Question
C++ Programming for a SKat Game Hello I need your help. My code doesnt sort the colors and I should rewrite the string face and
C++ Programming for a SKat Game Hello I need your help. My code doesnt sort the colors and I should rewrite the string face and string suit as an enum for card.h. How can I do it.
This are the question
It is played with 32 cards, of which each of the three players receives 10 cards. The remaining two cards remain face down on the table ('Skat'). So the global constants for the array sizes have the values DECK_SIZE = 32, PLAYER_SIZE = 10 and SKAT_SIZE = 2.
A Card constructor is supposed to have the two attributes suit and face with values adopted from outside initialize for card color and card value.
According to the rules of skat game, the enumeration types have:
-->for the card color (enum suit) the values {KARO = 0, HEART, PIK, KREUZ}
--> for the card value (enum face) the values {SEVEN = 0, EIGHT, NINE, TEN, BUBE, LADY, KOENIG, ASS}.
toString is supposed to separate the card color and the card value of the card by a space as a single return string (e.g. "diamond seven" or "heart lady").
The constructor of SkatGame should generate all 32 different cards of a Skat game (i.e. all 4 card colors (suit) with all 8 card values (face)) and save them in the attribute deck. Note: You can use loops with suitable int values for this and the int values for Transfer to the card constructor in suit or face cast.
shuffle is supposed to shuffle the cards, i.e. the 32 card objects in the array deck in a random order bring.
deal is supposed to completely transfer the 32 card objects in the array deck to the four arrays player1, player2, Split player3 and skat. In the first three arrays, each time after calling deal 10 of the card objects in deck and the other two card objects in skat. After all cards have been dealt, the arrays player1, player2 and player3 sorted in ascending order as follows: All CROSS cards are higher than all PIK cards, these are higher than all HERZ cards and higher than all KARO cards. Within the individual colors the order ASS, KOENIG, LADY, BUBE, TEN, NINE, EIGHT and SEVEN applies (ASS highest and SEVEN the lowest).
--> toString should return all card objects contained in the four arrays player1, player2, player3 and skat in a suitable formatting as a string. It must be possible to see how the 32nd Cards are divided between the three players and the skat (the other two cards).
-->getSkat should return the skat array, which contains two card objects, so that it can be called in the calling Program can be used.
--> Pay attention to 'const-correctness': All methods for which this is possible should be declared as const.
-->Creation of a SkatG
-->Mix and give the cards 10,000 times and determine how often under the two cards of the Skat at least one BUBE is included. Enter the value obtained both as an absolute numerical value (e.g.: "In 10,000 games viewed, there is at least one jack in the skat in 2379 games.") As well as relative -->Shuffle and give the cards 10,000 times and determine how often both Skat BUBE cards are. Enter the value obtained both as an absolute numerical value and as a relative frequency in percent out.
--> Mix and give the cards 10,000 times and determine how often under the two cards of the Skat at least one BUBE is included. Enter the value obtained both as an absolute numerical value (e.g.: "In 10,000 games viewed, there is at least one jack in the skat in 2379 games.") As well as relative Frequency in percent. (Example: "23.79 percent of the skat blades contain at least one jack in the skat.")
-->Shuffle and give the cards 10,000 times and determine how often both Skat BUBE cards are. Enter the value obtained both as an absolute numerical value and as a relative frequency in percent out.
This are the COdes
// card.h
#include
using std::string;
class Card { public: Card(); Card(string, string); ~Card(); string getSuit() const; string getFace() const; string toString() const;
private: string suit; string face; };
//skatGame.cpp
#include
SkatGame::SkatGame() { int k = 0; string Faces[8] = { "sieben","acht","neun","zehn","Bube","Dame","Koenig","As" }; //Definition Wert string Suits[4] = { "Kreuz","Pik","Herz","Karo" }; // Definition Farbe
for (int i = 0; i < 8; i++) { for (int j = 0; j < 4; j++) { deck[k] = Card(Faces[i], Suits[j]); cout << k + 1 << ": " << deck[k].toString() << endl; k++; } } }
SkatGame::~SkatGame() {
}
void SkatGame::shuffle() { Card mem; int pos;
for (int i = 0; i < 32; i++) { pos = 1 + rand() % 32; mem = deck[i]; deck[i] = deck[pos]; deck[pos] = mem; } }
void SkatGame::deal() { int pos = 0; for (int i = 0; i < 10; i++) { player1[i] = deck[pos]; pos++; }
for (int i = 0; i < 10; i++) { player2[i] = deck[pos]; pos++; }
for (int i = 0; i < 10; i++) { player3[i] = deck[pos]; pos++; }
for (int i = 0; i < 2; i++) { skat[i] = deck[pos]; pos++; }
}
void SkatGame::print() const { cout << "Karten fuer: " << endl << endl; cout << setw(15) << "Spieler 1 " << setw(15) << "Spieler 2 " << setw(15) << "Spieler 3 " << setw(15) << "Skat " << endl << setw(15) << "--------- " << setw(15) << "--------- " << setw(15) << "--------- " << setw(15) << "---- " << endl;
for (int i = 0; i < 10; i++) { cout << setw(15) << player1[i].toString() << setw(15) << player2[i].toString() << setw(15) << player3[i].toString(); if (i == 0 || i == 1) { cout << setw(15) << skat[i].toString(); } cout << endl; } cout << endl << endl; };
Card* SkatGame::getSkat() { return skat; }
//card.cpp
#include
Card::Card() {};
Card::Card(string a, string b) { face = a; suit = b; };
Card::~Card() {
}
string Card::getSuit() const //Ausgabe Farbe { return suit; }
string Card::getFace() const //Ausgabe Wert { return face; }
string Card::toString() const // Ausgabe Blatt des Spielers { string output; output = getFace() + " " + getSuit(); return output; }
//skatGame.h
#include "Card.h"
class SkatGame { private: Card deck[32]; Card player1[10]; Card player2[10]; Card player3[10]; Card skat[2]; public: SkatGame(); ~SkatGame(); void shuffle(); void deal(); void print() const; Card* getSkat(); };
//main.cpp
// Skat // Ziel: Sie sollen problemangepasste Datentypen als Komposition von Klassen realisieren und anwenden knnen.
#include
int main() { const int loops = 1000000; double sum;
int count = 0; SkatGame Skat; Card* card;
for (int i = 0; i < 5; i++) { Skat.shuffle(); Skat.deal(); Skat.getSkat(); Skat.print(); }
for (int i = 0; i < loops; i++) {
Skat.shuffle(); Skat.deal(); card = Skat.getSkat();
if (card[0].getFace() == "Bube" || card[1].getFace() == "Bube") {
count++; } }
cout << "Bei einer Million betrachteten Spielen ist bei " << count << " Spielen " << endl; cout << "mindestens ein Bube im Skat. " << endl;
sum = (static_cast
system("pause"); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
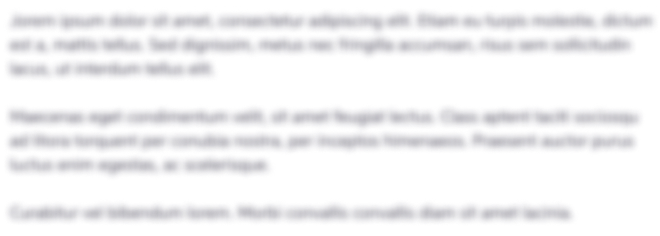
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started