Question
C++ Program Your program will calculate employees wages from data read from a file combined with data inputted by the user. The data in the
C++ Program
Your program will calculate employees wages from data read from a file combined with data inputted by the user. The data in the file consists of employee IDs and employees hourly rates. The program will read the file and store the employee IDs and hourly rates in parallel arrays. The program prompts the user to type in an employee ID, the number of hours worked by that employee, and searches for a match in the employee ID array. If a match is found, the program displays the hourly rate, calculates and displays the wage. The wage is calculated as hourly rate * number of hours worked. The program performs input validation: the employee ID must be >=1 and <=NUM_RANGE, and the number of hours must be >=0. If the input is invalid, the user is prompted to reenter the data.
1. Requirements
a) Functions
Your program must implement the following functions:
getData: This function takes as arguments an ID array and a rate array, reads from the input fil11e and populates the parallel arrays. The function returns the actual number of employees read from the file. If the file cannot be opened, your program should output an error message and exit.
search: This function takes as arguments an employee ID, an array, the actual number of employees, and searches the array for a match. The function returns the index of the element where a match is found, or -1 if no match is found. For this assignment, implement the linear search.
You are encouraged to implement additional functions to make your program more modular.
b) Assumptions
You can assume there are at most 1,000 employee records in the file, and set the array sizes to 1,000. However, the actual number of employees (determined by your getData function) is less or equal to 1,000.1
You can assume that each employee has a unique ID, and the possible ID values range from 1 to NUM_RANGE = 999999 inclusive.
Employee ID and rate are of type int
You can hard code the file name: "employeeFile.txt". The file is posted on eLearning.
c) Outline of main
Your main function must demonstrate your getData and search functions are working correctly by implementing this pseudo code:
Call getData while (user does not choose Quit)
Prompt the user to input an employee ID and number of hours Perform input validation Call search Depending on the outcome, print the employees rate and wage, or "Employee not found". The program also prints the number of comparisons it took to complete the search
d) Style
Make sure you follow the style requirements, especially regarding the comment header for functions, to avoid losing points.
2. Suggestions for Implementation
Apply the "divide and conquer" strategy by splitting the original problem into smaller pieces, and solve one piece at a time.
To print the number of comparisons, maintain a counter initialized at zero whenever a search starts, and incremented at each comparison.
3. Expected Output
This is an example of output if your implementation is correct. You can test your program by using the "HW1-2-Input" file. To save on typing, copy the content of the "HW1-2-Input" file, and paste it in the execution window, by right clicking the mouse.
4. Extra Credit (10 points)
You can earn up to 10 points extra credit if in addition, to the above, you implement binary search (you will have to implement a sorting algorithm to sort the data, since the data as read from the file is not sorted). Among the sorting algorithms learned in class, you can choose to implement the one you like.
e) Requirements
Normally when an array of values is sorted, the array is modified. However, in this case, we want to preserve the original employeeID and employeeRate arrays, and do the linear search on the original employeeID array. To preserve the original arrays, you need to copy them into duplicate arrays sortedEmployeeID and sortedEmployeeRate and perform the sorting on the duplicate arrays. The binary search will operate on the duplicate arrays.
f) Outline of main
Your main function must demonstrate your functions are working correctly by implementing this pseudo code (underlined are the additional parts required for extra credit)
Call getData
Copy data into duplicate arrays
Sort the duplicate arrays while (user does not choose Quit)
Prompt the user to input an employee ID and number of hours Perform input validation Call linear search (search on the original array) Depending on the outcome, print the employees rate and wage, or "Employee not found". The program also prints the number of comparisons it took to complete the search Call binary search (search on the duplicate array) Depending on the outcome, print the employees rate and wage, or "Employee not found". The program also prints the number of comparisons it took to complete the search
g) Implementation hints
Both the sortedEmployeeID and sortedEmployeeRate arrays should be sorted, and the sorting is done according to sortedEmployeeID.
5. Expected Output of Extra Credit
You can test your program by using the "HW1-2-Input" file. To save on typing, copy the content of the "HW1-2-Input" file, and paste it in the execution window, by right clicking the mouse
file content
00000042 87 00006335 40 00019170 64 00011479 98 00026963 84 00005706 85 00023282 47 00009962 31 00002996 42 00004828 96 00032392 64 00003903 93 00000293 82 00017422 96 00019719 75 00005448 66 00014772 38 00001870 92 00025668 79 00017036 74 00028704 71 00031323 33 00017674 44 00015142 51 00028254 88 00025548 64 00032663 57 00020038 79 00008724 81 00027530 78 00012317 95 00022191 22 00000289 46 00009041 82 00019265 28 00027447 65 00015891 29 00024371 90 00015007 81 00024394 48 00019630 83 00024085 54 00018757 20 00004967 36 00013932 88 00016945 59 00024627 63 00005538 38 00016119 22 00022930 81 00004834 95 00004640 78 00022705 30 00013978 86 00031674 86 00005022 45 00026925 52 00006271 89 00026778 73 00005098 52 00023987 30 00009162 96 00022356 67 00023656 74 00004032 72 00027351 50 00016942 64 00013967 90 00031108 51 00018008 77 00015458 67 00027754 83 00014946 49 00032210 98 00024222 48 00006423 86 00027507 90 00016414 68 00000901 51 00018763 75 00017411 59 00027625 77 00021549 23 00027596 61 00003603 50 00010292 56 00009375 80 00004597 41 00027349 99 00019669 24 00008282 34 00000054 99 00026419 38 00006901 48 00018128 87 00003729 33 00024649 23 00017808 41 00014311 77 00022814 94
User Input
22814 10 11122222 11479 -100 100 149 5 0
Step by Step Solution
There are 3 Steps involved in it
Step: 1
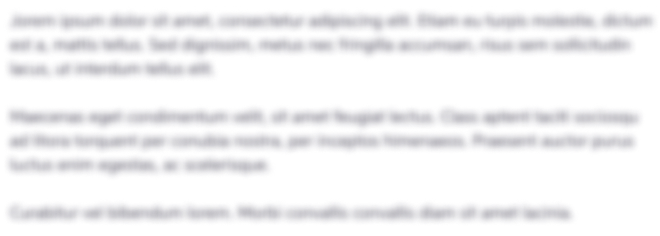
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started