Question
C# programming - I need to include a memory allocation method in an existing file system. It can be any method as long as it
C# programming - I need to include a memory allocation method in an existing file system. It can be any method as long as it supports the file system commands already coded. I have attached three classes the program is using. Thanks.
public class DUFS {
private static FileStream m_file = null; private static DUFS m_fs = null;
private DUFS(String VolumeName) { m_file = new FileStream(VolumeName, FileMode.Open); }
public static DUFS Mount(String VolumeName) { if (File.Exists(VolumeName)) m_fs = new DUFS(VolumeName);
return m_fs; }
public static Boolean Allocate(String VolumeName, int Size) { Boolean allocated = true;
FileStream file = new FileStream(VolumeName, FileMode.Create);
for (int i = 0; i < Size; i++) { file.WriteByte((byte)'\0'); }
file.Close();
return allocated; }
public static Boolean Deallocate(String VolumeName) { Boolean deallocated = true;
if (File.Exists(VolumeName)) File.Delete(VolumeName);
return deallocated; }
public static Boolean Truncate(String VolumeName) { Boolean truncated = true;
if (File.Exists(VolumeName)) { FileInfo fInfo = new FileInfo(VolumeName); FileStream file = new FileStream(VolumeName, FileMode.Open);
for (long i = 0; i < fInfo.Length; i++) { file.WriteByte((byte)'\0'); }
file.Close(); } else truncated = false;
return truncated; }
public static void Dump(String VolumeName) { if (File.Exists(VolumeName)) { FileStream file = new FileStream(VolumeName, FileMode.Open);
for (int i = 0; i < file.Length; i++) { Console.Write("{0}", file.ReadByte()); }
file.Close(); } }
public Boolean isMounted { get { return (m_fs != null); } }
public Boolean Create( String FileName ) { Boolean created = false; DateTime dtm_now = DateTime.Now; String dir_entry = String.Format("{0}", FileName);
return created; }
public void UnMount() { if (m_file != null) { m_file.Close(); m_file.Dispose(); m_file = null;
m_fs = null;
}
public class DirectoryEntry { private String m_file_name; private long m_file_size; private Boolean m_read_only; private long m_create_date; private long m_modified_date; private long m_start_cluster;
public DirectoryEntry() { m_file_name = String.Empty.PadRight(39); m_file_size = 0; m_read_only = false; m_create_date = DateTime.Now.ToBinary(); m_modified_date = DateTime.MinValue.ToBinary(); m_start_cluster = long.MinValue; }
public DirectoryEntry(Byte[] bytes) { String input = bytes.ToString();
String fileName = input.Substring(0, 39); Char read_only = input[40]; long file_size = long.Parse(input.Substring(41, 8));
}
public String FileName { get { return m_file_name; } set { m_file_name = value; } }
public long Size { get { return m_file_size; } set { m_file_size = value; } } public Boolean ReadOnly { get { return m_read_only; } set { m_read_only = value; } }
public String CreateDate { get { return new DateTime(m_create_date).ToString("MM/dd/yyyy"); } }
public String CreateTime { get { return new DateTime(m_create_date).ToString("HH:mm:ss"); } } public String ModifiedDate { get { return new DateTime(m_modified_date).ToString("MM/dd/yyyy"); } }
public String ModifiedTime { get { return new DateTime(m_modified_date).ToString("HH:mm:ss"); } }
public long StartCluster { get { return m_start_cluster; } set { m_start_cluster = value; } }
public override bool Equals(object obj) { if (this == obj) return true;
if (obj == null) return false;
if (GetType() != obj.GetType() ) return false;
DirectoryEntry entry = (DirectoryEntry)obj;
return this.FileName.Equals(entry.FileName, StringComparison.OrdinalIgnoreCase); }
public override int GetHashCode() { return m_file_name.GetHashCode(); }
public override string ToString() { return base.ToString(); }
} }
public class Program { public const int COMMAND_NAME = 0; // ALLOCATION public const int CMD_ALLOC_VOLNAME = 1; public const int CMD_ALLOC_SIZE = 2; // DEALLOCATION public const int CMD_DEALLOC_VOLNAME = 1; // TRUNCATE public const int CMD_TRUNC_VOLNAME = 1; // DUMP public const int CMD_DUMP_VOLNAME = 1; // MOUNT public const int CMD_MOUNT_VOLNAME = 1; // UNMOUNT // NO ARGUMENTS FOR UNMOUNT
public static Boolean isMounted = false; public static String volumeName = String.Empty; public static String Command = String.Empty; static void Main(string[] args) { DUFS dufs;
if (args.Length == 0) { Console.WriteLine("Invalid arguments"); return; }
Command = args[COMMAND_NAME];
// // ALLOCATE
String VolumeName = args[CMD_ALLOC_VOLNAME]; String Size = args[CMD_ALLOC_SIZE];
DUFS.Allocate(VolumeName, int.Parse(Size)); } else if (Command.Equals("DEALLOCATE")) { if (args.Length != 2) { Console.WriteLine("Invalid number of arguments for the DEALLOCATE command."); return; }
String VolumeName = args[CMD_DEALLOC_VOLNAME]; DUFS.Deallocate(VolumeName); } else if (Command.Equals("TRUNCATE")) { if (args.Length != 2) { Console.WriteLine("Invalid number of arguments for the TRUNCATE command."); return; }
String VolumeName = args[CMD_TRUNC_VOLNAME]; DUFS.Truncate(VolumeName); } else if (Command.Equals("DUMP")) { if (args.Length != 2) { Console.WriteLine("Invalid number of arguments for the DUMP command."); return; }
String VolumeName = args[CMD_DUMP_VOLNAME]; DUFS.Dump(VolumeName); } else if (Command.Equals("MOUNT")) { if (args.Length != 2) { Console.WriteLine("Invalid number of arguments for the MOUNT command."); return; }
if (isMounted) { Console.WriteLine("Volume '{0}' is already mounted. Unmount it before mounting another volume.", volumeName); return; }
String VolumeName = args[CMD_MOUNT_VOLNAME]; dufs = DUFS.Mount(VolumeName);
if (dufs != null) { String FileName = String.Empty; Boolean success = false; Console.WriteLine("Volume '{0}' is mounted.", VolumeName); while (dufs.isMounted) {
Console.Write("DUFS:>"); Command = Console.ReadLine();
if (Command.StartsWith("CREATE", StringComparison.OrdinalIgnoreCase)) { //CREATE FILENAME Command = Command.Trim(); FileName = Command.Split(' ')[1];
success = dufs.Create(FileName);
if (success) Console.WriteLine("File Created!"); else Console.WriteLine("The File Was Not Created! TO DO: Provide Error Messages!"); } else if (Command.StartsWith("EXIT", StringComparison.OrdinalIgnoreCase)) { dufs.UnMount(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
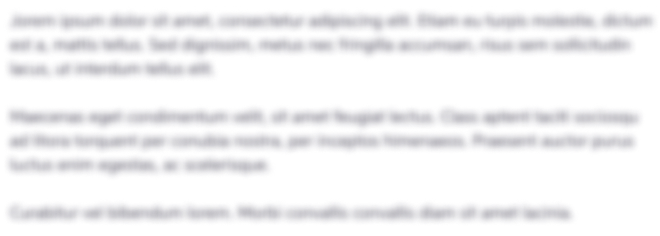
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started