} //end function push
//delete top element of stack
bool pop()
{
return removeFromFront();
} //end function pop
// return regardless of whether stack is empty
bool isStackEmpty() const
{
return isEmpty();
} //end function isStackEmpty
//print elements of stack
void printStack() const
{
print();
} //end function printStack
};
#endif
#include
using std::cout;
int main() //main begins program execution
{
Stack
char str[75];
//prompt user for input, read input
cout
cout
std::cin.getline(str, 75, ' ');
//push input into stack
int i = 0;
while (str[i])
{
charStack.push(str[i]);
i++;
} //end while
// print, reverse string
cout
charStack.printStack();
return 0; //program executed successfully
} //end of function, main
THE PROBLEM:
I get the errors seen in this picture:
So my question is, how do I correct these? I put all the code in the main ConsoleApplication6.cpp file,
should I have created classes somehow? If so, how do I correct this and make it work?
Error List 0 Warnings Messages Search Error List 3 Errors Description File Line ^ C ^ Project ^ 1 error C1010: unexpected end of file while looking list.cpp 2 error C2995: 'List
Step by Step Solution
There are 3 Steps involved in it
Step: 1
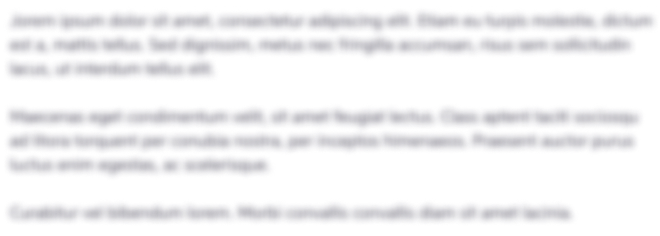
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started