Question
C++ Programming Language Assignment **** Inheritance *** (very important) Create a project that contains a base class and a class derived from the base class.
C++ Programming Language
Assignment
**** Inheritance *** (very important) Create a project that contains a base class and a class derived from the base class. | |
Purpose | Learn how to create a base class. Learn how to create a derived class. Create an object that accesses both the base and the derived class. Pass data to, and retrieve data from, both the base and derived objects. |
-----------------------------------------------------
Please help me by fixing my code.
-----------------------------------------------------
This is the original problem: "Create a program that sets the size and shape of a pie. The program should support pizza and desert pies. It should set the crust type, cheese type, and toppings 1, 2, and 3 for pizza pies, and the filling type for desert pies. The program should display the values set for each pie on the console".
-----------------------------------------------------
Below is the code I have so far. Please help me fix it.
-----------------------------------------------------
-----------------------------------------------------
// Module: pizzaClass.h
#pragma once class pizzaClass { protected: // protected so the derived class can use these data members string size; string shape; string crustType; string cheeseType; int toppings;
public: void setcrustType(string crust) { crustType = crust; } string getCrustType() { return crustType; } void setCheeseType(string cheese) { cheeseType = cheese; } string getCheeseType() { return cheeseType; } void setToppings(int number) { toppings = number; } int getToppings() { return toppings; } void setSize(string size) { this->size = size; } void setShape(string shape) { this->shape = shape; } string getsize() { return size; } string getShape() { return shape; }
pizzaClass(); ~pizzaClass(); };
-----------------------------------------------------
-----------------------------------------------------
// Module: pizzaClass.cpp
#include "stdafx.h" // include standard library #include
using namespace std; // using standard namespaces
pizzaClass::pizzaClass() { }
pizzaClass::~pizzaClass() { }
-----------------------------------------------------
-----------------------------------------------------
// Module: desertClass.h
#pragma once class desertClass :public pizzaClass //inherit pizzaClass { private: string fillingtype; //desert pie can access the data members and public functions of Pizza class
public: void setFillingType(string fill) { fillingtype = fill; } string getFillingType() { return fillingtype; }
desertClass(); ~desertClass(); };
-----------------------------------------------------
-----------------------------------------------------
// Module: desertClass.cpp
#include "stdafx.h" // include standard library #include
using namespace std;
desertClass::desertClass() { }
desertClass::~desertClass() { }
-----------------------------------------------------
-----------------------------------------------------
// Module: Main.cpp
#include "stdafx.h" // include standard library #include
using namespace std; // using standard namespaces
// main program entry void main() { desertClass desertPie; //creating object for DesertPie CLass
pizzaClass pizzaPie; // Creating Object for Pizza class
// Pizza class is base class and can access only its member function //through pizza object pizzaPie.setCheeseType("American Cheese"); pizzaPie.setToppings(5); pizzaPie.setShape("Round"); pizzaPie.setSize("Big"); pizzaPie.setcrustType("cheese-burst. Crust with oodles of yummy liquid cheese filled inside.");
cout << "The Pizza Cheese Type -->" << pizzaPie.getCheeseType() << endl; cout << "The pizza's Toppings are -->" << pizzaPie.getToppings() << endl; cout << "The Pizza's Shape is -->" << pizzaPie.getShape() << endl; cout << "The Pizza, Size is -->" << pizzaPie.getsize() << endl; cout << "The pizza crust Type is -->" << pizzaPie.getCrustType() << endl;
/* Since desertPie class inherit the pizza class as Public. So this class can use its member funcions and data members */ desertPie.setCheeseType("Cheddar"); desertPie.setFillingType("fried Potato"); desertPie.setShape("Round"); desertPie.setSize("Small"); desertPie.setToppings(5);
cout << "The desertPie Cheese Type -->" << desertPie.getCheeseType() << endl; cout << "The desertPie's Toppings are -->" << desertPie.getToppings() << endl; cout << "The desertPie's Shape is -->" << desertPie.getShape() << endl; cout << "The desertPie, Size is -->" << desertPie.getsize() << endl; cout << "The filling type is-->" << desertPie.getFillingType() << endl;
system("Pause"); //Pause the program so that the console does not disappear until a key is pressed. }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
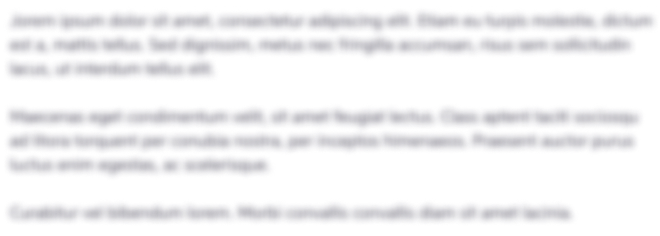
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started