Question
C PROGRAMMING Can someone help me compile my code? the goal is the get information from two text files called: rental.txt (which is filled as
C PROGRAMMING
Can someone help me compile my code? the goal is the get information from two text files called: rental.txt (which is filled as information for the rental function is p input) the other is cars.txt which has the createInventory information. Any help/advice would be appreciated :)
#include
#include
typedef struct {
int carId; // a unique ID of a car
char make[20]; // make of the car
char model[20]; // model of the car
int numDoors; // 2 or 4 door cars
double rate; // the rental rate of the car
} Car;
typedef struct {
char renterName[20]; // name of renter
int daysRenting; // days renting the car
int carId; // ID of the car rented
} Rental;
int createInventory(Car *allCars);
int addNewCar(Car *allCars, int totalCars, int size);
int addNewRental(Car *allCars, int totalCars, Rental *allRentals, int totalRentals, int size);
int findCarIDByMake(Car *allCars, int totalCars, char *carMake);
int findReservation(Rental *allRentals, int totalRentals, char *renterName);
int findCarById(Car *allCars, int totalCars, int carId);
void printAllRentals(Rental *allRentals, int totalRentals, Car *allCars, int totalCars);
void printCarInfo(Car *allCars, int totalCars, int carId);
double getAverageRentalDays(Rental *allRentals, int totalRentals);
// additional stuff
void writeRentals(char *filename, Rental *allRentals, int totalRentals);
int readRentals(char *filename, Rental *allRentals);
void writeCars(char *filename, Car *allCars, int totalCars);
int readCars(char *filename, Car *allCars);
/**
* main function, entry point of the program
*/
int main(int argc, char *argv[]) {
Car allCars[20]; // a list of cars
Rental allRentals[60]; // a list of rentals
int totalCars = 0; // number of all cars owned
int totalRentals = 0; // number of rental
int i = 0;
int menu_choice, renterIndex, carId;
char renterName[20], carMake[20];
totalCars = readCars(argv[1], allCars, totalCars);
totalRentals = readRentals(argv[2],allRentals, totalRentals);
while (1) {
printf("Please enter one of the menu choice: ");
printf("1. Add new car to the inventory. ");
printf("2. Make a reservation. ");
printf("3. Find a reservation using a renter name and print it to the screen. ");
printf("4. Print all rental information to the screen. ");
printf("5. Print car information to the screen of a selected car using the make of the car to search the inventory. ");
printf("6. Calculate and print average number of days rented. ");
printf("7. Exit program. ");
printf("Please input your choice:");
scanf("%d", &menu_choice);
switch (menu_choice) {
case 1:
totalCars = addNewCar(allCars, totalCars, 20);
break;
case 2:
totalRentals = addNewRental(allCars, totalCars, allRentals, totalRentals, 60);
break;
case 3:
printf("Please input the renter's name: ");
scanf("%s", renterName);
renterIndex = findReservation(allRentals, totalRentals, renterName);
printf("Renter name: %s ", renterName);
printf("Days renting: %d ", allRentals[renterIndex].daysRenting);
printCarInfo(allCars, totalCars, allRentals[renterIndex].carId);
break;
case 4:
printAllRentals(allRentals, totalRentals, allCars, totalCars);
break;
case 5:
printf("Please input the car make: ");
scanf("%s", carMake);
carId = findCarIDByMake(allCars, totalCars, carMake);
printCarInfo(allCars, totalCars, carId);
break;
case 6:
printf("The average number of days rented is: %.2lf ", getAverageRentalDays(allRentals, totalRentals));
break;
case 7:
printf("Thank you for using! Bye-bye! ");
totalCars = writeCars(argv[1], allCars, totalCars);
totalRentals = writeRentals(argv[2],allRentals, totalRentals);
return 0;
default:
printf("Invalid input! ");
}
printf(" ");
}
return 0;
}
void writeRentals(char *rental, Rental *allRentals, int totalRentals)
{
FILE *fp2 = fopen("rentals.txt", "w");
for(int i = 0; i < totalRentals; i++) {
fprintf(fp2, "%s %d %d ", allRentals[i].renterName, allRentals[i].daysRenting, allRentals[i].carId);
}
int readRentals(char *rental, Rental *allRentals){
int i = 0;
FILE *fp1 = fopen("rental.txt", "r");
if(fp1 ==NULL) {
printf("File doesn't exist");
}
while(!feof(fp1)){
fscanf(fp1, "%s %d %d", allRentals[i].renterName, &allRentals[i].daysRenting, &allRentals[i].carId);
i++;
}
return i;
}
void writeCars(char *car, Car *allCars, int totalCars){
FILE *fp2 = fopen("car.txt", "w");
if(fp2== NULL){
printf("File doesn't exist");
}
for(int i = 0; i < totalCars; i++)
fprintf(fp2, "%d %s %s %d %.2lf ", allCars[i].carId, allCars[i].make, allCars[i].model, allCars[i].numDoors, allCars[i].rate);
}
int readCars(char *car, Car *allCars){
int i = 0;
FILE *fp1 = fopen("car.txt", "r");
if(fp1== NULL) {
printf("File doesn't exist!");
}
else{
while(!feof(fp1)) {
fscanf(fp1, "%d %s %s %d %lf", &allCars[i].carId, allCars[i].make, allCars[i].model, &allCars[i].numDoors, &allCars[i].rate);
i++;
}
return i;
}
}
/**
* Creates the initial inventory
*/
int createInventory(Car *allCars) {
allCars[0].carId = 1234;
strcpy(allCars[0].make, "VW");
strcpy(allCars[0].model, "Golf");
allCars[0].numDoors = 2;
allCars[0].rate = 66.0;
allCars[1].carId = 2241;
strcpy(allCars[1].make, "Ford");
strcpy(allCars[1].model, "Focus");
allCars[1].numDoors = 4;
allCars[1].rate = 45.0;
allCars[2].carId = 3445;
strcpy(allCars[2].make, "BMW");
strcpy(allCars[2].model, "X3");
allCars[2].numDoors = 4;
allCars[2].rate = 128.0;
return 3;
}
/**
* Adds a new car to the list
*/
int addNewCar(Car *allCars, int totalCars, int size) {
if (totalCars == size) {
printf("Inventory full, add new car failed! ");
return totalCars;
}
printf("Please input the car ID: ");
scanf("%d", &allCars[totalCars].carId);
printf("Please input car make: ");
scanf("%s", allCars[totalCars].make);
printf("Please input car model: ");
scanf("%s", allCars[totalCars].model);
printf("Please input the number of doors: ");
scanf("%d", &allCars[totalCars].numDoors);
printf("Please input the rate: ");
scanf("%lf", &allCars[totalCars].rate);
totalCars++;
return totalCars;
}
/**
* Adds a new rental
*/
int addNewRental(Car *allCars, int totalCars, Rental *allRentals, int totalRentals, int size) {
char carMake[20];
int carId;
if (totalRentals == size) {
printf("Cannot add more rental information! ");
return totalRentals;
}
printf("Please input the renter's name: ");
scanf("%s", allRentals[totalRentals].renterName);
printf("Please input the days to rent: ");
scanf("%d", &allRentals[totalRentals].daysRenting);
do {
printf("Please input the car make: ");
scanf("%s", carMake);
} while ((carId = findCarIDByMake(allCars, totalCars, carMake)) == -1);
allRentals[totalRentals].carId = carId; // assume user input correct car make
totalRentals++;
return totalRentals;
}
/**
* Find a car ID by the maker of car
*/
int findCarIDByMake(Car *allCars, int totalCars, char *carMake) {
int i;
for (i = 0; i < totalCars; i++)
if (!strcmp(allCars[i].make, carMake)) return allCars[i].carId;
return -1;
}
/**
* Find a reservation by renter's name
*/
int findReservation(Rental *allRentals, int totalRentals, char *renterName) {
int i;
for (i = 0; i < totalRentals; i++)
if (!strcmp(allRentals[i].renterName, renterName)) return i;
return -1;
}
/**
* Find a car by car ID
*/
int findCarById(Car *allCars, int totalCars, int carId) {
int i;
for (i = 0; i < totalCars; i++)
if (allCars[i].carId == carId) return i;
return -1;
}
/**
* Print the details of all rentals
*/
void printAllRentals(Rental *allRentals, int totalRentals, Car *allCars, int totalCars) {
int i;
for (i = 0; i < totalRentals; i++) {
printf("Rental #%d ", i);
printf("Renter's name: %s ", allRentals[i].renterName);
printf("Days renting: %d ", allRentals[i].daysRenting);
printCarInfo(allCars, totalCars, allRentals[i].carId);
printf(" ");
}
}
/**
* Print the information of a car
*/
void printCarInfo(Car *allCars, int totalCars, int carId) {
int carIndex = findCarById(allCars, totalCars, carId);
printf("Car make: %s ", allCars[carIndex].make);
printf("Car model: %s ", allCars[carIndex].model);
printf("Number of doors: %d ", allCars[carIndex].numDoors);
printf("Renting rate: %.2lf ", allCars[carIndex].rate);
}
/**
* Calculate the average rental days
*/
double getAverageRentalDays(Rental *allRentals, int totalRentals) {
int i, sum = 0;
for (i = 0; i < totalRentals; i++)
sum += allRentals[i].daysRenting;
return (double) sum / totalRentals;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
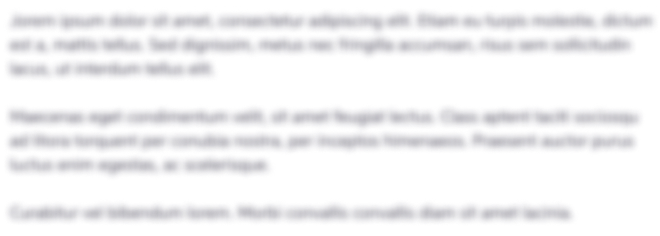
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started