Question
C++ PROGRAMMING Hi! I'm sorry to bombard you with so much code. It's just that they might be needed to understand the question: The domain
C++ PROGRAMMING
Hi! I'm sorry to bombard you with so much code. It's just that they might be needed to understand the question: The domain is a rectangular array of cells that can be occupied by shapes. The goal of the assignment is to implement a program that places randomly selected shapes at random positions on an arbitrary rectangular domain until the domain is full, and creates a graphical representation of the domain. I have written out most of my code for shape.cpp, I am having trouble with domain.cpp and the additional public members of shape. I have included the assignment, my shape code, domain.h, and the provided fill.cpp file. All help is appreciated!! Thank you
#include
#include
#include
#include "Shape.h"
Shape::~Shape(void)
{
delete x;
delete y;
}
int Shape::getX(int i) const
{
return i;
}
int Shape::getY(int i) const
{
return i;
}
void Shape::print(void) const
{
int i = 0;
cout
while (i
cout
i++;
}
cout
}
void Shape::draw(void) const
{
}
void Shape::move(int dx, int dy)
{
int i = 0;
while (i
x[i] = x[i] + dx;
y[i] = y[i] + dy;
i++;
}
}
bool Shape::overlap(const Shape & t) const
{
bool overlap = false; /o overlap by default
int i, j;
for (i = 0; i
for (j = 0; j
if ((x[i] == t.x[j]) && (y[i] == t.y[j])) {
overlap = true; // yes overlap
}
}
}
return overlap;
}
Shape * Shape::makeShape(char ch, int posx, int posy)
{
Shape* newShape = NULL;
if (ch == 'O') { // calls on appropriate shape depending on ch
newShape = new O(posx, posy);
}
else if (ch == 'I') {
newShape = new I(posx, posy);
}
else if (ch == 'L') {
newShape = new L(posx, posy);
}
else if (ch == 'S') {
newShape = new S(posx, posy);
}
else if (ch == 'X') {
newShape = new X(posx, posy);
}
else if (ch == 'U') {
newShape = new U(posx, posy);
}
else {
throw invalid_argument("invalid type"); // throws invalid argument if ch is not a correct kind of shape
}
return newShape;
}
O::O(int posx, int posy)
{
x = new int[1];
y = new int[1];
x[0] = posx; // creates positions for O
y[0] = posy;
}
char O::name(void) const
{
return 'O';
}
int O::size(void) const
{
return 1; // shape 'O' occupies 1 space
}
const char * O::color(void) const
{
return "cyan";
}
I::I(int posx, int posy)
{
x = new int[2];
y = new int[2];
x[0] = posx; // creates positions for I
y[0] = posy;
x[1] = posx;
y[1] = posy + 1;
}
char I::name(void) const
{
return 'I';
}
int I::size(void) const
{
return 2; // shape 'I' occupies 2 spaces
}
const char * I::color(void) const
{
return nullptr;
}
L::L(int posx, int posy)
{
x = new int[3];
y = new int[3];
x[0] = posx; // creates positions for L
y[0] = posy;
x[1] = posx + 1;
y[1] = posy;
x[2] = posx;
y[2] = posy + 1;
}
char L::name(void) const
{
return 'L';
}
int L::size(void) const
{
return 3; // shape 'L' occupies 3 spaces
}
const char * L::color(void) const
{
return nullptr;
}
S::S(int posx, int posy)
{
x = new int[4];
y = new int[4];
x[0] = posx; // creates positions for S
y[0] = posy;
x[1] = posx + 1;
y[1] = posy;
x[2] = posx + 1;
y[2] = posy + 1;
x[3] = posx + 2;
y[3] = posy + 1;
}
char S::name(void) const
{
return 'S';
}
int S::size(void) const
{
return 4; // shape 'S' occupies 4 spaces
}
const char * S::color(void) const
{
return nullptr;
}
X::X(int posx, int posy)
{
x = new int[5];
y = new int[5];
x[0] = posx; // creates positions for X
y[0] = posy;
x[1] = posx - 1;
y[1] = posy + 1;
x[2] = posx;
y[2] = posy + 1;
x[3] = posx + 1;
y[3] = posy + 1;
x[4] = posx;
y[4] = posy + 2;
}
char X::name(void) const
{
return 'X';
}
int X::size(void) const
{
return 5; // shape 'X' occupies 5 spaces
}
const char * X::color(void) const
{
return nullptr;
}
U::U(int posx, int posy)
{
x = new int[7];
y = new int[7];
x[0] = posx; // creates positions for U
y[0] = posy;
x[1] = posx + 1;
y[1] = posy;
x[2] = posx + 2;
y[2] = posy;
x[3] = posx;
y[3] = posy + 1;
x[4] = posx + 2;
y[4] = posy + 1;
x[5] = posx;
y[5] = posy + 2;
x[6] = posx + 2;
y[6] = posy + 2;
}
char U::name(void) const
{
return 'U';
}
int U::size(void) const
{
return 7; // shape 'U' occupies 7 spaces
}
const char * U::color(void) const
{
return nullptr;
}
//
// Domain.h
//
#ifndef DOMAIN_H
#define DOMAIN_H
#include "Shape.h"
#include
class Domain
{
public:
Domain(int sx, int sy);
void addShape(char type, int x, int y);
bool fits(const Shape &s) const;
void draw(void) const;
bool full(void) const;
private:
const int size_x, size_y;
std::vector sList;
};
#endif
//
// fill.cpp
//
#include "Shape.h"
#include "Domain.h"
#include
int main(int argc, char **argv)
{
// use: fill size_x size_y seed
// domain size
int size_x = atoi(argv[1]);
int size_y = atoi(argv[2]);
// seed for random number generator
int seed = atoi(argv[3]);
srand((unsigned) seed);
// create the domain
Domain domain(size_x,size_y);
char type[] = { 'O', 'I', 'L', 'S', 'X', 'U' };
while ( !domain.full() )
{
// try to insert a random shape
// draw a random number in [0,5]
// to select a random letter among 'O','I','L','S','X','U'
int itype = rand() % 6;
// generate a random position in [0,size_x), [0,size_y)
int x = rand() % size_x;
int y = rand() % size_y;
domain.addShape(type[itype],x,y);
}
// draw the domain
domain.draw();
}
//
// Shape.h
//
#ifndef SHAPE_H
#define SHAPE_H
class Shape
{
public:
virtual ~Shape(void);
virtual char name(void) const = 0;
virtual int size(void) const = 0;
virtual const char* color(void) const = 0;
int getX(int i) const;
int getY(int i) const;
void print(void) const;
void draw(void) const;
void move (int dx, int dy);
bool overlap(const Shape &t) const;
static Shape *makeShape(char ch,int posx,int posy);
protected:
int *x, *y;
};
class O: public Shape
{
public:
O(int posx, int posy);
virtual char name(void) const;
virtual int size(void) const;
virtual const char* color(void) const;
};
class I: public Shape
{
public:
I(int posx, int posy);
virtual char name(void) const;
virtual int size(void) const;
virtual const char* color(void) const;
};
class L: public Shape
{
public:
L(int posx, int posy);
virtual char name(void) const;
virtual int size(void) const;
virtual const char* color(void) const;
};
class S: public Shape
{
public:
S(int posx, int posy);
virtual char name(void) const;
virtual int size(void) const;
virtual const char* color(void) const;
};
class X: public Shape
{
public:
X(int posx, int posy);
virtual char name(void) const;
virtual int size(void) const;
virtual const char* color(void) const;
};
class U: public Shape
{
public:
U(int posx, int posy);
virtual char name(void) const;
virtual int size(void) const;
virtual const char* color(void) const;
};
#endif
input Input for the fill program is provided on the command line. It does not read input from any file. The command line argument of the fill program are: size x size y seed size x: size of the domain (number of cells) in the x direction size y: size of the domain (number of cells) in the y direction seed: an integer used as a seed for the random number generator rand used in fill.cpp The program is run, for example, using the following command line ./fill 12 10 1234 output. svg The fill program will be tested with various sizes in the range 1,151, and various seed values. It is not necessary to test for incorrect values in the command line arguments. You can use the fill executable with specific command line arguments, and compare your svg output with svg output test files provided on the web site to check the functionality of your classes. Note: the random numbers generated on different computers may differ even if the same seed is used. Make sure to generate and compare your output on the CSIF computers. Make sure that your program reproduces the test output exactly. Use the diff command to compare your files with the example files. Other command line arguments will also be used when grading your implementation. The example files are generated using the following naming convention. For example: /fill 12 10 2 test 12 10 2.svg input Input for the fill program is provided on the command line. It does not read input from any file. The command line argument of the fill program are: size x size y seed size x: size of the domain (number of cells) in the x direction size y: size of the domain (number of cells) in the y direction seed: an integer used as a seed for the random number generator rand used in fill.cpp The program is run, for example, using the following command line ./fill 12 10 1234 output. svg The fill program will be tested with various sizes in the range 1,151, and various seed values. It is not necessary to test for incorrect values in the command line arguments. You can use the fill executable with specific command line arguments, and compare your svg output with svg output test files provided on the web site to check the functionality of your classes. Note: the random numbers generated on different computers may differ even if the same seed is used. Make sure to generate and compare your output on the CSIF computers. Make sure that your program reproduces the test output exactly. Use the diff command to compare your files with the example files. Other command line arguments will also be used when grading your implementation. The example files are generated using the following naming convention. For example: /fill 12 10 2 test 12 10 2.svgStep by Step Solution
There are 3 Steps involved in it
Step: 1
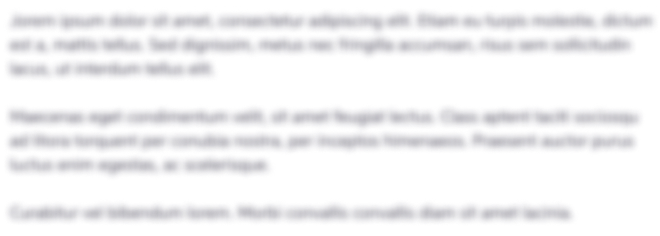
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started