Question
C++ Program: You are to write a program for the Peoria network of public libraries to keep track of books available at each of the
C++ Program:
You are to write a program for the Peoria network of public libraries to keep track of books available at each of the branches. Each branch has one string as its name. Each branch keeps its books in a linked list in lexicographical order by author's name. Books of the same author are arranged in lexicographical order by title. There may be many copies of the same book in a library branch.
The data structure to be used should be an array of structures, with the structure containing a branch_name field (string) followed by a pointer field.
The pointer field should point to an ordered linked list of the books in that branch. Each node of the linked list corresponds to a book and is a structure with an author_name field, a book_title field, a num_copies field which indicates how many copies of that book are available for checkout, and a pointer field pointing to the next book in the branch.
When a book is checked out from a branch, it is deleted from the branch's linked list. When it is returned to a branch, it is inserted in the appropriate place in the ordered list. A book can be returned to a different branch than the one it was checked out from.
Customers can ask to find how many books of a specific author and title are available in a given branch.
Your program should be menu driven with the menu containing the following options:
1. Create a branch and insert its books. (for each branch, the user creates the list of all books contained in the branch by repeated choice of menu choice number 1, as shown below. S/he inputs the first and last name of author, followed by the title. If more than one copy, each copy has to be added individually. When all books have been inserted to the branch, the user is to type none none none, and go to insert books into the next branch. Only after the whole library database has been created for all branches, the user will venture into menu choices 2 and higher).
2. Give an author name (first, last), the title and a branch name, to CHECKOUT that book from the branch, (if there is at least one copy of the book available, otherwise print that the branch holds no such book).
3. Give an author name (first, last), the title and a branch name, to RETURN that book to the branch. (books can be returned to a different branch than the one they were checked out from)
4. Give an author name (first, last), the title and a branch name, to FIND the number of copies of that book that are available in that branch (returns zero if there are none).
5. Give a branch name, to PRINT the author name (first, last) and title of all books contained in that branch.
6. Give a branch name and an author name, to FIND the number of books by that author contained in the branch.
7. Give an author name (first, last), to FIND the number of books by that author contained in all the branches of the library system.
8. Give an author name (first, last), to PRINT in lexicographical order the titles of all books by that author contained in all the branches of the library system.
9. Exit the program.
------
When the user chooses menu choice 1, the following dialogue for input of data should ensue:
What is your menu choice?
1
What is the name of the branch?
ALPHA
What is author and title of book?
JOHN SMITH FREEDOM
What is author and title of book?
MICHAEL JONES LIFE ADVENTURES
What is author and title of book?
NONE NONE NONE
Thank you, I created branch ALPHA
...menu is displayed again...
What is your menu choice?
1
What is the name of the branch?
BETA
What is author and title of book?
NAJ GUPTA ALGORITHMS
What is author and title of book?
NAJ GUPTA ALGORITHMS
What is author and title of book?
LEV LAGOS GENIUS
What is author and title of book?
NONE NONE NONE
Thank you, I created branch BETA
...menu is displayed again...
What is your menu choice?
7
Give an author name (first, last), to FIND the number of books by that author contained in all the branches of the library system.
LEV LAGOS
There are 1 books in the library system by LEV LAGOS
What is your menu choice?
2
Give author, title and branch from which to checkout the book:
LEV LAGOS GENIUS BETA
Thank you! The book GENIUS by LEV LAGOS has been checked out from BETA
...menu is displayed again...
What is your menu choice?
7
Give an author name (first, last), to FIND the number of books by that author contained in all the branches of the library system.
LEV LAGOS
There are 0 books in the library system by LEV LAGOS
What is your menu choice?
4
Give an author name (first, last), a title and a branch name, to FIND the number of copies of that book that are available in that branch (returns zero if there are none).
LEV LAGOS GENIUS BETA
There are 0 books in the library branch BETA by LEV LAGOS titled GENIUS
What is your menu choice?
2
Give author, title and branch from which to check out the book:
MATTHEW JONES LIFE ADVENTURES ALPHA Thank you! The book LIFE ADVENTURES by MATTHEW JONES has been checked out from ALPHA
...menu is displayed again...
What is your menu choice?
3
Give author, title and branch to which book is to be returned:
LEV LAGOS GENIUS APLPHA
Thank you! The book GENIUS by LEV LAGOS has been returned to branch ALPHA
...menu is displayed again...
What is your menu choice?
2
Give author, title and branch from which to checkout the book:
JOE JONES LIFE ALPHA
Sorry! This book is not available at branch ALPHA
...menu is displayed again...
What is your menu choice?
4
Give an author name (first, last), a title and a branch name, to FIND the number of copies of that book that are available in that branch (returns zero if there are none).
LEV LAGOS GENIUS ALPHA
There are 1 books in the library branch ALPHA by LEV LAGOS titled GENIUS
...menu choice is displayed again...
what is your menu choice?
5
Give a branch name, to PRINT the author name (first, last) and title of all books contained in that branch.
BETA
The books in BETA are:
ALGORITHMS by NAJ GUPTA number of copies 2
...menu is displayed again...
What is your menu choice?
9
Thank you and goodbye. //End of Program
The setup is an array of "branch" structures, each branch structure has two fields, the name of the branch and a pointer which is the head of a linked list
of "book" structures/booknodeTypes. The "book" nodeType has 5 fields, first name of author, last name of author, title of book, number of copies, pointer to the next book in the branch.
So, here is a possible setup:
//GIVEN CODE
#ifndef LIBBRANCH_GUARD
#define LIBBRANCH_GUARD
#include
#include
#include
struct BookType //the structure for each book
{
std::string author_first_name;
std::string author_last_name;
std::string book_title;
int num_copies;
BookType * next = nullptr;
};
typedef BookType * NodePtr; //will point to the next book in the library
struct BranchType // an array of BranchTypes, has a pointer to the books
{
std::string branchname;
NodePtr ptrToBooksInBranch;
};
class LibBranch
{
public:
LibBranch();
void Insert(std::string branch, std::string author, std::string title);
bool Delete(std::string branch, std::string author, std::string title);
int Find(std::string branch, std::string author, std::string title);
void newBranch(std::string name);
std::string toString(std::string branch, LibBranch name);
std::string getBranchName(int i, LibBranch name);
private:
static BranchType branchArr[100];
static int numBranches; //the number of branches stored in the branchArr
};
#endif
//Additional Code
// A O(n) solution for finding rank of string
#include
using namespace std;
#define MAX_CHAR 256
// A utility function to find factorial of n
int fact(int n)
{
return (n <= 1) ? 1 : n * fact(n - 1);
}
// Construct a count array where value at every index
// contains count of smaller characters in whole string
void populateAndIncreaseCount(int* count, char* str)
{
int i;
for (i = 0; str[i]; ++i)
++count[str[i]];
for (i = 1; i < MAX_CHAR; ++i)
count[i] += count[i - 1];
}
// Removes a character ch from count[] array
// constructed by populateAndIncreaseCount()
void updatecount(int* count, char ch)
{
int i;
for (i = ch; i < MAX_CHAR; ++i)
--count[i];
}
// A function to find rank of a string in all permutations
// of characters
int findRank(char* str)
{
int len = strlen(str);
int mul = fact(len);
int rank = 1, i;
// all elements of count[] are initialized with 0
int count[MAX_CHAR] = { 0 };
// Populate the count array such that count[i]
// contains count of characters which are present
// in str and are smaller than i
populateAndIncreaseCount(count, str);
for (i = 0; i < len; ++i) {
mul /= len - i;
// count number of chars smaller than str[i]
// fron str[i+1] to str[len-1]
rank += count[str[i] - 1] * mul;
// Reduce count of characters greater than str[i]
updatecount(count, str[i]);
}
return rank;
}
// Driver program to test above function
int main()
{
char str[] = "string";
cout << findRank(str);
return 0;
}
If you have questions, please mention what you need specification for, since this is all the information I was given. I really need help with it.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
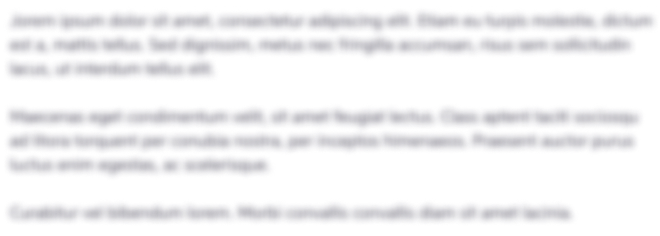
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started