Answered step by step
Verified Expert Solution
Question
1 Approved Answer
C + + Programming Assignment: Implementing a Simple Singly Linked List Objective: Create a singly linked list that supports adding nodes, printing the list, and
C Programming Assignment: Implementing a Simple Singly Linked List
Objective:
Create a singly linked list that supports adding nodes, printing the list, and deleting nodes with a
given string.
Assignment Details:
Create a Node Structure:
o Define a Node class with a std::string data field and a pointer to the next node.
o Include a default constructor, a parameterized constructor, and a destructor.
Implement an addnode Function:
o This function should take a pointer to the head of the linked list and a string to
add as a new node at the end of the list.
Implement a printlist Function:
o This function should take a pointer to the head of the linked list and print all the
nodes in the list.
Implement a deletenode Function:
o This function should take a pointer to the head of the linked list and a string to
delete the corresponding node if it exists.
Requirements:
Node Class Definition:
#include
#include
class Node
public:
std::string data;
Node next;
Default constructor
Node : data nextnullptr
Parameterized constructor
Nodestd::string d : datad nextnullptr
Destructor
~Node
Clean up resources if necessary
In this simple case, there's nothing specific to clean up
;
Add Node Function:
void addnodeNode& head, const std::string& newdatainsert code here
Print List Function:
void printlistNode head
insert code here
Delete Node Function:
void deletenodeNode& head, const std::string& target
insert code here
Example Usage:
int main
Node head nullptr;
Adding nodes
addnodehead "first";
addnodehead "second";
addnodehead "third";
Printing list
std::cout "List after adding nodes: ;
printlisthead;
Deleting a node
deletenodehead "second";
Printing list after deletion
std::cout "List after deleting 'second': ;
printlisthead;
Clean up memory
while head nullptr
Node temp head;
head headnext;
delete temp;
return ;
Additional Instructions:
Testing: Test the functions with various inputs to ensure they work correctly. Test edge
cases like deleting from an empty list or deleting a nonexistent node.
Memory Management: Ensure proper memory management to avoid memory leaks.
Delete all nodes before the program terminates.
Documentation: Comment the code to explain the logic behind each function.Submission:
Submit the C source code file containing the implementation of the linked list, including the
main function that demonstrates adding, printing, and deleting nodes.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
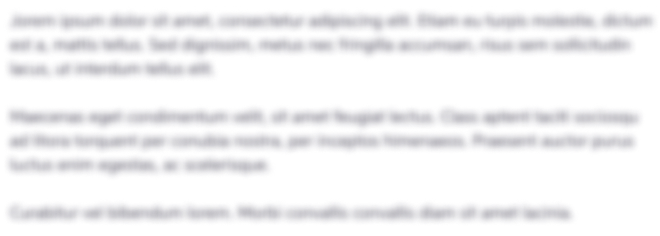
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started