Question
C programming: Please copy-and-paste the following files: bubbleSort.c #include header.h // PURPOSE: To sort the 'arrayLen' integers in array 'array' with the // bubble-sort algorithm.
C programming:
Please copy-and-paste the following files:
bubbleSort.c
#include "header.h" // PURPOSE: To sort the 'arrayLen' integers in array 'array' with the // bubble-sort algorithm. No return value. void bubbleSort (int* array, int arrayLen ) { int haveSwapped; int i; do { haveSwapped = 0; for (i = 0; i < arrayLen-1; i++) if (array[i] > array[i+1]) { swap(array,i,i+1); haveSwapped = 1; } } while (haveSwapped); }
insertionSort.c
#include "header.h" // PURPOSE: To sort the 'arrayLen' integers in array 'array' with the // insertion-sort algorithm. No return value. void insertionSort (int* array, int arrayLen ) { int i; int j; for (i = 0; i < arrayLen-1; i++) for (j = i+1; j < arrayLen; j++) if (array[i] > array[j]) swap(array,i,j);
}
These two files need a main() to run their functions bubbleSort() and insertionSort(). Then all three C files need a header file to inform them of what the others have that they need. Please finish both the main() and header.h.
Please note! Not everything needs to be shared.
main() needs bubbleSort() and insertionSort()
Both bubbleSort() and insertionSort() need swap().
Otherwise, it is best not to share too much, kind of like keeping methods and members private in C++ and Java.
header.h
#include#include #include #define MAX_LINE 256 #define RANGE_LOWEST 0 #define RANGE_HIGHEST 50000
main.c
#include "header.h" // PURPOSE: To hold the lowest allowed random number. int low = RANGE_LOWEST; // PURPOSE: To hold the highest allowed random number. int high = RANGE_HIGHEST; // PURPOSE: To return another randomly-generated number. int getNextNumber () { return( (rand() % (high - low + 1)) + low ); } // PURPOSE: To exchange the integers at indices 'i' and 'j' in 'array'. No // return value. void swap (int* array, int i, int j ) { // YOUR CODE HERE } // PURPOSE: To repeatedly ask the user the text "Please enter ", followed // by the text in 'descriptionCPtr', followed by the numbers 'low' and // 'high', and to get an entered integer from the user. If this entered // integer is either less than 'low', or is greater than 'high', then // the user is asked for another number. After the user finally enters // a legal number, this function returns that number. int obtainNumberBetween (const char* descriptionCPtr, int low, int high ) { // YOUR CODE HERE } // PURPOSE: To create and return an array of 'numNums' random integers. int* createArray (int numNums ) { int* array = (int*)malloc(numNums * sizeof(int)); int i; for (i = 0; i < numNums; i++) array[i] = getNextNumber(); return(array); } // PURPOSE: To print the 'arrayLen' integers in 'array'. No return value. void printArray (int* array, int arrayLen ) { // YOUR CODE HERE } // PURPOSE: To use the function obtainNumberBetween() to obtain the values // for variable 'numNums' (which must be between RANGE_LOWEST and // RANGE_HIGHEST). // Then it enters a loop asking the user what they want to do. If the // user chooses integer 1 then the program runs bubbleSort(array,numNums). // If the user chooses integer 2 then the program runs // quickSort(array,numNums). Returns 'EXIT_SUCCESS' to OS. int main () { int numNums = obtainNumberBetween ("number of numbers to sort", RANGE_LOWEST, RANGE_HIGHEST ); const char* msgCPtr = "What would you like to do? " "(1) Sort with bubble-sort " "(2) Sort with insertion-sort " "Your choice "; int choice = obtainNumberBetween(msgCPtr,1,2); int* array = createArray(numNums); switch (choice) { case 1 : bubbleSort(array,numNums); break; case 2 : insertionSort(array,numNums); break; } printArray(array,numNums); free(array); return(EXIT_SUCCESS); }
Sample Initial Output:
$ ./assign1 Please enter number of numbers to sort (0-50000): -5 Please enter number of numbers to sort (0-50000): 65536 Please enter number of numbers to sort (0-50000): 20 Please enter What would you like to do? (1) Sort with bubble-sort (2) Sort with insertion-sort Your choice (1-2): 1 2619 2623 3023 3237 3298 4719 6866 8639 9144 13018 13948 17629 20989 24577 27498 29668 29851 31859 33091 48010
Step by Step Solution
There are 3 Steps involved in it
Step: 1
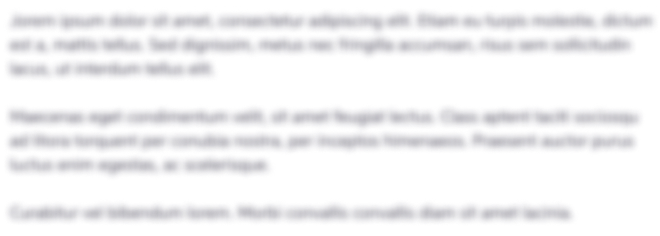
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started