Question
C Programming Lab Lab Procedures Setup The following steps will help you set up the lab. 1. Create a new folder in your OneDriveCISC130 folder,
C Programming Lab
Lab Procedures
Setup
The following steps will help you set up the lab.
1. Create a new folder in your OneDrive\CISC130 folder, and name it Lab09.
2. In Code::Blocks, make a new c file. Title it name1_name2_utilities.c and save it in the folder
you created above. Your names take the place of nameX above.
3. Make sure your complete first and last names are at the top of the file in comments.
4. Ensure that you have executing code that you have built and run prior to turning in your assignment. Test
your code and make sure it works for all equivalent inputs.
Activities
Today we will be solving some fun problems using recursion. You are NOT allowed to split up the tasks. All
group members must participate in solving each task. Your tasks are as follows:
0. Write a function that prints out your names.
1. Write a function that asks the user (I/O can be handled within the function) to enter a positive integer. If
the user enters a negative integer, warn them and then keep prompting them until they enter a positive
one. Your function should return the positive integer entered. You are not allowed to use a loop.
2. Write a recursive function for fast powering (it should work faster than the way we wrote it in class). It
should take an integer base and exponent; your function should return the base raised to the exponent. For
full credit, your function should leverage the fact that x^8 = x^4*x^4 and x^13=x^6*x^6*x. No loops are
allowed in this function.
3. Write a function that displays the Jarvis Sequence. You are not allowed to use loops. The Jarvis Sequence
is calculated as follows:
a. Take any positive integer n
b. Print n
c. If n is even, divide it by 2
d. If n is odd, multiply it by 3 and add 1
e. Repeat this process and print each integer in the sequence. Stop when n=1.
4. Write a function that takes an array and its size and fills the array with random numbers ranging from 7
to 320 (inclusive). You may not use loops.
5. Write a function that takes an integer array and its size and prints the array. Put commas between the
elements with the exception of the last element and, you guessed it, no loops.
6. Write a function that takes two integer arrays of the same size and copies the first one into the second one
after reversing the array. You will need additional function parameters to accomplish this. No loops.
7. Write a function that takes an integer x, an integer array, and the arrays size. It should return the number
of times x appears in the array. No loops.
8. Finally, create a driver.c file and a utilities.h file that uses all of these functions to generate the following
output (notice that every time a number needs to be entered the function from 1 is used.) Your output
should match exactly. Make your functions as atomic as possible. Driver.c should handle I/O.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
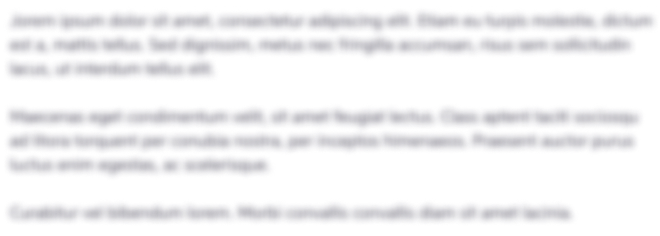
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started