Question
C++ PROGRAM ** the .txt file is the code below with comments & .XLS is the second image(excel spreadsheet) *** /* Program Data ID. #
C++ PROGRAM
** the .txt file is the code below with comments & .XLS is the second image(excel spreadsheet) *** /* Program Data ID. # C/Type First Name Last Name Rate Daily Hours Days Worked Earnings Ded. YTD Net YTD 101 F Michael Smith 5000 10000 40000 102 P Maria Rodriguez 25 10 14 7000 28000 103 P James Robert 25 8 12 4800 19200 104 P Kenny Mercedes 25 12 12 7200 28800 105 F Kyndra Hernandez 4000 8000 32000 */
//Note: tax and bonus are in percentage that 20% and 10% respectively /* Format for Final Report ID. # C/Type First Name Last Name Rate Hours Earnings Deductions NetPay Ded. YTD Net YTD 101 F Michael Smith A D=A * Tax(20%) G=A - D J=5000+D M=40000+G 105 F Kyndra Hernandez B E=B * Tax(20%) H=A - E K=4000+E N=32000+H Full-Time Sub-Total C=A+B F=D+E I=G + H L=J + K O=M + N 102 P Maria Rodriguez 20 P=Hours*Day T=P*Rate X=T * Tax(20%) AB=T - X AF=7000 + X AJ=28000 + AB 103 P James Robert 25 Q=Hours*Day U=Q*Rate Y=U * Tax(20%) AC=U - Y AG=4800 + Y AK=19200 + AC 104 P Kenny Mercedes 22 R=Hours*Day V=R*Rate Z=V * Tax(20%) AD=V - Z AH=7200 + Z AL=28800 + AD Part-Time Sub-Total S=P + Q + R W=T + U + V AA=X + Y + Z AE=AB+AC+AD AI=AF+AG+AH AM=AJ+AK+AL Total Summary AN=C + W AO=F + AA AP=I + AE AQ=L + AI AR=O + AM */
**** COPY AND PASTE CODE ****
#include
#include
#include
const float tax = 20;
const int bonus = 10;
class EmployeeData{
protected: float gross;
float deductions;
float netPay; f
loat netYTD;
float DedYTD;
public: int idNum;
char fName[15];
char lName[15];
char contract;
EmployeeData(){
//Feel free to add anything in here. }
void CalculateGross(){
cout
}
};
class FullTime : public EmployeeData{
protected:
float earnings;
public:
FullTime(int ivar=0, string fvar=" ", string lvar=" ", char cvar=' ', float dyvar=0, float nyvar=0){
idNum = ivar;
strcpy(fName, fvar.c_str());
strcpy(lName, lvar.c_str());
contract = cvar;
earnings = 0;
gross = 0;
deductions = 0;
netPay = 0;
DedYTD = dyvar;
netYTD = nyvar;
}
void CalculateGross(){
//coutgross = earnings;
}
};
class PartTime : public EmployeeData{
protected:
float payRate;
int hours;
int workDays;
public:
PartTime(int ivar=0, string fvar=" ", string lvar=" ", char cvar=' ', float dyvar=0, float nyvar=0){
idNum = ivar;
strcpy(fName, fvar.c_str());
strcpy(lName, lvar.c_str());
contract = cvar;
payRate = 0;
hours = 0;
workDays = 0;
gross = 0;
deductions = 0;
netPay = 0;
DedYTD = dyvar;
netYTD = nyvar;
cout
}
void CalculateGross(){
cout
gross = payRate * hours * workDays;
gross = payRate * hours * workDays; } }; //Note: //gross = earnings (for FullTime) //gross = payRate * hours * workDays (for PartTime) //deductions = gross * tax/100 /etPay = gross - deductions /etPay = netPay + (gross * (bonus/100)) (for only the PartTime object with the highest hours worked - hours * workDays) //Use the spreadsheet for more informationint main(){
EmployeeData *objptr;
FullTime ftobj1(101, "Michael", "Smith", 'F', 10000, 40000);
PartTime ptobj1(102, "Maria", "Rodriguez", 'P', 7000, 28000);
FullTime ftobj2(105, "Kyndra", "Hernandez", 'F', 8000, 32000);
PartTime ptobj2(103, "James", "Robert", 'P', 4800, 19200);
PartTime ptobj3(104, "Kenny", "Mercedes", 'P', 7200, 32000);
//Use the input operator to enter hour and earnings //for FullTime objects, input required is: earnings //for PartTime objects, input required are: payRate, hours and workDayscin >> ftobj1;
cin >> ftobj2;
cin >> ptobj1;
cin >> ptobj2;
cin >> ptobj3;
//Calculate gross income using member function already given in the classes and the EmployeeData pointer (objptr) //for FullTime objects - objptr->CalculateGross() must run the CalculateGross() in FullTime class and not another class //for PartTime objects - likewise objptr->CalculateGross() must run the CalculateGross() in PartTime class.
(objptr); objptr = &ftobj1;
objptr->CalculateGross();
objptr = &ftobj2; objptr->CalculateGross();
objptr = &ptobj1;
objptr->CalculateGross();
objptr = &ptobj2;
objptr->CalculateGross();
objptr = &ptobj3; objptr->CalculateGross();
//Calculate deduction and netPay using the substraction assignment operator overloading //deductions = gross * tax/100; netPay = gross - deductions;ftobj1 -= tax;
ftobj2 -= tax;
ptobj1 -= tax;
ptobj2 -= tax;
ptobj3 -= tax;
//Use (++) Prefix operator to update netYTP with netPay to reflect YTD-netPay /etYTD = netYTD + netPay;++ftobj1;
++ftobj2;
++ptobj1;
++ptobj2;
++ptobj3;
//Use Postfix (++) operator to update dedYTP with deductions to reflect total deductions to date in the financial year
//dedYTD = dedYTD + deductions;
ftobj1++;
ftobj2++;
ptobj1++;
ptobj2++;
ptobj3++;
//Use the relational operator overloading to find Part-time staff with the highest worked hours (workday*hours) and use function call () operator to add bonus (10% of gross) to the netPay
//to add bonus ==> netPay = netPay + (gross * bonus/100)if (ptobj1 > ptobj2){
if (ptobj1 > ptobj3){
ptobj1(bonus); /etPay = netPay + (gross * bonus/100
}
}
else{
if (ptobj2 > ptobj3){
ptobj2(bonus); /etPay = netPay + (gross * bonus/100)
}
else{
ptobj3(bonus); /etPay = netPay + (gross * bonus/100)
}
}
//Declared for data summary manipulations
FullTime totalftobj;
PartTime totalptobj;
PartTime totalptobj1;
PartTime totalptobj2;
//First use assignment operator to assign ptobj1 to totalptobj1 because you cant pass more than two arguments totalptobj1 = ptobj1;
//Attributes of interest for the summary report are: gross, deductions, netPay, netYTD, DedYTD//Use the + operator to sum the Deductions, netPay, DedYTD and netYTD for each group
totalptobj2 = ptobj2 + ptobj3;
//Use the + operator to sum the Deductions, netPay, DedYTD and netYTD for each grouptotalptobj = totalptobj1 + totalptobj2;
totalftobj = ftobj1 + ftobj3;
//Use output operator to display/print for example: cout
cout
Net YTD
cout
cout
// and continue as required.
return 0;
}
Purpose: This lab will help improve your understanding and knowledge of all the special topics we have discussed in class such as class inheritance, encapsulation, data hiding, and polymorphism. This exercise presents an opportunity to gain more programming skills and higher-level insight to C++ classes as you work on operators overloading, virtual functions and friend functions as member and non-member. Task: Complete the missing segments of the code in the attached text file "Lab 6-classes and operator overloading.txt" to calculate salary for employees. You are free to modify the codes where necessary. However, it is important that you keep the structure and program flow. Also, ensure that the entire program works. Due date is Sunday 11:59 PM EST Note: You cannot change any of the hard-coded requirements (anywhere you find this: 1 ...). Also, note that tax and bonus are in percentage; that is 20% and 10% respectively. Problem: Use the information provided in the spreadsheets "Lab 6 - classes and operator overloading.xls" to calculate employees' salary and print the paystub. The information on each paystub should contain: ID.#, First Name, Last Name, Earnings, Deductions, NetPay, DedYTD and NetYTD. (Almost like lesson 3 lab). Hints: Full-Time (Annual) Calculation: For a full-time salaried employee that makes $56,000 annually. The pay is monthly (12 pay periods in a year). Determining the employee's pay is a simple calculation and based on the business' pay schedule. It should look like this: $56,000 / 12 = $4,666.67. This is the employee's gross pay (earnings). 20% flat tax is applied to the above earnings. Part-Time Calculation: For a part-time hourly employee that makes $15 an hour. The pay is simply: Part-time employee's gross pay (earnings) = $15 x (Expected hours to work per day) x (Days worked) 20% flat tax is applied to the above earnings as well. Note: Part-time staff with the highest hours worked (workday*hours) gets a bonus (10% of gross) in addition to the netPay. Attached are the two files you need for this exercise: Lab 6 - classes and operator overloading.txt". Lab 6 - classes and operator overloading.xls". Contract ID. # Type First Name Last Name 101 F Michael Smith 102 P Maria Rodriguez 103 P James Robert 104 P Kenny Mercedes 105 F Kyndra Hernandez Expected Days Rate tours Per Day Worked Earnings Ded. YTD Net YTD 5000 10000 40000 20 10 14 7000 28000 25 8 12 4800 19200 22 12 12 7200 28800 4000 8000 32000 F=Full-Time Bonus for Part-time=20% Tax=20% on Gross P=Part-Time
Step by Step Solution
There are 3 Steps involved in it
Step: 1
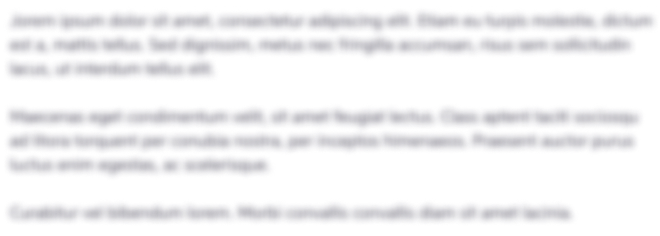
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started