Question
C++ PROGRAMMING. I've finished all the code but its not quite running correctly. Will attach all instructions and code. All code to be edited is
C++ PROGRAMMING. I've finished all the code but its not quite running correctly. Will attach all instructions and code. All code to be edited is in Text.cpp copied below. Please do not edit anything except Text.cpp.
Instructions:
- Implement the Text ADT (use the declaration in the Text.h file) (40 points)
- Text(), operator=() and ~Text(), getLength(), clear(), operator[]
- Programming Exercise 2: Implement the functions toUpper() and toLower() and test them by activating LAB1_TEST1 (30 points)
- Programming Exercise 3: Implement the ope
rators ==, <, > and test them by activating LAB1_TEST2 (30 points)
config.h:
/** * Text class (Lab 1) configuration file. * Activate test 'N' by defining the corresponding LAB1_TESTN to have the value 1. */
#define LAB1_TEST1 0 // Programming exercise 2: toUpper and toLower #define LAB1_TEST2 0 // Programming exercise 3: ==, <, and >
Text.h:
//-------------------------------------------------------------------- // // Laboratory 1 Text.h // **Instructor's Solution** // Class declaration for the array implementation of the Text ADT // //--------------------------------------------------------------------
#ifndef TEXT_H #define TEXT_H
#include
using namespace std;
class Text { public:
// Constructors and operator= Text ( const char *charSeq = "" ); // Initialize using char* Text ( const Text &other ); // Copy constructor void operator = ( const Text &other ); // Assignment
// Destructor ~Text ();
// Text operations int getLength () const; // # characters char operator [] ( int n ) const; // Subscript void clear (); // Clear string
// Output the string structure -- used in testing/debugging void showStructure () const;
//-------------------------------------------------------------------- // In-lab operations // toUpper/toLower operations (Programming Exercise 2) Text toUpper( ) const; // Create upper-case copy Text toLower( ) const; // Create lower-case copy
// Relational operations (Programming Exercise 3) bool operator == ( const Text& other ) const; bool operator < ( const Text& other ) const; bool operator > ( const Text& other ) const;
private:
// Data members int bufferSize; // Size of the string buffer char *buffer; // Text buffer containing a null-terminated sequence of characters
// Friends
// Text input/output operations (In-lab Exercise 1) friend istream & operator >> ( istream& input, Text& inputText ); friend ostream & operator << ( ostream& output, const Text& outputText );
};
#endif
test1.cpp:
//-------------------------------------------------------------------- // // Laboratory 1 test1.cpp // // Test program for the operations in the Text ADT // //--------------------------------------------------------------------
#include
//-------------------------------------------------------------------- // // Function prototype
void copyTester ( Text copyText ); // copyText is passed by value void print_help ( );
//--------------------------------------------------------------------
int main() { Text a("a"), // Predefined test text objects alp("alp"), alpha("alpha"), epsilon("epsilon"), empty, assignText, // Destination for assignment inputText; // Input text object int n; // Input subscript char ch, // Character specified by subscript selection; // Input test selection
// Get user test selection. print_help();
// Execute the selected test. cin >> selection;
cout << endl; switch ( selection ) { case '1' : // Test 1 : Tests the constructors. cout << "Structure of various text objects: " << endl; cout << "text object: alpha" << endl; alpha.showStructure(); cout << "text object: epsilon" << endl; epsilon.showStructure(); cout << "text object: a" << endl; a.showStructure(); cout << "empty text object" << endl; empty.showStructure(); break; case '2' : // Test 2 : Tests the length operation. cout << "Lengths of various text object:" << endl; cout << " alpha : " << alpha.getLength() << endl; cout << " epsilon : " << epsilon.getLength() << endl; cout << " a : " << a.getLength() << endl; cout << " empty : " << empty.getLength() << endl; break;
case '3' : // Test 3 : Tests the subscript operation. cout << "Enter a subscript : "; cin >> n; ch = alpha[n]; cout << " alpha[" << n << "] : "; if ( ch == '\0' ) cout << "\\0" << endl; else cout << ch << endl; break;
case '4' : // Test 4 : Tests the assignment and clear operations. cout << "Assignments:" << endl; cout << "assignText = alpha" << endl; assignText = alpha; assignText.showStructure(); cout << "assignText = a" << endl; assignText = a; assignText.showStructure(); cout << "assignText = empty" << endl; assignText = empty; assignText.showStructure(); cout << "assignText = epsilon" << endl; assignText = epsilon; assignText.showStructure(); cout << "assignText = assignText" << endl; assignText = assignText; assignText.showStructure(); cout << "assignText = alpha" << endl; assignText = alpha; assignText.showStructure(); cout << "Clear assignText" << endl; assignText.clear(); assignText.showStructure(); cout << "Confirm that alpha has not been cleared" << endl; alpha.showStructure(); break;
case '5' : // Test 5 : Tests the copy constructor and operator= operations. cout << "Calls by value:" << endl; cout << "alpha before call" << endl; alpha.showStructure(); copyTester(alpha); cout << "alpha after call" << endl; alpha.showStructure();
cout << "a before call" << endl; a.showStructure(); a = epsilon; cout << "a after call" << endl; a.showStructure(); cout << "epsilon after call" << endl; epsilon.showStructure(); break;
case '6' : // In-lab Exercise 2 // Test 6 : Tests toUpper and toLower cout << "Testing toUpper and toLower." << "Enter a mixed case string: " << endl; cin >> inputText; cout << "Input string:" << endl; inputText.showStructure(); cout << "Upper case copy: " << endl; inputText.toUpper().showStructure(); cout << "Lower case copy: " << endl; inputText.toLower().showStructure(); break;
case '7' : // In-lab Exercise 3 // Test 7 : Tests the relational operations. cout << " left right < == > " << endl; cout << "--------------------------------" << endl; cout << " alpha epsilon " << (alpha
default : cout << "'" << selection << "' specifies an inactive or invalid test" << endl; }
system("pause"); return 0; }
//--------------------------------------------------------------------
void copyTester ( Text copyText )
// Dummy routine that is passed a text object using call by value. Outputs // copyText and clears it.
{ cout << "Copy of text object" << endl; copyText.showStructure(); cout << "Clear copy" << endl; copyText.clear(); copyText.showStructure(); }
//--------------------------------------------------------------------
void print_help() { cout << endl << "Tests:" << endl; cout << " 1 Tests the constructors" << endl; cout << " 2 Tests the length operation" << endl; cout << " 3 Tests the subscript operation" << endl; cout << " 4 Tests the assignment and clear operations" << endl; cout << " 5 Tests the copy constructor and operator= operations" << endl;
cout << " 6 Tests the toUpper and toLower operations " #if LAB1_TEST1 << "(Active : " #else << "(Inactive : " #endif // LAB1_TEST1 << "In-lab Exercise 2)" << endl;
cout << " 7 Tests the relational operations " #if LAB1_TEST2 << " (Active : " #else << " (Inactive : " #endif // LAB1_TEST2 << "In-lab Exercise 3)" << endl; cout << "Select the test to run : "; }
Text.cpp:
#include
Text::Text ( const char *charSeq ) { bufferSize = strlen(charSeq) + 1; buffer = new char[bufferSize]; strcpy(buffer, charSeq); }
Text::Text ( const Text &other ) { bufferSize = other.bufferSize; buffer = new char[bufferSize]; strcpy(buffer, other.buffer); }
void Text::operator = ( const Text &other ) { int len = other.bufferSize; if (len > bufferSize) { delete[] buffer; bufferSize = other.bufferSize; buffer = new char[bufferSize]; } strcpy(buffer, other.buffer); }
Text::~Text () { delete[] buffer; }
int Text::getLength () const { return bufferSize - 1; }
char Text::operator [] ( int n ) const { // check if n is out of range first return 0; }
void Text::clear () { buffer[0] = '\0'; }
void Text::showStructure() const
// Outputs the characters in a string. This operation is intended for // testing/debugging purposes only.
{ int j; // Loop counter
for (j = 0; j < bufferSize; j++) cout << j << "\t"; cout << endl; for (j = 0; buffer[j] != '\0'; j++) cout << buffer[j] << "\t"; cout << "\\0" << endl; }
Text Text::toUpper( ) const { Text temp; for (int i = 0; i < bufferSize; i++) { if ((buffer[i] > 96 && (buffer[i] < 123))) { temp.buffer[i] = char(buffer[i] - 32); } } return temp; }
Text Text::toLower( ) const { Text temp; for (int i = 0; i < bufferSize; i++) { if ((buffer[i] > 64 && (buffer[i] < 91))) { temp.buffer[i] = char(buffer[i] + 32); } } return temp; }
bool Text::operator == ( const Text& other ) const { { if (bufferSize == other.getLength()) { if (bufferSize == 0) { return true; } else { for (int i = 0; i < bufferSize; i++) { if (buffer[i] != other.buffer[i]) { return false; } } return true; } } else { return false; } } }
bool Text::operator < ( const Text& other ) const { if (bufferSize < other.getLength()) { return true; } else { return false; } }
bool Text::operator > ( const Text& other ) const { if (bufferSize > other.getLength()) { return true; } else { return false; } }
textio.cpp:
//-------------------------------------------------------------------- // // Laboratory 1, In-lab Exercise 1 textio.cpp // // String input/output operations // //--------------------------------------------------------------------
#include
#include "Text.h"
//--------------------------------------------------------------------
istream & operator >> ( istream &input, Text &inputText )
// Text input function. Extracts a string from istream input and // returns it in inputText. Returns the state of the input stream.
{ const int textBufferSize = 256; // Large (but finite) char textBuffer [textBufferSize]; // text buffer
// Read a string into textBuffer, setw is used to prevent buffer // overflow.
input >> setw(textBufferSize) >> textBuffer;
// Apply the Text(char*) constructor to convert textBuffer to // a string. Assign the resulting string to inputText using the // assignment operator.
inputText = textBuffer;
// Return the state of the input stream.
return input; }
//--------------------------------------------------------------------
ostream & operator << ( ostream &output, const Text &outputText )
// Text output function. Inserts outputText in ostream output. // Returns the state of the output stream.
{ output << outputText.buffer; return output; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
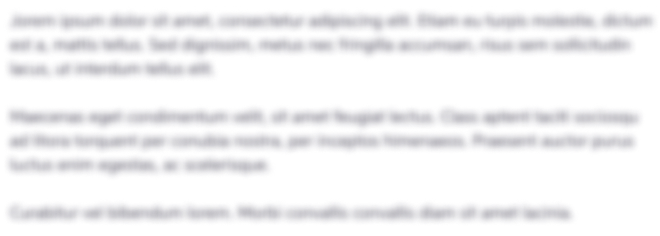
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started